Whiteboards have always been essential for collaboration, but with ZEGOCLOUD whiteboard SDK, you can build a smart whiteboard that takes your team’s productivity to the next level. With advanced features such as real-time collaboration, video conferencing, and cloud-based storage, the ZEGOCLOUD SDK makes building a highly functional and intuitive whiteboard that meets your team’s needs easy.
How to Create a Smart Interactive Whiteboard for Collaboration
ZEGOCLOUD Whiteboard SDK helps developers create smart interactive whiteboards. It’s a comprehensive solution for modern organizations to improve collaboration and productivity. Therefore, an innovative interactive whiteboard built using the ZEGOCLOUD Whiteboard SDK can enhance team collaboration.
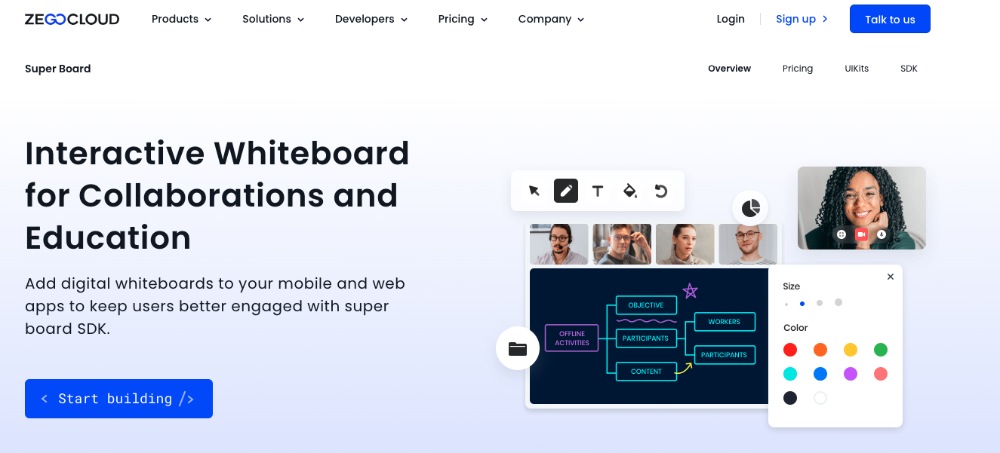
Features of the ZEGOCLOUD Whiteboard SDK
1. Real-time collaboration
The SDK allows multiple users to collaborate on the same whiteboard in real time, enabling team members to work together seamlessly regardless of location.
2. Video conferencing
Users can communicate via video and audio chat while working on the same whiteboard. This feature ensures that all team members are on the same page and can discuss ideas more effectively.
3. Cloud-based storage
The cloud stores all whiteboards created using the SDK, ensuring users can access them from anywhere, anytime, and on any device.
4. Whiteboard customization
The SDK allows developers to customize the whiteboard’s interface to match their brand, ensuring a consistent look and feel.
5. Cross-platform compatibility
The SDK is compatible with various operating systems and devices, making it easy for developers to create a whiteboard solution accessible from any device.
Preparation
- A ZEGOCLOUD developer account – Sign up
- A computer multimedia and web support
- Web development fundamentals
Steps to Build Smart Whiteboard with ZEGOCLOUD SDK
Building a smart whiteboard with ZEGOCLOUD SDK is easy. Just follow a few simple steps to create a highly functional and intuitive whiteboard for your team. In this section, we’ll guide you through building a smart whiteboard using the ZEGOCLOUD SDK.
Follow the steps below to get started:
1. Download the SDK
Getting the ZegoSuperBoard SDK and ZegoExpress-Video SDK is a breeze using NPM. By executing the code below, you can easily download these SDKs and benefit from their advanced capabilities. You’ll be able to leverage their powerful features in no time and start building your smart whiteboard with confidence.
npm i zego-superboard-web
npm i zego-express-engine-webrtc
2. Integrate the SDK
To incorporate the Super Board SDK from ZEGOCLOUD into your project, use the following JavaScript code:
import { ZegoSuperBoardManager } from 'zego-superboard-web';
import {ZegoExpressEngine} from 'zego-express-engine-webrtc'
3. Initialize the SDKs
Using NPM, we added two SDKs. For us to fully utilize their tremendous functionality, we must initialize them.
ZegoExpress-Video SDK
Using your AppID
and Server URL
from the ZEGOCLOUD Admin Console, instantiate the ZegoExpressEngine
class.
// Initialize the ZegoExpressEngine instance
const zg = new ZegoExpressEngine(appID, server);
ZegoSuperBoard SDK
Initialize the SDK using the ZegoExpressEngine
instance by calling the init method after retrieving the ZegoSuperBoard
instance using getInstance in the ZegoSuperBoardManager. With ease, begin utilizing the ZegoSuperBoard SDK.
<!-- Parent container to be mounted to -->
<div id="parentDomID"></div>
// Obtain the ZegoSuperBoard instance.
zegoSuperBoard = ZegoSuperBoardManager.getInstance();
// Initialize ZegoSuperBoard.
const result = await zegoSuperBoard.init(zg, {
parentDomID: 'parentDomID', // D of the parent container to be mounted to.
appID: 0, // The AppID you get.
userID: '', // User-defined ID
token: '' // The Token you get that is used for validating the user identity.
});
4. Configure and monitor event callbacks
By monitoring the event callbacks after the SuperBoard has been initialized, you can tailor them to your application’s needs. These include error notifications, remote whiteboard file addition, deletion, and switching.
