In the complex landscape of telecommunications, call duration stands as a simple yet powerful metric. This measure, the length of a phone call from start to finish, plays a critical role in areas ranging from billing to customer service. Despite its simplicity, it can unlock a wealth of insights when properly utilized. This article explores the significance of call duration, its applications, and its potential to optimize resources and drive business growth.
What is Call Duration?
Calling duration refers to the length of time a phone call lasts, from the moment the call is initiated (picked up or answered) to the moment it is ended (hung up). This term is commonly used in telecommunications to measure the usage of phone services. It can be particularly important in scenarios where billing is based on the length of the call, such as in certain mobile or long-distance calling plans.
Call duration formula
The calculation formula is as follows:
Call duration = call end timestamp - call start timestamp
You only need to get the call start timestamp
and call end timestamp
to calculate the call duration.
However, there are some exceptions. For example, in the case of a network interruption or app crash, how to ensure the accuracy of calculation? Furthermore, how to ensure that the billing logic is safe and that there is no manipulation?
Let’s see how to tackle these issues.
Real-time display of call duration
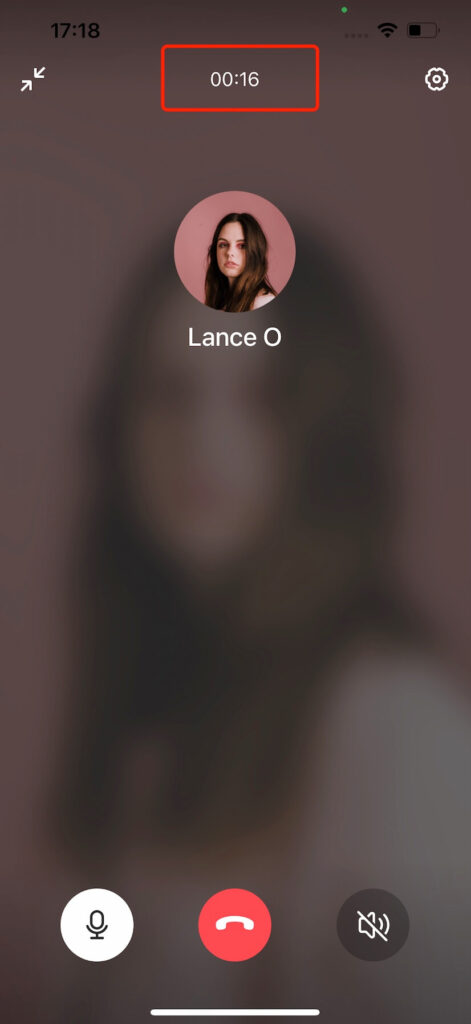
The calling duration function is often implemented on the client side. Real-time and fluency of timing updates need special attention.
The processing logic is as such:
- the App listens to the call
start event
- The timer starts, polls once per second, and adds 1 second to the call duration
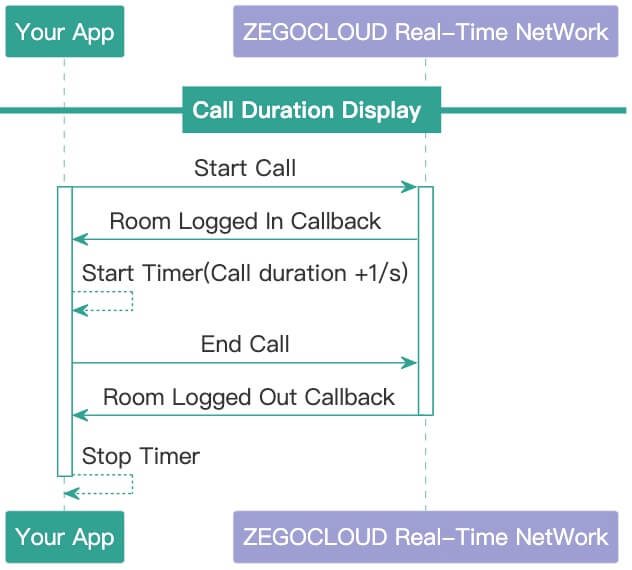
ZEGOCLOUD UIKits determines when the user starts and ends a call by monitoring the callback events of the user joining and exiting the call room.
ZEGOCLOUD UIKits provides RoomStateChanged
callback notifications. In particular, you can get notified when:
- Joining a call room.
- Being kicked out of the call room.
- Exiting the call room.
We can get the start
and end timestamp
of the call through it.
1. Add event listener
First, you need to call the ZegoUIKit.addRoomStateChangedListener
method to listen for room state changes.
private void addRoomStateChangedListener() {
roomStateChangedListener = new RoomStateChangedListener() {
@Override
public void onRoomStateChanged(String s, ZegoRoomStateChangedReason zegoRoomStateChangedReason, int i, JSONObject jsonObject) {
switch (zegoRoomStateChangedReason) {
case LOGINED:
roomID = s;
addTextView();
startTimer();
break;
case LOGOUT:
case KICK_OUT:
case RECONNECT_FAILED:
stopTimer();
break;
default:
break;
}
}
};
ZegoUIKit.addRoomStateChangedListener(roomStateChangedListener);
}
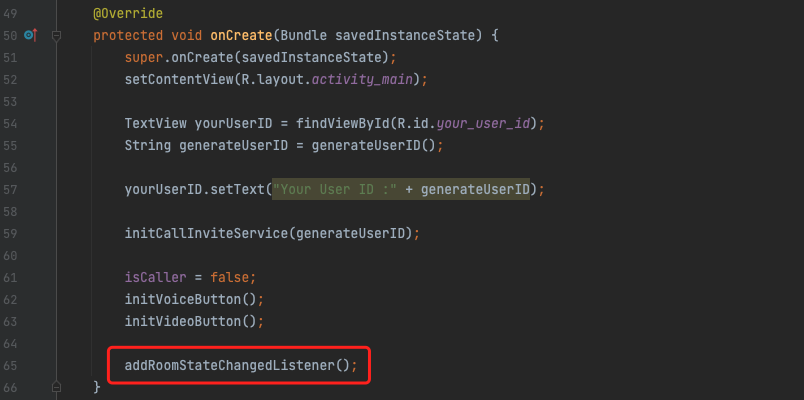
2. Callback event handling
In the RoomStateChanged
callback event, we need to handle two types of events:
- call start
- call end.
