Looking to create a random chat app that connects users at random and fosters new connections? In this article, we’ll share expert tips on developing a chat app that stands out from the crowd and delivers an engaging user experience using ZEGOCLOUD In-app Chat SDK. From selecting a niche to integrating exciting features, you’ll learn everything you need to know to build a random chat app that people will love to use.
Must-have features of a Random Chat App
Random chat apps have been gaining popularity in recent years due to their ability to connect people from different parts of the world. They provide a fun and engaging way to meet new people and make friends. However, not all random chat apps are created equal. The best ones have certain must-have features that make them stand out from the rest.
- Privacy and Security: A random chat app must have robust privacy and security features to protect users’ personal information. This is important to ensure that users have control over what information they share and with whom. It also helps prevent harassment and ensure a safe and respectful environment.
- Easy-to-Use Interface: A good random chat app should have an intuitive interface with easy-to-use controls. This allows users to quickly find and connect with other users, as well as filter and search for specific interests. An easy-to-use interface also makes the app more accessible to users of all ages and technical abilities.
- Engaging Features: To keep users entertained and engaged, a random chat app should have a variety of features. This can include games, virtual gifts, and other interactive features. These features help users connect with others in a fun and interactive way, making the app more enjoyable and memorable.
How to Develop a Random Chat App with ZEGOCLOUD
Developing a random chat app can be a daunting task, but with the right tools and resources, it can be a smooth and successful process. One such tool is the ZEGOCLOUD In-app Chat SDK, which provides developers with the necessary components to build a robust and secure random chat app.
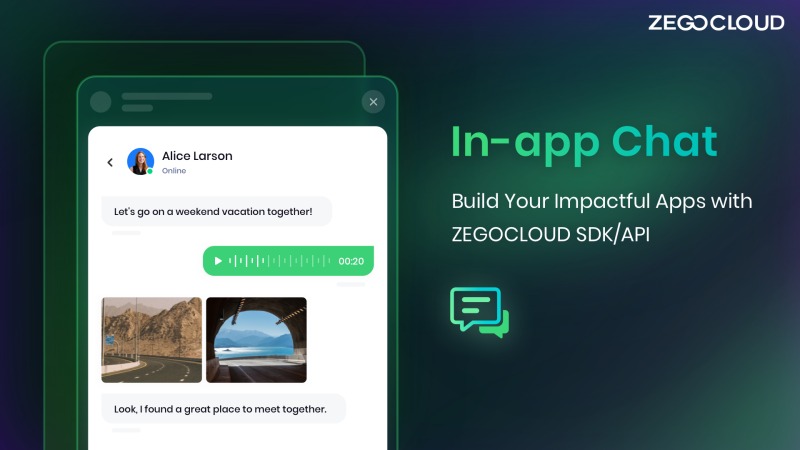
In-app Chat SDK is a versatile tool designed to integrate chat functionality into various applications seamlessly. It supports real-time messaging, including text, voice, and video communications, across iOS, Android, and Web platforms.
This chat SDK is known for its rich media support, allowing the sharing of images, videos, and documents, enhancing user engagement. Notably, it offers a customizable user interface, which developers can adapt to align with their application’s design. The SDK’s scalability makes it suitable for both small and large-scale applications.
Key features:
- provides real-time messaging capabilities, allowing users to communicate with each other instantly.
- also offers push notifications, which help users stay informed about new messages even when the app is not open.
- uses end-to-end encryption to ensure that all messages and data are secure and protected from unauthorized access.
- offers a customizable user interface, allowing developers to tailor the app to their specific needs and preferences.
- supports multiple platforms, including iOS, Android, and the Web, making building a cross-platform chat app easier.
Moreover, ZEGOCLOUD proudly introduces its UIKits SDK, an innovative solution designed to make the creation of a random chat app more straightforward and faster than ever. This comprehensive SDK equips developers with a range of user-friendly tools and features, streamlining the development process for iOS, Android, Flutter, and React Native platforms. Whether you are looking to build a random chat app like Omegle from scratch or enhance an existing one, the ZEGOCLOUD UIKits SDK is your go-to resource.
Steps on How to Build a Random Chat App
In this section, you’ll learn how to create a random chat app using ZEGOCLOUD Chat SDK. Follow the instructions below to build your own app and connect with people from around the world:
Preparation
- ZEGOCLOUD developer account – sign up
- A computer with internet connectivity.
