Want to learn how to build web applications? You’ve found the right guide! We’ll explain what web application development is, the different parts of it, what skills you’ll need, and how to get started. Even if you’re a development beginner, this guide will give you the basics to start making your own web apps. We’ll also share web application development tips and resources to help you learn.
What is a Web Application?
A web application is a software program that runs on a web server and is accessed through a web browser over a network such as the Internet or an intranet. Unlike traditional desktop applications, which are launched by your operating system, web apps must be accessed through a web browser. This approach means that web applications do not need to be downloaded or installed on the user’s device; instead, they are accessed and used directly in the browser, which makes them platform-independent and easily accessible from anywhere.
Web applications can range from simple tools like email services and online calculators to complex software systems such as project management tools, social media platforms, and e-commerce sites. They are developed using standard web technologies, including HTML, CSS, and JavaScript, and often involve both frontend and backend components. These components work together to manage the user interface, application logic, and data storage and retrieval. The convenience, ease of updates, and ability to support multiple users simultaneously are key reasons for the growing popularity of web applications.
What is Web Application Development?
Web application development is the process of making apps that you use in a web browser over the internet. Web apps are not installed on your computer like regular programs. Instead, they are hosted on remote servers and you access them through a website.
The main parts of a web app are:
- The front-end user interface is made with HTML, CSS, and JavaScript that runs in the browser. This is the part you see and interact with.
- The back-end code is made with languages like PHP, Ruby, Python, Java, etc. that run on the server. This handles the behind-the-scenes logic.
- The database that stores and manages data for the app.
To build web apps, web application developers need skills in:
- Front-end coding for the user interface
- Back-end coding for server-side logic
- Databases for storing and managing data
Frameworks like React, Angular, Django, Rails, Spring, and Laravel help speed up web app development. The goal is to build an interactive app that is useful to users over the web with a great experience.
Web apps are very common today – webmail, social networks, streaming, shopping sites, and more are web apps we use daily. With complex features and the need to handle many users, web app development today uses agile methodologies.
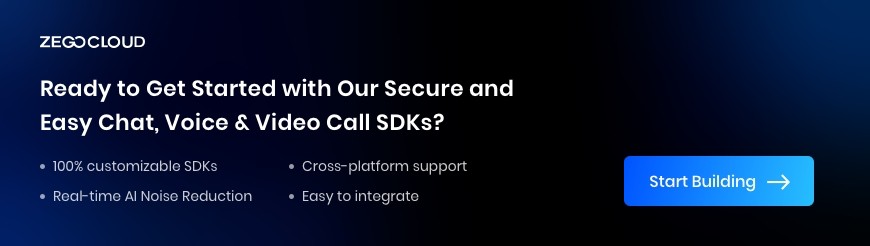
What is the Difference Between Web Applications and Websites?
Websites and web applications are different. Websites are static, meaning they do not change unless you refresh the page. They typically provide information, such as text, images, and videos. You can click links or fill out forms, but that’s all you can do.
Web applications, on the other hand, are dynamic. They change as you use them, allowing you to do more, such as login, change data, leave comments, and buy things. Webmail, document editors, social media, and shopping sites are all examples of web applications.
Websites and web applications can both look nice, but web applications require more programming behind the scenes to work well. Website design focuses on how the website looks, while web application design focuses on making the features work using databases and servers.
In short, websites provide static information, while web applications make that information interactive. If you just need information, use a website. If you need to interact with data, use a web application. They both have important roles in our online experiences.
Skills Required for Web App Development
Developing web applications requires a mix of technical and soft skills to build functional and user-friendly platforms. Here’s a streamlined overview:
Technical Skills:
- Frontend Development: Key skills include HTML/CSS for structure and style, JavaScript for interactivity, and frameworks like React or Angular for efficient UI development.
- Backend Development: Involves programming languages like Python (Django), JavaScript (Node.js), or Java, and understanding database management with SQL or NoSQL databases. Knowledge of creating and managing APIs using REST or GraphQL is also essential.
