Flutter-WebRTC stands at the forefront of enabling real-time communication in mobile and web applications. This detailed guide dives into the essentials of integrating WebRTC with Flutter, providing developers with the knowledge to enhance app interactivity and connectivity. From setting up your environment to advanced features, we’ll cover everything you need to know to harness the power of Flutter-WebRTC in your projects.
What is Flutter?
Flutter is an open-source UI toolkit developed by Google. It allows developers to create high-performance, cross-platform mobile, web, and desktop apps using a single codebase. The toolkit uses the Dart programming language. It offers a rich set of customizable widgets to build visually appealing and responsive user interfaces. Flutter enables faster development cycles and smooth deployment across platforms. One standout feature is hot reload. This feature lets developers see code changes instantly, enhancing productivity and reducing development time.
What is WebRTC?
WebRTC (Web Real-Time Communications) is a real-time communication technology that allows network applications or sites to establish a peer-to-peer (Peer-to-Peer) connection between browsers without intermediaries to achieve video streaming and/or the transmission of audio streams or other arbitrary data.
WebRTC is not just for building desktop apps; it can be used to create iOS and Android apps too. The main difference between WebRTC for desktop and mobile apps is that you need to use a third-party library for the browser on mobile devices to access the camera and microphone.
What is Flutter WebRTC?
Flutter WebRTC is a plugin that enables real-time communication capabilities in Flutter applications. It leverages the Web Real-Time Communication (WebRTC) technology to allow audio and video communication to occur directly between browsers and devices without the need for an intermediary server, except for the initial signaling and connection establishment.
By integrating the Flutter WebRTC plugin, developers can build cross-platform Flutter applications that support live video calls and audio calls, peer-to-peer connections, and data channels. This is particularly useful for creating apps that require real-time interaction, such as video conferencing tools, live broadcasting apps, collaborative platforms, and more.
Advanced Features Of Flutter WebRTC
Focusing on a selection of advanced features from Flutter-WebRTC, let’s delve into the details of five key capabilities that significantly enhance the functionality and user experience of real-time communication applications built with Flutter.
1. Data Channel Support
Flutter-WebRTC’s data channel support is crucial for creating interactive and collaborative applications. This feature enables peer-to-peer text and binary data exchange, allowing developers to implement functionalities like chat systems, real-time game mechanics, or file transfers. It opens up a plethora of possibilities for real-time interaction beyond audio and video communication, making apps more engaging and versatile.
2. Screen Sharing
Screen sharing is an invaluable feature for educational, business, and collaborative applications, enabling users to share their entire screen or specific applications/windows with others. This functionality is essential for presentations, remote learning, and providing technical support, enhancing the utility of communication platforms by allowing for more detailed and interactive discussions.
3. Multi-party Conferencing
The ability of Flutter-WebRTC to support multi-party conferencing transforms business and educational interactions. ZEGOCLOUD simplifies this by providing a robust platform for seamless integration, ensuring high-quality, scalable conferencing solutions directly within Flutter apps.
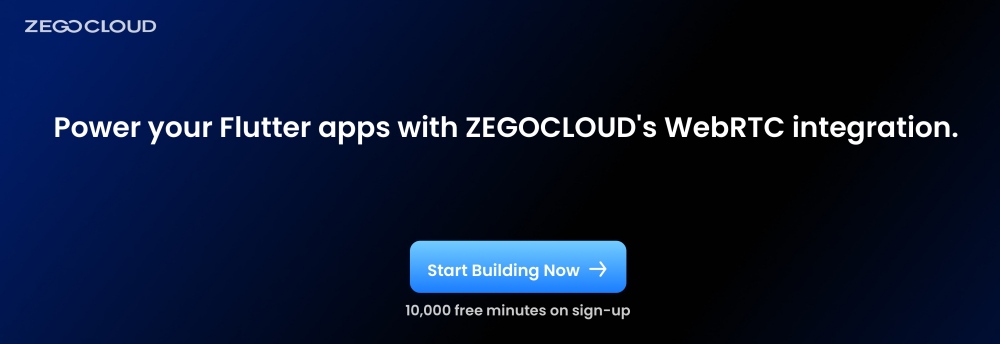
4. Network Adaptability
Network adaptability ensures that communication remains smooth and consistent even in varying network conditions. Flutter-WebRTC automatically adjusts the video quality based on the user’s bandwidth availability, minimizing interruptions and buffering issues. This adaptive streaming capability is crucial for maintaining a high-quality user experience, especially in regions with unstable internet connections.
