Flutter is a versatile tool that enables developers to create applications with a wide range of functionalities. It is capable of developing applications that can run on multiple platforms seamlessly. As video calls become more and more popular since the covid-19, many developers are interested in making flutter call apps. No worry, keep going on! This tutorial shows us how to create a flutter video call application with ZEGOCLOUD SDK.
What is a Video Call?
A video call is a live communication service that enables two or more people to interact in real time through audio and video transmission. This technology combines the visual element with traditional voice communication, allowing participants to see each other using devices like smartphones, tablets, or computers equipped with cameras.
The use of video calls has become widespread, thanks to improved internet connectivity and the proliferation of camera-equipped devices, making it a popular choice for personal interactions, business meetings, and remote education. This technology bridges geographical gaps, enhancing communication with the added benefit of visual context and presence.
How Does Flutter Video Call Work?
Implementing a video call in a Flutter application involves using a combination of Flutter’s powerful UI capabilities and third-party libraries or APIs that support real-time video communication. Here’s a high-level overview of how video calls work in a Flutter environment:
1. Choosing a Video Call SDK/API
The first step is to select a reliable video call SDK or API that facilitates video calling. Services like Agora, ZEGOCLOUD, and Twilio provide Flutter-compatible SDKs that are specifically designed for real-time audio and video communication.
2. Adding the SDK to Your Project
Once you’ve chosen an SDK, you integrate it into your Flutter project. This typically involves adding the SDK as a dependency in your pubspec.yaml
file and importing the necessary libraries in your Dart code.
3. Initialization and Configuration
You need to initialize the SDK within your application, which might include setting up various configuration options such as tokens for authentication, server locations, and other settings that ensure the security and efficiency of your video call.
4. Accessing Device Hardware
Video calls require access to the device’s camera and microphone. Flutter apps handle these requirements by using specific plugins (like camera
for camera access and permission_handler
for requesting necessary permissions) to interact with native device features.
5. Creating a Call Interface
With Flutter’s widget-based architecture, you can design and customize the user interface for the video call. This includes buttons for starting and ending calls, toggling the camera, and muting the microphone, as well as the video display area where the video feeds will be shown.
6. Establishing the Connection
Using the SDK’s APIs, you establish a connection between the calling parties. This involves signaling to negotiate the media types and parameters and setting up a peer-to-peer connection or connecting via a server for the video and audio transmission.
7. Handling Real-Time Video and Audio
The SDK manages the complex aspects of the video call like capturing and encoding video and audio data, transmitting it over the internet, and then decoding it on the recipient’s device. This process also includes handling network fluctuations and adjusting the call quality accordingly to provide a smooth experience.
8. Ending the Call and Cleanup
When the call is concluded, the application must properly end the connection and release any resources used by the SDK, such as camera and microphone access, to avoid draining the device’s battery or using unnecessary data.
9. Testing and Optimization
Before final deployment, it’s crucial to test the video call functionality across multiple devices and network conditions to ensure its reliability and performance. Feedback from these tests can be used to tweak and optimize the call setup.
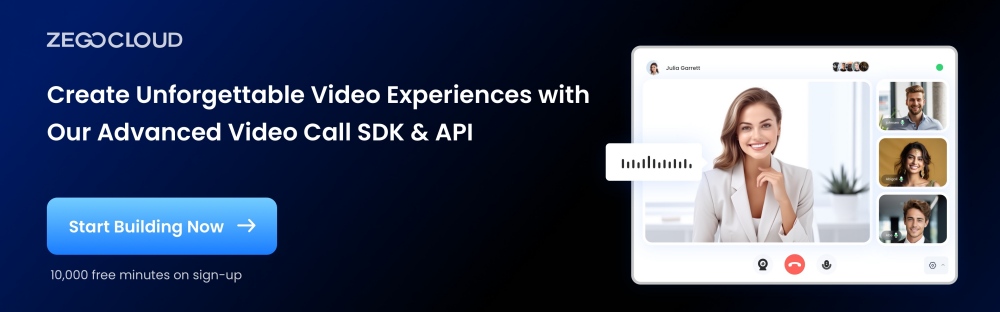
Why ZEGOCLOUD SDK for Building a Video Calling App
Choosing the right SDK is crucial for the success of a video calling app. In the context of Twilio and Agora Video SDK, ZEGOCLOUD SDK stands out for several compelling reasons, especially considering the discontinuation of Twilio’s Video SDK services.