- Full-Stack Development: Combines both frontend and backend skills, requiring knowledge of web frameworks such as Django for Python or Express for Node.js, and version control tools like Git.
- Testing and Debugging: Skills in writing unit tests, performing integration testing, and debugging help ensure the reliability and functionality of the application.
- Security and Performance: Includes implementing security best practices, understanding authentication and authorization, and using strategies like caching and load balancing to optimize performance.
Soft Skills:
- Problem-Solving: Essential for diagnosing issues and devising effective solutions.
- Communication: Important for explaining complex ideas and collaborating with team members.
- Adaptability: Crucial for keeping up with evolving technologies and methodologies.
These competencies help developers create robust, scalable web applications that cater to diverse user needs and drive business success.
Web Application Development Frameworks
Web application development frameworks are like toolkits for creating dynamic websites and apps. Here are some top picks:
Frontend
- React – This is a JavaScript library perfect for crafting user interfaces. It’s fast and comes with lots of tools.
- Vue.js – Known for being light and easy to get into. It’s awesome for creating interactive screens.
- Angular – Made by Google and uses TypeScript. It’s great for single-page apps and allows two-way data sharing.
- Ember – This one focuses on setting standards rather than making you decide everything. It uses modern JavaScript and handles common tasks well.
Backend
- Express – A no-fuss framework using Node.js. It’s ideal for making REST APIs and web apps.
- Django – A Python framework that comes with lots of features, like a database manager, right out of the box.
- Ruby on Rails – Uses Ruby and focuses on getting things done quickly and elegantly. It sets strong guidelines to follow.
- Laravel – Built on PHP and offers a clean, elegant way to code. It’s packed with robust features.
Full-stack frameworks such as ASP.NET and Spring Boot make it easy to create everything you need for a web app. This includes the user interface on the front end, the backend functions, and even the database.
They use a design approach called the Model-View-Controller pattern. This helps keep different parts of the app separate but well-organized, making the whole process of building a complete web app more straightforward.
5 Examples of Web Applications
Here are some real-world examples of web applications that you probably use every day. These apps make our lives easier, more fun, and more connected.
- Google Docs: We have Google Docs. It’s an online word processor that lets you create, edit, and share documents. You can collaborate with others in real time, making team projects a breeze.
- Facebook: Who hasn’t heard of Facebook? It’s a social media platform where you can connect with friends, share photos, and even join groups that interest you.
- Netflix: The go-to web app for streaming movies and TV shows. Just log in, pick what you want to watch, and you’re good to go.
- Dropbox: It’s a cloud storage app that allows you to save files online and access them from any device. Hence, super handy for keeping your files organized and safe.
- Amazon: It’s an online marketplace where you can buy pretty much anything, from books to electronics to groceries.
So there you have it, five web applications that serve different needs but all make our lives easier in some way. Whether it’s for work, socializing, entertainment, storage, or shopping, web apps have got you covered!
How Can ZEGOCLOUD Help for Web Application Development
ZEGOCLOUD lets you easily add real-time communication to your web apps. To demonstrate, we will build a simple chat app using JavaScript and the ZEGOCLOUD chat SDK. First, we will set up our project. Next, we will initialize the ZEGOCLOUD SDK. Then, we will create the UI for the chat window. Finally, we will add the code to send and receive messages. Now, let’s get started building our chat app.