5. Encryption and Security
Security is paramount in any application, and Flutter-WebRTC provides end-to-end encryption for all communications, ensuring that audio and video streams are protected from eavesdropping and interception. Utilizing standards like DTLS (Datagram Transport Layer Security) and SRTP (Secure Real-time Transport Protocol), Flutter-WebRTC guarantees that user data remains confidential and secure, fostering trust and compliance with privacy regulations.
How Does WebRTC Work?
WebRTC (Web Real-Time Communication) is a technology that enables real-time audio, video, and data sharing directly between browsers and devices without needing plugins or external software. Here’s how it works:
- Peer-to-Peer Connection: WebRTC establishes direct connections between users (peers) without going through a central server, reducing latency and improving performance.
- Signaling: Before the connection is made, signaling protocols (using WebSockets, SIP, etc.) exchange network information, session control messages, and media capabilities to ensure both users are ready to communicate.
- ICE Framework: The Interactive Connectivity Establishment (ICE) framework helps WebRTC find the best path for data to travel between peers, navigating firewalls and NATs (Network Address Translators) to establish the connection.
- Media Exchange: WebRTC uses codecs like VP8, VP9, and H.264 for video, and Opus for audio, ensuring high-quality, real-time communication. SRTP (Secure Real-time Transport Protocol) is used to encrypt the media streams.
- Data Channels: WebRTC also supports data channels, enabling fast peer-to-peer transfer of arbitrary data, which can be used for file sharing, gaming, or text-based communication.
WebRTC is widely used in applications like video conferencing, file sharing, and live streaming, allowing smooth, secure, and low-latency communication.
Seamlessly Integrate WebRTC into Your Flutter App with ZEGOCLOUD
Creating a flutter WebRTC video call application from scratch can be challenging, time-consuming, and require much technical knowledge. Fortunately, ZEGOCLOUD provides prebuilt UI components that allow developers to build robust and scalable applications quickly and easily.
ZEGOCLOUD UIKits is designed to help developers build video conferencing applications using Flutter WebRTC. This UIKit is a set of customizable components that enable developers to create high-quality video conferencing apps without worrying about technical details. It has many features that make it a reliable and efficient toolkit for building Flutter Webrtc video call applications.
ZEGOCLOUD features include:
- One-to-One Video Calls: Enables private video chats with high-quality, low-latency video and audio. Features like noise suppression and echo cancellation ensure clear audio, even in noisy environments.
- Group Video Calls: Supports video calls with up to 100 participants, perfect for large meetings or webinars. Includes speaker identification and active speaker tracking to enhance focus during conversations.
- Customizable UI Components: Offers developers the flexibility to customize the video call interface, including buttons and chat, to create a tailored user experience.
- Scalable Infrastructure: Built for scalability, it allows for global access with minimal latency and high reliability. Advanced security, including end-to-end encryption, protects user data.
Steps to Build WebRTC Apps with Flutter
Follow the steps below to create your WebRTC video conference app using ZEGOCLOUD’seo Conference Kit:
1. Add ZegoUIKitPrebuiltVideoConference
as dependencies
Execute this code in your project’s directory to add the dependency.
flutter pub add zego_uikit_prebuilt_video_conference
2. Import the SDK
Import the prebuilt Video Conference Kit SDK into your Dart code.
import 'package:zego_uikit_prebuilt_video_conference/zego_uikit_prebuilt_video_conference';
3. Utilize ZegoUIKitPrebuiltVideoConference in your project.
Retrieve your project appSign from the ZEGOCLOUD Admin Console. Specify userID
and userName
for Video Conference Kit connection. Generate or create a conferenceID
for the desired video conference.
class VideoConferencePage extends StatelessWidget {
final String conferenceID;
const VideoConferencePage({
Key? key,
required this.conferenceID,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltVideoConference(
appID: YourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: YourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
conferenceID: conferenceID,
config: ZegoUIKitPrebuiltVideoConferenceConfig(),
),
);
}
}
Initiate a video conference by accessing. VideoConferencePage
.