- Access to the Admin console to create a new project.
- Basic knowledge of web development
- Have NodeJS installed on your system
1. Integrate the SDK
The first step to building a random chat app with ZEGOCLOUD is to integrate the In-chat SDK into your project. The following steps can be taken by you to accomplish this:
a. To install the dependencies:
npm i @zegocloud/zimkit-vue
b. Import ZIMKiti18n
to your project’s main.js
file.
import { ZIMKiti18n } from '@zegocloud/zimkit-vue';
// Add i18n to the Vue instance.
new Vue({
i18n: ZIMKiti18n,
}).$mount('#app');
2. Initialize In-app Chat SDK and login
To use In-app Chat UI components, create an instance of the Chat Kit and initialize the SDK with the init
method. Login by calling connectUser
with user info
and Token
, authentication must pass for successful login.
<template>
<Common></Common>
</template>
<script>
// Integrate the SDK using NPM
import { ZIMKitManager, Common } from '@zegocloud/zimkit-vue';
import '@zegocloud/zimkit-vue/index.css';
export default {
name: 'App',
components: {
Common
},
data() {
return {
appConfig: {
appID: 0, // The AppID you get from ZEGOCLOUD Admin Console.
serverSecret: '' // The serverSecret you get from ZEGOCLOUD Admin Console.
},
// The userID and userName is a strings of 1 to 32 characters.
// Only digits, letters, and the following special characters are supported: '~', '!', '@', '#', '$', '%', '^', '&', '*', '(', ')', '_', '+', '=', '-', '`', ';', ''', ',', '.', '<', '>', '/', '\'
userInfo: {
// Your ID as a user.
userID: '',
// Your name as a user.
userName: '',
// The image you set as a user avatar must be a network image. e.g., https://storage.zego.im/IMKit/avatar/avatar-0.png
userAvatarUrl: ''
},
}
},
async created() {
const zimKit = new ZIMKitManager();
const token = zimKit.generateKitTokenForTest(this.appConfig.appID, this.appConfig.serverSecret, this.userInfo.userID);
await zimKit.init(this.appConfig.appID);
await zimKit.connectUser(this.userInfo, token);
}
}
</script>
3. Start a one-on-one chat
Retrieve the userID
using your business logic for the peer user you wish to chat with. Fill in the userID parameter in the code snippet below and execute it.
Note: To initiate a one-on-one or group chat successfully, ensure the peer user(s) you wish to chat with have logged in to the In-app Chat UIKit at least once. Otherwise, you may encounter errors.
createChat(userID: string) {
ZIMKitChatListVM.getInstance().initWithConversationID(userID, ZIMKitConversationType.ZIMKitConversationTypePeer);
}
4. Start a group chat
Retrieve the userIDList
and groupName
for the users you want to add to the group chat using your business logic. Fill in the respective parameters in the code snippet below and execute it.
createGroupChat(groupName: string, userIDList: string[]) {
ZIMKitManager.getInstance()
.createGroup(groupName, userIDList)
.then((data) => {
const { groupInfo, errorUserList } = data;
const { baseInfo } = groupInfo;
if (errorUserList.length) {
// Implement the logic for the prompt window based on your business logic when there is a non-existing user ID in the group.
} else {
// Directly enter the chat page when the group chat is created successfully.
const groupID = baseInfo.groupID
ZIMKitChatListVM.getInstance().initWithConversationID(groupID, ZIMKitConversationType.ZIMKitConversationTypeGroup);
}
}).catch((error) => {
// Implement the logic for the prompt window based on the returned error info when failing to create a group chat.
});;
}
5. Join a group chat
Retrieve the groupID
for the group chats you wish to join using your business logic. Fill in the groupID
parameter in the code snippet below and execute it.
joinGroupChat(groupID: string) {
ZIMKitManager.getInstance()
.joinGroup(groupID)
.then((data) => {
const groupID = data.groupInfo.baseInfo.groupID;
ZIMKitChatListVM.getInstance().initWithConversationID(groupID, ZIMKitConversationType.ZIMKitConversationTypeGroup);
});
}
Run a Demo
If you’re interested in building a random chat app, you can use the sample demo to get started
How Much Does It Cost to Develop a Random Chat App?