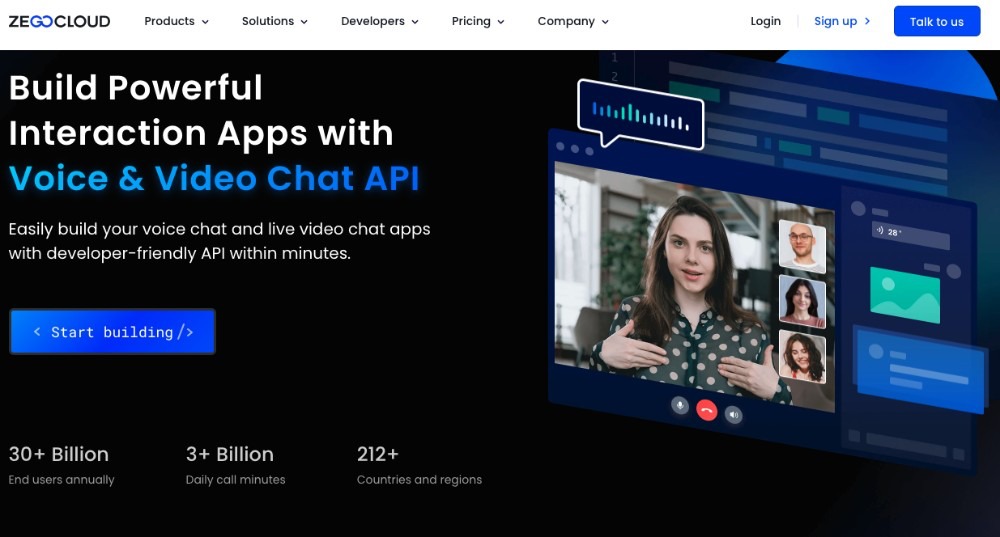
Preparation
- A ZEGOCLOUD developer account – Sign up
- Create a project in the ZEGOCLOUD Admin Console to get app credentials
- Basic knowledge of the web
- Recent web browsers
Create you app
- To make a folder for your audio and video projects, first create a new folder with subfolders inside it like this:
├── assets
│ ├── css
│ │ └── index.css # styling.
│ └── js
│ ├── biz.js # Personal JavaScript logic
│ └── zim.js # zim sdk
├── index.html # UI file
- Place the following code below into your
index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no" />
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" />
<meta name="renderer" content="webkit" />
<title>ZIM</title>
<link rel="stylesheet" href="assets/css/index.css" />
</head>
<body></body>
<script src="./assets/js/zim.js"></script>
<script src="./assets/js/biz.js"></script>
</html>
Integrate the SDK
To build a real-time chat app using JavaScript, the first thing you need to do is add ZEGOCLOUD’s In-chat SDK to your project. Here’s how to do it in a few simple steps:
- To get the required components, run the given command to pull all the dependencies:
npm i zego-zim-web
- In your project’s zim.js file, add the following import line:
import { ZIM } from 'zego-zim-web';
Create a ZIM SDK instance
To start a ZIM instance, create a unique instance for each client when they log in. For example, for clients A and B to chat, both should use the create
method with the AppID from the Prerequisites section. This sets up individual ZIM SDK instances for smooth messaging between them.
var appID = xxxx; // Note: appID is a number, not a string.
// The 'create' method initializes a ZIM instance once. Further calls to 'create' will return null.
ZIM.create({ appID });
// Use 'getInstance' to prevent issues with hot updates or multiple 'create' invocations.
var zim = ZIM.getInstance();
Set event callbacks
Before a user signs in, it’s important to use the on
method to set up custom event responses. This lets you get alerts for any SDK mistakes or message events, making it easier to handle various situations.
// Set up a callback for handling error codes.
zim.on('error', function (zim, errorInfo) {
console.log('SDK Error:', errorInfo.code, errorInfo.message);
});
// Initialize a callback to monitor changes in the connection status.
zim.on('connectionStateChanged', function (zim, { state, event, extendedData }) {
console.log('Connection Status Changed:', state, event, extendedData);
// Re-login if SDK logs out due to prolonged network disconnection.
if (state === 0 && event === 3) {
zim.login(userInfo, token);
}
});
// Establish a callback for incoming direct messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('Direct Message Received:', messageList, fromConversationID);
});
// Set up a callback to get alerts when the token is about to expire.
zim.on('tokenWillExpire', function (zim, { second }) {
console.log('Token Expiry Alert:', second);
// Use renewToken method to refresh the expiring token.
zim.renewToken(token)
.then(function({ token }) {
// Token successfully renewed.