4. Configure your project
For seamless integration with your app, configuration of your setup is required. Follow the steps below to do so:
Android
- For Flutter 2. x.x projects, adjust the
compileSdkVersion
to 33 inyour_project/android/app/build.gradle
file. - Include app permissions by opening
your_project/app/src/main/AndroidManifest.xml
file and adding the following code:
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
- Prevent code obfuscation.
To avoid obfuscation of SDK public class names, perform the following steps:
a. Create a proguard-rules.pro file <strong>in</strong> your_project > android > app
folder with the following content:
-keep class .zego. { *; }
b. Add the following configuration code to the release section of your_project/android/app/build.gradle
file:
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
iOS
To grant permissions, access your_project/ios/Runner/Info.plist
and add the following code to the dict
section:
<key>NSCameraUsageDescription</key>
<string>Access permission to camera is required.</string>
<key>NSMicrophoneUsageDescription</key>
<string>Access permission to microphone is required.</string>
Integrate WebRTC with Flutter via ZEGOCLOUD
Integrating WebRTC with Flutter through ZEGOCLOUD offers a streamlined approach to incorporating real-time communication features into your mobile apps. By leveraging ZEGOCLOUD’s robust SDK, developers can easily harness WebRTC’s capabilities to enable high-quality audio, video, and data sharing without the complexities of manual integration. ZEGOCLOUD simplifies the process with its pre-built UIKits, ensuring that your app can deliver low-latency, secure communication experiences across platforms.
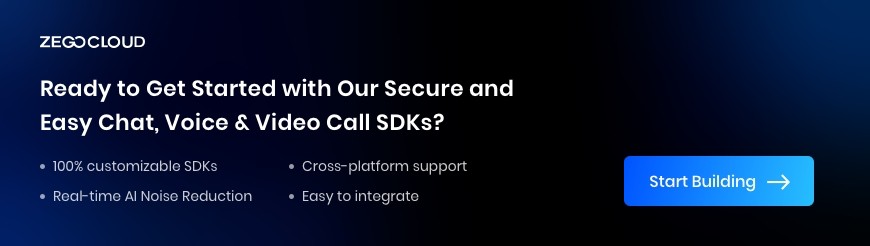
Additionally, ZEGOCLOUD provides excellent scalability, reliable performance, and built-in tools for managing user connections, making it an ideal solution for developers looking to integrate real-time communication efficiently. Whether for video conferencing, online collaboration, or live streaming, ZEGOCLOUD’s API ensures that WebRTC works seamlessly with Flutter, helping you create a feature-rich, user-friendly app.
Conclusion
Building a Flutter WebRTC app can be made easier using ZEGOCLOUD’s SDK, particularly the pre-built UIKits. By following this step-by-step Flutter WebRTC tutorial and utilizing this kit, developers can easily create robust and scalable video conferencing applications. Sign up now for ZEGOCLOUD and get 10,000 free minutes to start.
Read more:
Flutter WebRTC FAQ
Q1: How can I achieve low latency in Flutter WebRTC applications?
Achieving low latency in Flutter WebRTC applications involves optimizing network conditions, using efficient codecs, and selecting the closest server for signaling. Additionally, ensuring that your application’s infrastructure is robust and scalable can help reduce latency.
Q2: Can Flutter WebRTC be integrated with other real-time communication platforms like Firebase or Twilio?
Flutter WebRTC can be integrated with other real-time communication platforms, such as Firebase or Twilio. These integrations can enhance your app’s capabilities with additional features like messaging, storage, and more, although direct communication setup for WebRTC will still be necessary.
Q3: Are there any limitations to using Flutter WebRTC on different platforms?
While Flutter WebRTC aims to provide a consistent experience across platforms, there may be some platform-specific limitations or requirements, such as permissions handling, codec support variations, and performance differences due to hardware capabilities. Testing across devices and platforms is crucial to ensure a smooth user experience.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!