- End of Twilio Video SDK Services: With Twilio discontinuing its Video SDK, developers need a reliable and long-term solution. ZEGOCLOUD SDK offers stability and ongoing innovation, making it an attractive Twilio migration choice for future-proofing your app.
- Feature Comparison with Agora: ZEGOCLOUD SDK boasts features essential for a modern video calling application as the best Agora SDK alternative: low-latency communication, high-definition video, and scalability for large-scale interactive live streaming, positioning it as a strong competitor to Agora Video SDK.
- Platform Support: ZEGOCLOUD SDK provides extensive support across iOS, Android, Web, Flutter, React Native, and Unity, enabling broader audience reach without compromising quality across different devices and operating systems.
- Scalability and Performance: Designed for growth, ZEGOCLOUD can handle a large number of users simultaneously, thanks to its global data centers. This scalability is crucial for apps targeting a wide user base.
- Pricing Model: With a flexible and competitive pricing model, ZEGOCLOUD SDK is accessible for startups and enterprises alike, allowing businesses to scale without facing prohibitive costs.
- Developer Support: ZEGOCLOUD emphasizes easy API integration and robust developer support, offering comprehensive documentation, an active community, and responsive support teams to resolve issues and implement features efficiently.
You may also like: ZEGOCLOUD vs Agora vs Twilio vs Vonage vs Zoom: In-Depth Comparison
ZEGOCLOUD UIKits – Build Faster & Ship More
ZEGOCLOUD UIKits is a collection of pre-designed user interface (UI) elements that can be used by developers to create visually appealing and intuitive applications with ease. It allows developers to build video communication applications with a seamless experience.
The UIKits come complete with a diverse range of design templates, including buttons, icons, frames, and other UI components, making it easier for developers to integrate video communication functionalities into their applications. These design templates can be used to create comprehensive UI designs for applications that are visually appealing and intuitive. It saves developers time and effort in designing UIs for their applications.
Through it, We can complete the development of video calls, live streaming, app chat and video conferences, and other scenarios within 10 minutes.
UIKits support many frameworks such as Flutter, react native, iOS, Android, and Web, which is very friendly for different developers to build their applications. If you are wondering how to build a video call app with Flutter, UIKits is the most recommended pre-built SDK to help you complete your app development easily and quickly.
As shown in the figure, Flutter UIKit SDK is responsible for processing audio and video calls and the logic related to text chat. Include:
- UI and interaction of the calling module
- Call status management
- Audio and video data transmission
You only need to implement business-related logic. For example:
- User login registration
- Friends List Management
- Call billing recharge
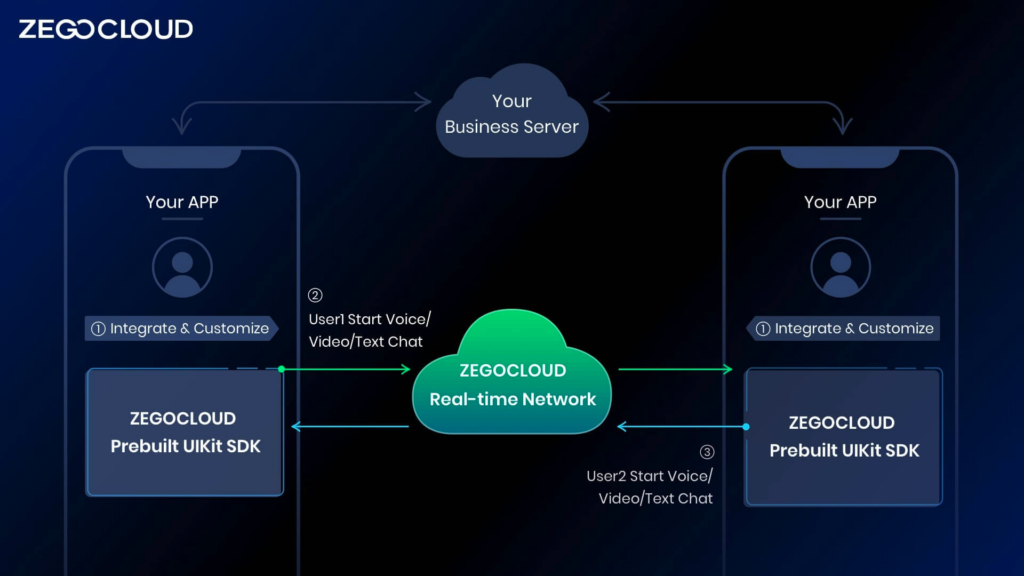
How to Build a Video Calling App in Flutter
Preparation
- A ZEGOCLOUD developer account–Sign up
- Flutter 1.12 or later.