The cost of developing a random chat app depends on a wide range of factors that shape the scope, complexity, and timeline of the development process. These factors include:
- App complexity: Basic chat apps cost less, but if your app includes video filters, AI-based moderation, gamified coin systems, or real-time translation, the cost goes up.
- Platform choice: Native iOS/Android development is typically more expensive than cross-platform frameworks like Flutter or React Native.
- Features and functionalities: Core features include one-on-one video/audio chat, text chat, matchmaking filters, user reporting/blocking, and in-app purchases.
- UI/UX design: A sleek, intuitive user interface boosts user retention but requires more time and design effort.
- Technology stack: Using scalable and real-time friendly tools like WebRTC, ZEGOCLOUD, Firebase, or AWS will influence both cost and performance.
- Team size and expertise: The more team members (developers, designers, testers, PMs) you involve, the higher the budget.
- Time frame: A tight deadline often leads to higher costs due to overtime or needing more developers.
- Developer region and hourly rates: Developer location heavily affects cost. For instance, US developers may charge $100–$200/hour, while teams in South Asia may charge $20–$50/hour.
- Hosting and security: Real-time chat apps require reliable servers and secure communication, especially for video transmission and personal data protection.
Based on the above factors, the average development cost for a basic random chat app is typically between $20,000 to $30,000 for core functionality.
However, if you’re building a feature-rich or customized solution—for example, a cross-platform VoIP (Voice over Internet Protocol) app with smart matching, moderation, and monetization—the total budget could rise to $50,000 to $100,000 or more.
How ZEGOCLOUD Can Help with Your Random Chat App
Building a random chat app from scratch can be time-consuming and costly—especially when it comes to handling real-time video, audio, and signaling infrastructure. That’s where ZEGOCLOUD comes in.
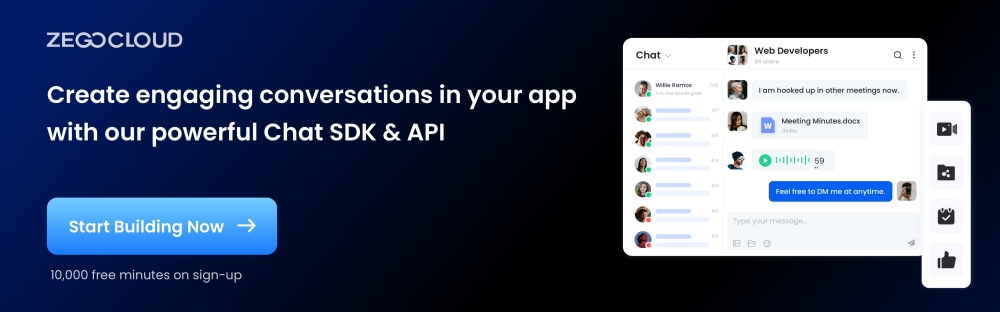
With ZEGOCLOUD’s ready-to-use 1-on-1 video chat SDK, real-time messaging, and content moderation APIs, you can focus on user experience and product logic while leaving the complex backend to us. Whether you’re launching an MVP or scaling to millions of users, ZEGOCLOUD ensures:
- High-quality, low-latency video/audio chat
- Easy integration with mobile and web apps
- Built-in features like gender filters, coin-based calls, and moderation
- Global infrastructure for smooth connections worldwide
Start faster, scale smarter—ZEGOCLOUD helps you bring your random chat idea to life with less code, lower cost, and better performance.
Conclusion
Building a random chat app is an exciting project that can connect people from all over the world. By following the steps outlined in this guide, you can use ZEGOCLOUD to create your own chat app with in-app chat UI components and features like one-on-one and group chats. Whether you’re building an app for personal or business purposes, this guide can help you get started.
Read More
FAQ
Q1: What are the essential features needed to include for the random chat app?
Essential features for a Random Chat App include user anonymity options, real-time text/video/audio chat functionalities, user matching algorithms, user reporting and blocking capabilities for safety, and a simple, intuitive user interface.
Q2: How do I ensure user privacy and security in the App?
To ensure user privacy and security, implement end-to-end encryption for chats, enforce strict data protection policies, regularly update security protocols, and provide users with privacy settings to control their information and interaction preferences.
Q3: How can I monetize my Random Chat App?
Monetization strategies for a Random Chat App can include in-app purchases (like special features or customizations), subscription models for premium services, targeted advertising based on user preferences, and partnerships or sponsorships.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!