// Callback of the listening-for error. zegoSuperBoard.on('error', function(errorData) {
// Error code, error prompt
conosole.log(errorData.code, errorData.message)
});
// Monitor paging and scrolling in the whiteboard via event listeners.
zegoSuperBoard.on('superBoardSubViewScrollChanged', function(uniqueID, page, step) {
});
// You can listen for the remote operation of zooming in or out on a whiteboard.
zegoSuperBoard.on('superBoardSubViewScaleChanged', function(uniqueID, scale) {
});
// Remote whiteboard addition event listener.
zegoSuperBoard.on('remoteSuperBoardSubViewAdded', function(uniqueID) {
});
// Monitor the remote whiteboard destruction operation.
zegoSuperBoard.on('remoteSuperBoardSubViewRemoved', function(uniqueID) {
});
// Listen for remote whiteboard switching.
zegoSuperBoard.on('remoteSuperBoardSubViewSwitched', function(uniqueID) {
});
// Listen for remote Excel sheet switching.
zegoSuperBoard.on('remoteSuperBoardSubViewExcelSwitched', function(uniqueID, sheetIndex) {
});
// Listen for remote whiteboard permission changes.
zegoSuperBoard.on('remoteSuperBoardAuthChanged', function(data) {
console.log(data.scale, data.scroll)
});
// Listen for remote whiteboard element permission changes.
zegoSuperBoard.on('remoteSuperBoardGraphicAuthChanged', function(data) {
console.log(data.create, data.delete, data.move, data.update, data.clear)
});
5. Log in to a room
Use the loginRoom
the method with the following parameters to access a roomroomID, token, userID, and userName. You can also provide a configuration object if necessary. If the roomID is not found, the method will automatically log you in.
const result = await zg.loginRoom(roomID, token, {userID, userName}, {userUpdate: true});
6. Create a whiteboard
Both common and file-based whiteboards are supported by Super Board. A file whiteboard is formed based on an existing file, whereas an ordinary whiteboard can be made by selecting its width, height, and number of pages. A valid login is required before making a whiteboard; a whiteboard can only be made after that.
A room can only have a maximum of 50 whiteboards; if the room currently has 50 whiteboards, adding more will not work. Use the querySuperBoardSubViewList method to find the number of whiteboards now present in a room.
Create a common whiteboard.
const model = await zegoSuperBoard.createWhiteboardView({
name: '', // Whiteboard name
perPageWidth: 1600, // Whiteboard page width
perPageHeight: 900, // Whiteboard page height
pageCount: // Page count of a whiteboard
});
Create a file whiteboard.
Get the file’s fileID
before creating a file-based whiteboard. To upload the file, please refer to Shared File Management for details.
const model = await zegoSuperBoard.createFileView({
fileID // fileID of a file, which is the unique identifier returned after a file is successfully uploaded.
});
7. Mount the current whiteboard
The ZegoSuperBoard SDK cannot mount the whiteboard to the parent container if a client logs in to a room with a whiteboard because it is unsure of the parent container’s existence. Using the querySuperBoardSubViewList
andswitchSuperBoardSubView
APIs, you can alert and mount the whiteboard to the parent container after a successful login.
// Obtain SuperBoardSubViewList.
const superBoardSubViewList = await zegoSuperBoard.querySuperBoardSubViewList();
// Obtain SuperBoardView.
const superBoardView = zegoSuperBoard.getSuperBoardView();
// Obtain the current SuperBoardSubView.
const zegoSuperBoardSubView = superBoardView.getCurrentSuperBoardSubView()
// Obtain the model corresponding to SuperBoardSubView.
const model = zegoSuperBoardSubView.getModel();
// Obtain a uniqueID of the whiteboard to be mounted.
const uniqueID = model.uniqueID;
// Get the file type and obtain sheetIndex for Excel whiteboard.
let sheetIndex;
const fileType = model.fileType;
if (fileType === 4) {
// Excel whiteboard
const sheetName = zegoSuperBoardSubView.getCurrentSheetName();
// Retrieve the list of sheets for the current Excel file.
const zegoExcelSheetNameList = zegoSuperBoardSubView.getExcelSheetNameList();
// To get the sheetIndex from zegoExcelSheetNameList, use the corresponding sheetName.
sheetIndex = zegoExcelSheetNameList.findIndex(function(element, index) {
return element === sheetName;
});
}
// Mount the current whiteboard.
const result = await superBoardView.switchSuperBoardSubView(uniqueID, sheetIndex);
8. Verify whiteboard creation
Run the project on various devices while logging in to room ID to test it. When you use your mouse to draw a ride of any window’s ZegoSuperBoardView, the image will appear on the other window.
9. Destroy a whiteboard
After the current whiteboard is destroyed, the ZegoSuperBoard SDK will automatically transition to a new one.
const result = await zegoSuperBoard.destroySuperBoardSubView(uniqueID)
Run a Demo
You can check out the features via the sample demo if you want to build intelligent whiteboard capabilities for your apps.
You may also like: Top 5 Whiteboard App Lists You Will Like
Conclusion
Building an intelligent whiteboard using ZEGOCLOUD SDK is an exciting and innovative way to enhance collaboration and communication. With its powerful features and user-friendly interface, it provides a seamless experience for both individuals and teams. Following the steps outlined in this guide, you can easily create a cutting-edge innovative interactive whiteboard solution that impresses you.
Read more:
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!