- Basic understanding of Flutter development
Implement video call
Create Project
Run the following code to create a new project.
flutter create --template app.
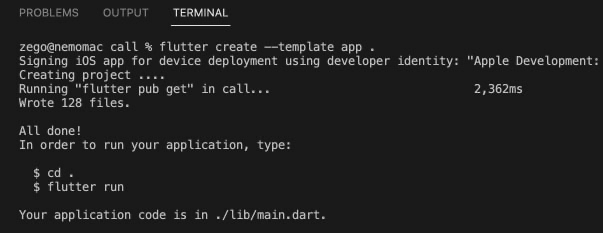
Add ZegoUIKitPrebuiltCall as a dependency
Run the following code in your project root directory:
flutter pub add zego_uikit_prebuilt_call
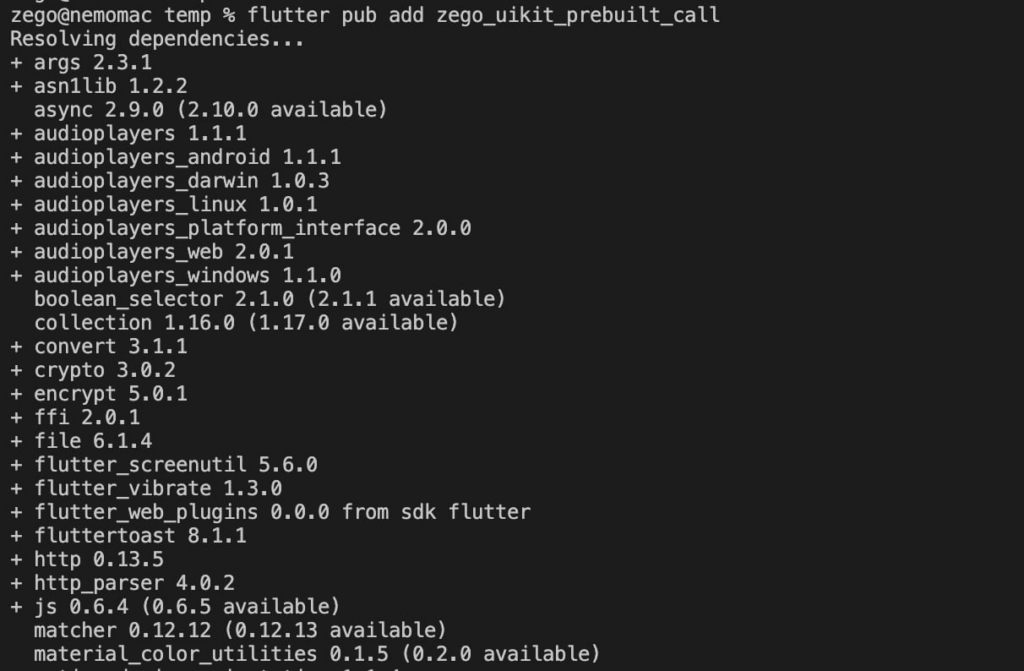
Add ZegoUIKitPrebuiltCall as dependency
Run the following code in your project root directory:
flutter pub add zego_uikit_prebuilt_call
Import the SDK
Now in your Dart code, import the prebuilt CallKit Flutter SDK.
