Call history is a very important feature of your call app. It’s a great way to provide your users with the information they need when they are in the middle of an important call.
The function consists of a list of calls made or received by the user. The information includes the date and time of each call, duration, as well as any contact details for the person on the other end of the line. This article will explain how to implement the history function in your app with ZEGOCLOUD.
Understanding call status
Before implementing the call history function, you need to understand the conversion logic of different call statuses.
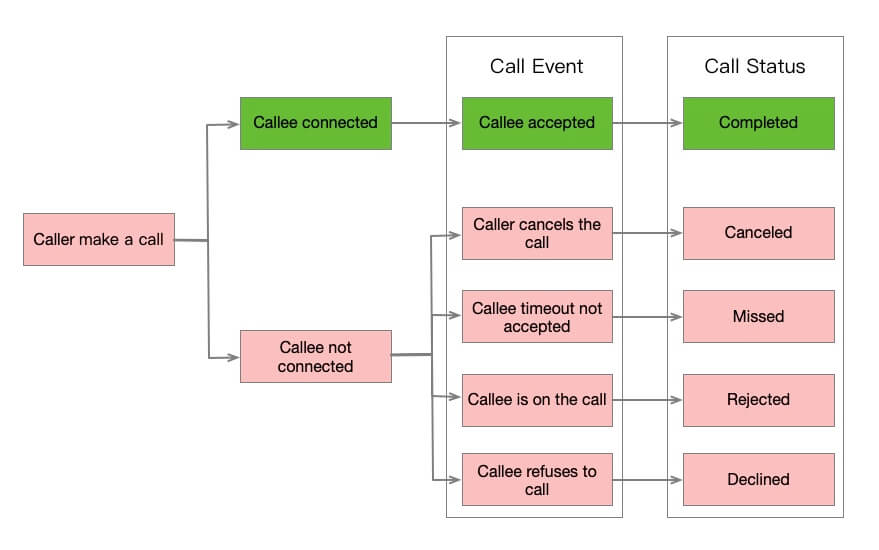
As shown in the figure, there are two types of call history.
- Connected
- Not connected
It is caused by 5 kinds of events, and finally, 5 kinds of call statuses are generated. See the table below for details.
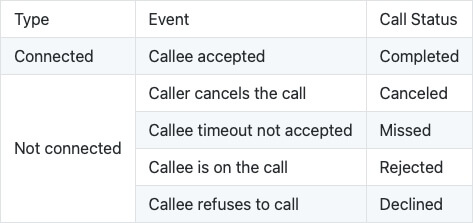
Call history implementation process
Through the explanation in the previous section, you have learned about the various call status in the call. By listening to the callback of the call event provided by the ZEGOCLOUD Call Kit, you can get all the call statuses.
But how to record these statuses and ensure that the status of the Caller and Callee are consistent?
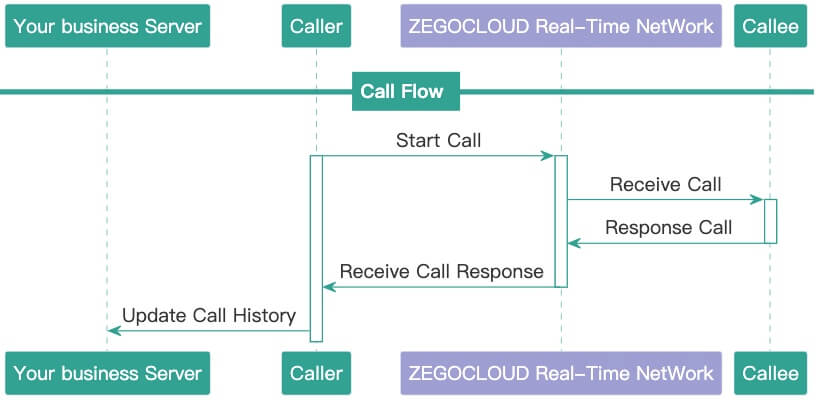
As shown in the figure, the call history is sent to your business server by the Caller. Call history is only uploaded by the Caller, which can avoid the problem of inconsistent call history between the Caller and Callee.
You may ask if the Caller is interrupted due to abnormal conditions such as network disconnection or application crash. If it is only reported by the Caller to your business server, will the call history be lost? The solution in this case is to use a local cache.
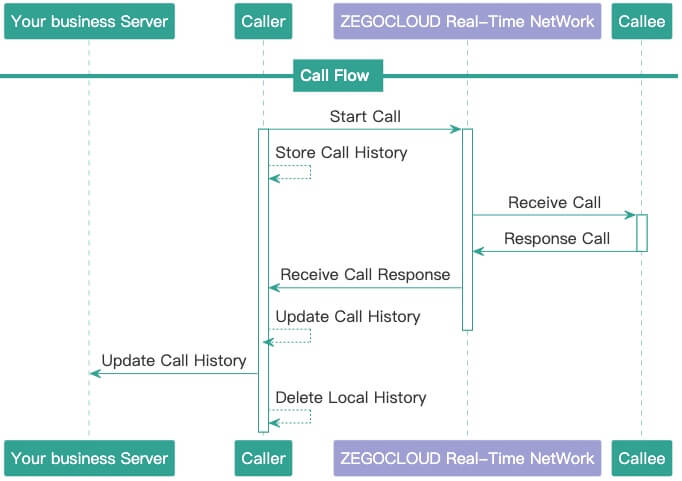
As shown in the figure, when a call starts, a call history is recorded locally, and when the call status is updated, the call history status is updated synchronously.