})
.catch(function(err) {
// Token renewal failed.
});
});
Log in to the ZIM SDK
After creating the ZIM SDK instances for client A and client B, you need to have both clients log in to the ZIM SDK. This will allow them to exchange messages and renew their tokens when needed.
Follow these steps:
- For each client, use the
ZIMUserInfo
method to create a user object. This user object will contain information specific to that client. - Call the
login
method, providing the user object you created in step 1 along with the Token you obtained earlier from the Prerequisites section.
var userInfo = { userID: 'xxxx', userName: 'xxxx' };
var token = '';
zim.login(userInfo, token)
.then(function () {
// Login successful.
})
.catch(function (err) {
// Login failed.
});
Send messages
After logging in, client A can start chatting with client B. The ZIM SDK supports various message types. Client A can use the sendMessage
method to send direct messages to client B. A confirmation is sent back to client A to confirm the message was delivered.
Additionally, the onMessageAttached
feature allows client A to work with unsent messages. This is useful for getting the message ID before sending or for adding special effects when sending large files like videos.
// Send direct text messages.
var toConversationID = ''; // ID of the user you're messaging.
var conversationType = 0; // Type of chat: 1-on-1 chat (0), in-room chat (1), group chat (2).
var config = {
priority: 1, // Message priority levels: Low (1, default), Medium (2), or High (3).
};
var messageTextObj = {
type: 1,
message: 'Message text',
extendedData: 'Optional extra info for the message'
};
var notification = {
onMessageAttached: function(message) {
// Placeholder for loading actions.
}
};
// Send the message.
zim.sendMessage(messageTextObj, toConversationID, conversationType, config, notification)
.then(function ({ message }) {
// Message sent successfully.
})
.catch(function (err) {
// Message sending failed.
});
Receive messages
Once client B logs in, they can easily get messages from client A through a callback set up in the receivePeerMessage
method. This built-in callback ensures smooth receipt and handling of incoming messages from client A.
// Set up a callback to handle incoming direct messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('Received Direct Message:', messageList, fromConversationID);
});
Log out
To end a client’s session and disconnect them, just use the logout
method to log them out.
zim.logout();
Destroy the ZIM SDK instance
To completely remove the ZIM SDK instance, use the destroy
method. This will wipe out all traces of the SDK from the system.
zim.destroy();
Run a Demo
To fully test the features of ZEGOCLOUD’s In-app Chat SDK, try out the sample demo that is provided. The demo gives a good example of how to use the SDK’s capabilities.
Conclusion
If you’re new to web app development, 2024 is a great time to dive in. With user-friendly tools like ZEGOCLOUD SDK, you can easily add real-time messaging and more to your projects. This guide has given you the basics to get started. As you go along, remember that practice makes perfect. So, keep learning and building, and you’ll be a pro in no time!
Read more:
Web Application Development FAQ
Q1: What are the key stages in web application development?
The key stages in web application development include planning and research, where developers define the app’s purpose and target audience; design, where the user interface and experience are planned; development, where actual coding and database management are done; testing, to ensure everything works as expected and are bug-free; deployment, where the application goes live; and maintenance, to keep the application updated and operational over time.
Q2: What are the most popular programming languages and frameworks used in web application development?
Popular programming languages for web application development include JavaScript, Python, Ruby, PHP, and Java. JavaScript and its frameworks like React, Angular, and Vue.js are widely used for frontend development, while Node.js is popular for the backend. Python’s Django and Flask frameworks are also commonly used for backend development, as are Ruby on Rails for Ruby, and Laravel for PHP.
Q3: How important is security in web application development, and how can it be ensured?
Security is crucial in web application development to protect sensitive data and prevent malicious attacks. Developers ensure security by implementing practices like input validation, using HTTPS, securing data storage and transmission, employing proper authentication and authorization mechanisms, and regularly updating the application and its dependencies. Additionally, conducting security audits and penetration testing can help identify and mitigate potential vulnerabilities.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!