import 'package:zego_uikit_prebuilt_call/zego_uikit_prebuilt_call.dart';
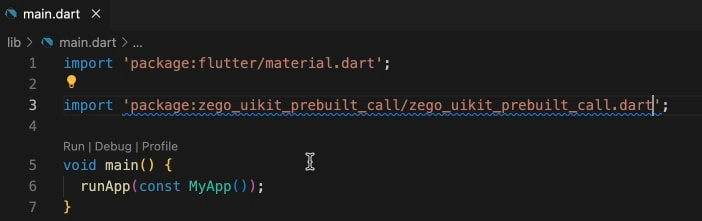
Start Video Call
Use ZegoUIKitPrebuiltCall
to build a Call page.
class CallPage extends StatelessWidget {
const CallPage({Key? key, required this.callID}) : super(key: key);
final String callID;
@override
Widget build(BuildContext context) {
return ZegoUIKitPrebuiltCall(
appID: yourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: yourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
callID: callID,
// You can also use groupVideo/groupVoice/oneOnOneVoice to make more types of calls.
config: ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall()
..onOnlySelfInRoom = () => Navigator.of(context).pop(),
);
}
}
Now, you can make a call by navigating to this. CallPage
.
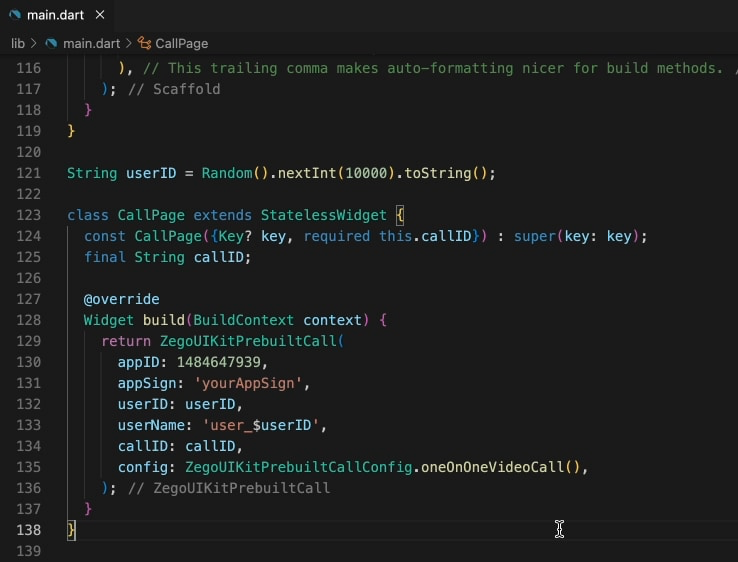
Add a call button
Add a Call button, and navigate to the CallPage.
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:zego_uikit_prebuilt_call/zego_uikit_prebuilt_call.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(home: HomePage());
}
}
class HomePage extends StatelessWidget {
final callIDTextCtrl = TextEditingController(text: "testCallID");
HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 10),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Expanded(
child: TextFormField(
controller: callIDTextCtrl,
decoration:
const InputDecoration(labelText: "start a call by id"),
),
),
ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) {
return CallPage(callID: callIDTextCtrl.text);
}),
);
},
child: const Text("call"),
)
],
),
),
),
);
}
}
Configure your project
- Android:
- If your project is created with Flutter 2. x.x, you will need to open the
your_project/android/app/build.gradle
file, and modify thecompileSdkVersion
to 33.