After the call history is successfully reported to the business server, the call record will be deleted.
In case of abnormal situations such as network disconnection or application crash, the call history will be wholly saved locally. When the app reconnects to the Internet, check the local call history. If call history has not been uploaded, upload them again.
Implement Call History Function
Now you should have understood the entire process of implementing call history, let’s see how to implement the call history function through ZEGOCLOUD Call Kit.
Here will explain how to record all call statuses. You can choose to record the required call status and call data according to your business needs.
1. Call Data
Before discussing specific practices, it is necessary to clarify the data that needs to be counted. A complete call record includes the following data:
- Caller name
- Caller ID
- Callee name
- Callee ID
- Call status
- Call start time
- Call duration
By sorting out the data that needs to be recorded, it can be determined that when the Caller reports data to the server, it needs to report the following data:
{
"call_id":"id123123",
"caller_name": "James",
"caller_id": "123456",
"callee_name": "Sara",
"calleee_id": "898989",
"call_status": 1,
"call_start_timestamp": 1671789222,
}
You may have questions, why not report call duration data?
I will answer this question for you in the function implement section.
2. Call completed status
The call completed status needs to monitor the onOutgoingCallAccepted callback method in ZegoUIKitEventHandle, which will be triggered when the callee clicks to accept the invitation. Since the call has just started at this time, no call duration data has been generated yet. so the call duration data will not be reported.
Refer to the article Call Duration Statistics for the call duration data, and use your business server statistics.
func onOutgoingCallAccepted(_ callID: String, callee: ZegoCallUser) {
let userID = UserManager.shared.loginUser?.userID ?? ""
let userName = UserManager.shared.loginUser?.userName ?? ""
let requestJson: [String : Any] = [
"call_id":callID,
"caller_name": userName,
"caller_id": userID,
"callee_name": callee.userName ?? "",
"calleee_id": callee.userID ?? "",
"call_status": 1,
"call_start_timestamp": callStartTimestamp,
]
uploadCallHistoryAPI(requestJson: requestJson)
}
3. Call canceled status
The call canceled state needs to listen to the onOutgoingCallCancelButtonPressed
callback method in ZegoUIKitEventHandle
, which is triggered when the caller cancels the call.
func onOutgoingCallCancelButtonPressed(_ callID: String, callee: ZegoCallUser) {
let userID = UserManager.shared.loginUser?.userID ?? ""
let userName = UserManager.shared.loginUser?.userName ?? ""
let requestJson: [String : Any] = [
"call_id":callID,
"caller_name": userName,
"caller_id": userID,
"callee_name": callee.userName ?? "",
"calleee_id": callee.userID ?? "",
"call_status": 2,
"call_start_timestamp": callStartTimestamp,
]
uploadCallHistoryAPI(requestJson: requestJson)
}
4. Call missed status
The call missed status needs to monitor the onOutgoingCallTimeout
callback method in ZegoUIKitEventHandle
, which will be triggered when the callee does not answer for more than 60 seconds.
The callees returned by this callback are an array because it supports inviting multiple people to call simultaneously. So if you need to do different logic processing in different call types.
- 1-on-1 call, After receiving the callback, fetches the first Callee information in the array to report the call timeout status.
- Group call, You need to wait for everyone to return the call timeout status before reporting the call status to the server.
func onOutgoingCallTimeout(_ callID: String, callees: [ZegoCallUser]) {
let userID = UserManager.shared.loginUser?.userID ?? ""
let userName = UserManager.shared.loginUser?.userName ?? ""
let requestJson: [String : Any] = [
"call_id":callID,
"caller_name": userName,
"caller_id": userID,
"callee_name": callees.first?.userName ?? "",
"calleee_id": callees.first?.userID ?? "",
"call_status": 3,
"call_start_timestamp": callStartTimestamp,
]
uploadCallHistoryAPI(requestJson: requestJson)
}
5. Call rejected status
The call was rejected status needs to listen to the onOutgoingCallRejected
callback method in ZegoUIKitEventHandle
, which is triggered when the callee is on a call.
func onOutgoingCallRejected(_ callID: String, callee: ZegoCallUser) {
let userID = UserManager.shared.loginUser?.userID ?? ""
let userName = UserManager.shared.loginUser?.userName ?? ""
let requestJson: [String : Any] = [
"call_id":callID,
"caller_name": userName,
"caller_id": userID,
"callee_name": callee.userName ?? "",
"calleee_id": callee.userID ?? "",
"call_status": 4,
"call_start_timestamp": callStartTimestamp,
]
uploadCallHistoryAPI(requestJson: requestJson)
}
5. Call declined status
The call was declined status needs to listen to the onOutgoingCallDeclined
callback method in ZegoUIKitEventHandle
, which is triggered when the callee actively refuses the call.
func onOutgoingCallDeclined(_ callID: String, callee: ZegoCallUser) {
let userID = UserManager.shared.loginUser?.userID ?? ""
let userName = UserManager.shared.loginUser?.userName ?? ""
let requestJson: [String : Any] = [
"call_id":callID,
"caller_name": userName,
"caller_id": userID,
"callee_name": invitee.userName ?? "",
"calleee_id": invitee.userID ?? "",
"call_status": 5,
"call_start_timestamp": callStartTimestamp,
]
uploadCallHistoryAPI(requestJson: requestJson)
}
Conclusion
One of the most common reasons people stop using a call app is because they forget to record or save their call history. Implementing this feature will allow users to easily keep track of their past calls, and also make it easy to share them with others.
Now you should know how to implement the call history function, let’s act now!
Read More
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!