The call start
implements the following logic:
- View to display call duration.
- Start the timer
The call end
implements:
- Stop the timer.
public void onRoomStateChanged(String s, ZegoRoomStateChangedReason zegoRoomStateChangedReason, int i, JSONObject jsonObject) {
switch (zegoRoomStateChangedReason) {
case LOGINED:
roomID = s;
addTextView();
startTimer();
break;
case LOGOUT:
case KICK_OUT:
case RECONNECT_FAILED:
stopTimer();
break;
default:
break;
}
}
2.1 Added view
Next, you need to add a TextView
to display the calling duration.
private void addTextView() {
ZegoUIKitPrebuiltCallFragment fragment = ZegoUIKitPrebuiltCallInvitationService.getPrebuiltCallFragment();
ConstraintLayout rootView = (ConstraintLayout) fragment.getView();
textView = new TextView(MainActivity.this);
textView.setTextColor(Color.WHITE);
ConstraintLayout.LayoutParams params = new ConstraintLayout.LayoutParams(-2, -2);
params.startToStart = ConstraintLayout.LayoutParams.PARENT_ID;
params.endToEnd = ConstraintLayout.LayoutParams.PARENT_ID;
params.topToTop = ConstraintLayout.LayoutParams.PARENT_ID;
params.topMargin = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP,20,getResources().getDisplayMetrics());
rootView.addView(textView, params);
}
2.2 Start timer
When the call starts, you need to create a Timer
, poll once a second, and update the text in TextView
.
private void startTimer() {
duration = 0;
timer = new Timer();
uploadCallDuration(duration);
TimerTask task = new TimerTask() {
@Override
public void run() {
duration += 1;
handler.post(new Runnable() {
@Override
public void run() {
textView.setText(transToHourMinSec(duration));
}
});
}
};
timer.schedule(task, 0, 1000);
}
2.3 Stop timer
When the call ends, you need to call timer.cancel()
to end the timer.
private void stopTimer() {
timer.cancel();
}
Call recording and call billing
Call records, as proof of payment. Accuracy and safety are paramount. In various abnormal situations, it is also necessary to accurately count the duration of a call. Therefore, the call duration cannot be reported only at the end of the call. If an abnormal situation occurs, such as network disconnection, application exit, etc. the calling duration cannot be counted. Our solution is to regularly report it. For example, report once every 30 seconds. You can also set the reporting frequency according to your billing rules. If billing is by the minute, the frequency of once every 30 seconds is the most appropriate.
1. Upload Call Duration
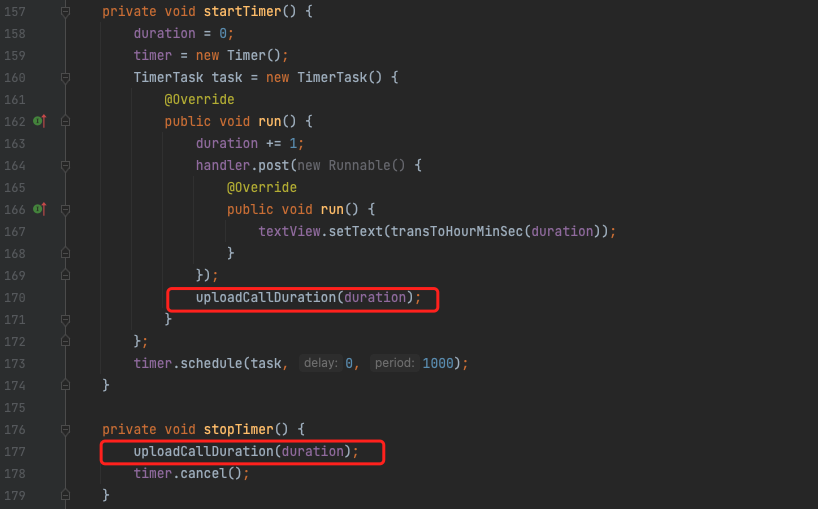
private void uploadCallDuration(int duration) {
int interval = 30;
if ((duration - 1) % interval != 0) {
return;
}
if (!isCaller) {
return;
}
Map<String, Object> param = new HashMap<String, Object>();
param.put("roomID", roomID);
param.put("duration", duration);
// request bussiness server API to upload call duration
requestBussinessServerAPI(param);
}
There are two things to note here:
1. In order to ensure that the call is recorded at the beginning when calculating the reporting frequency, use duration - 1
.
if ((duration - 1) % interval != 0) {
return;
}
2. In order to avoid repeated reporting, the call duration is reported by the caller. So you need to judge whether you are the caller.
if (!isCaller) {
return;
}
Conclusion
We have seen together the commonly used calculation method for call duration. However, it will vary according to different business scenarios and billing methods.
Of course, in case of necessity, you can contact us. We will formulate the best plan for you.
You can also download the sample code for this article from here.
Read more
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!