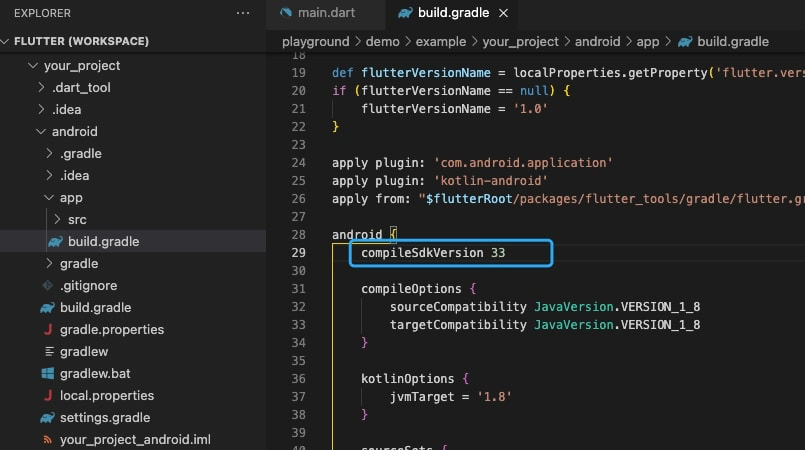
- Add app permissions.
Open the fileyour_project/app/src/main/AndroidManifest.xml
, and add the following code:
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
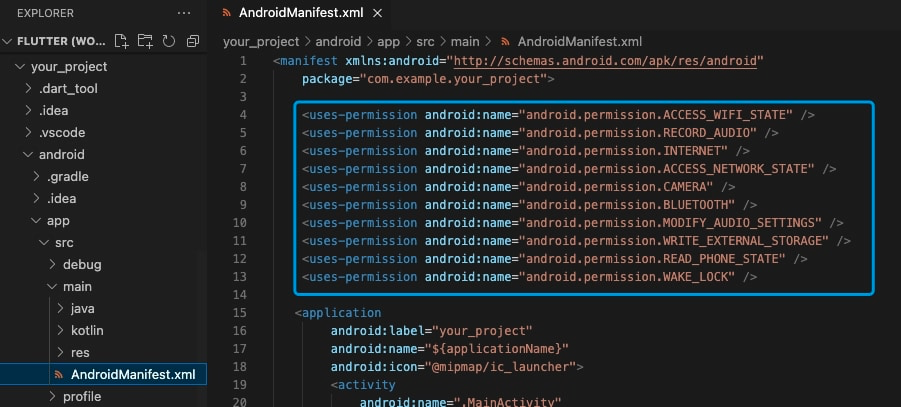
- Prevent code obfuscation.
To prevent obfuscation of the SDK public class names, do the following:
a. In your project’s your_project > android > app
folder, create a proguard-rules.pro
file with the following content as shown below:
-keep class **.zego.** { *; }
b. Add the following config code to the release
part of the your_project/android/app/build.gradle
file.
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
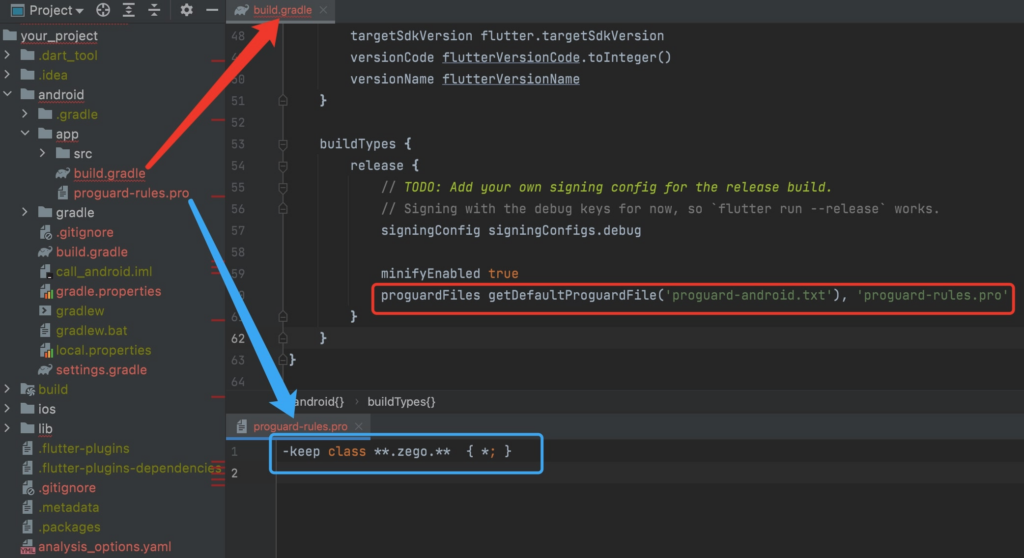
- iOS:
To add permissions, open your_project/ios/Runner/Info.plist
, and add the following code to the dict
part:
<key>NSCameraUsageDescription</key>
<string>We require camera access to connect to a call</string>
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to connect to a call</string>
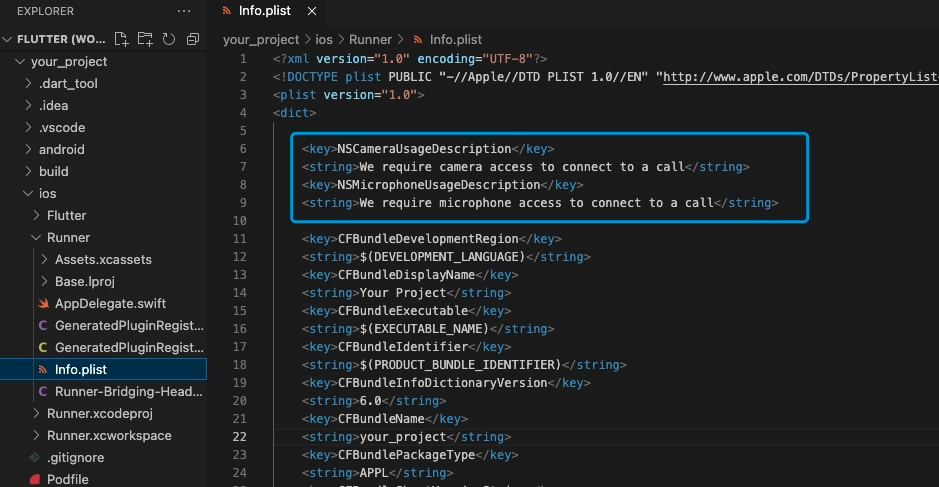
Run a Demo
Conclusion
It’s way easier to make a video call now than it was five or ten years ago. Additionally, their recent popularity also makes a point. In today’s market, a simple and secure video call app will definitely find its audience.
You can download the sample demo source code of this article to learn how to. If you have deeper requirements, such as streaming mix, noise reduction, censorship, etc. you can use our Core SDK. ZEGOCLOUD has the full customized video call SDK to meet what you want.
If you have any questions, you can always consult us at 24 hours for technical support.
Read more:
Flutter Video Call FAQ
Q1: How do I handle permissions for video calling in a Flutter app?
To handle permissions for video calling, especially for camera and microphone access, you can use the permission_handler
package. It’s essential to request and confirm these permissions at runtime, particularly on iOS and Android, to ensure the app functions properly and respects user privacy.
Q2: Can Flutter handle group video calls or only one-on-one video calls?
Flutter can handle both one-on-one and group video calls. The capability largely depends on the backend service you choose (like Agora, Twilio, or ConnectyCube) and how you configure the video streaming and connection management in your Flutter app.
Q3: Are there any specific UI/UX design considerations when building a video call app with Flutter?
Several UI/UX design considerations should be noted:
- Ensure buttons and controls are easily accessible during the call, considering both portrait and landscape modes.
- Provide visual feedback for user actions, like muting/unmuting the mic or switching cameras.
- Consider using overlays or minimizing the video feed window to allow multitasking within the app.
- Keep the interface clean and minimal to avoid distracting from the main functionality of the video call.
- Always test your UI on multiple devices to ensure a consistent and user-friendly experience across all screen sizes.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!