Integrating virtual gifts into your live streaming app is a fantastic way to boost engagement and create a new revenue channel. Start by understanding your audience’s preferences and designing a diverse range of appealing virtual gifts. Next, develop a seamless interface where viewers can easily purchase and send these gifts during live streams. This article will explain how to implement the virtual gift function with ZEGOCLOUD.
What is Virtual Gifts in Live Streaming?
Virtual gifts are a feature in live streaming that enhances viewer interaction within social apps. They allow the audience to send digital items, like a cup of coffee or a car, to hosts during broadcasts. This feature makes the streaming experience more interactive and lively, enabling viewers to show their support in a fun and meaningful way.
These gifts not only foster a stronger connection between hosts and their audience but also provide a monetization avenue for creators. By sending virtual gifts, viewers can actively participate in the stream, helping to create a vibrant and supportive community atmosphere.
How to Ensure the Safety and Stability of Virtual Gift
Virtual gifts are exchanged for money. To ensure the safety and stability of the process, one needs a stable system of sending virtual gifts. Since virtual gifts involve expensive consumption, they cannot be given directly to the host by the audience. Your business server must verify that the gift-giving operation is safe and reliable.
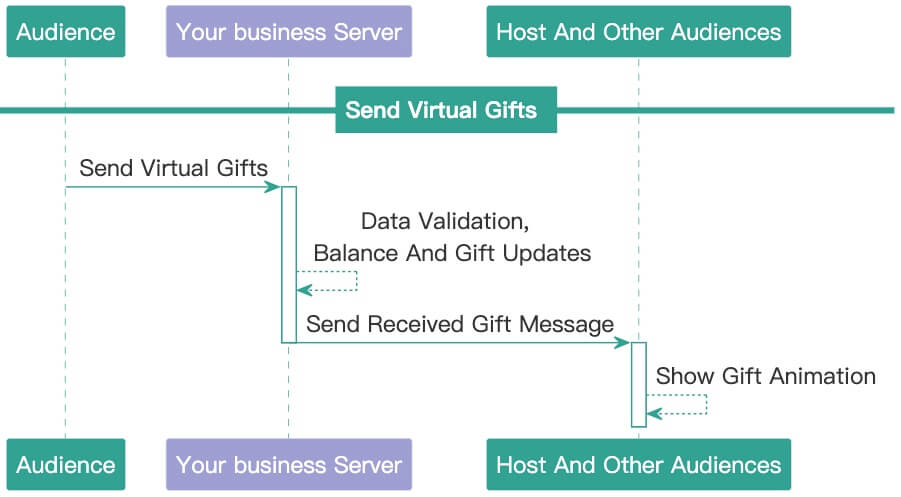
The figure shows that the audience needs to call your business server API first to send a virtual gift. The server will:
- Verify the authenticity of the data to ensure that the user balance is sufficient.
- Deduct the user balance.
- Update the number of the host’s gifts.
- Send a gift message to the host and the audience.
After receiving the gift message, it will display the virtual gift animation.
How to Notify the Host of Receiving a Gift
The figure shows that ZEGOCLOUD Real-Time Network provides a signaling channel for message transmission. Your business server can directly call the API of ZEGOCLOUD Real-Time Network to send messages.
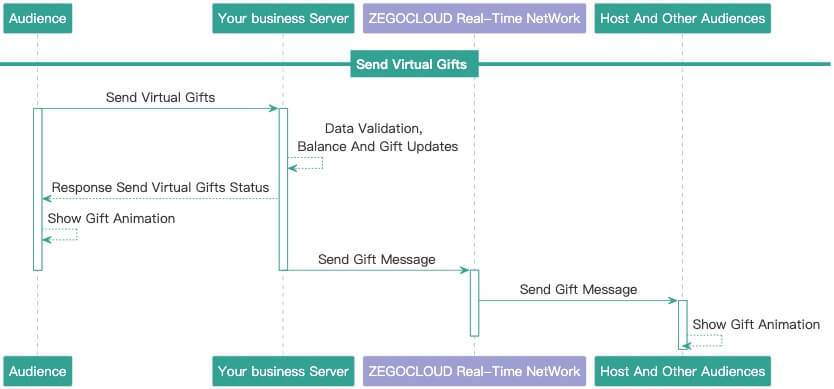
When the audience pays for the gift, the host receives the gift and responds to the audience. For this to happen, the signaling channel for sending messages to the host must be reliable.
ZEGOCLOUD Real-Time Network provides reliable and unreliable signaling channels. You choose an appropriate signaling channel according to your business requirements.
How to Ensure Service Stability Under High Concurrency
In live streaming scenarios, one must consider high concurrency. Tens of thousands of live streaming are common concurrency. Often, there is a sudden increase in gifts at a particular time. For example, the host performs a show in PK and invites the audience to give gifts, causing the number of gifts to increase sharply in a short period.
Every time the audience sends a gift, the server sends a gift message to the host and the audience. Therefore, exceeding the signaling interface call frequency limit.
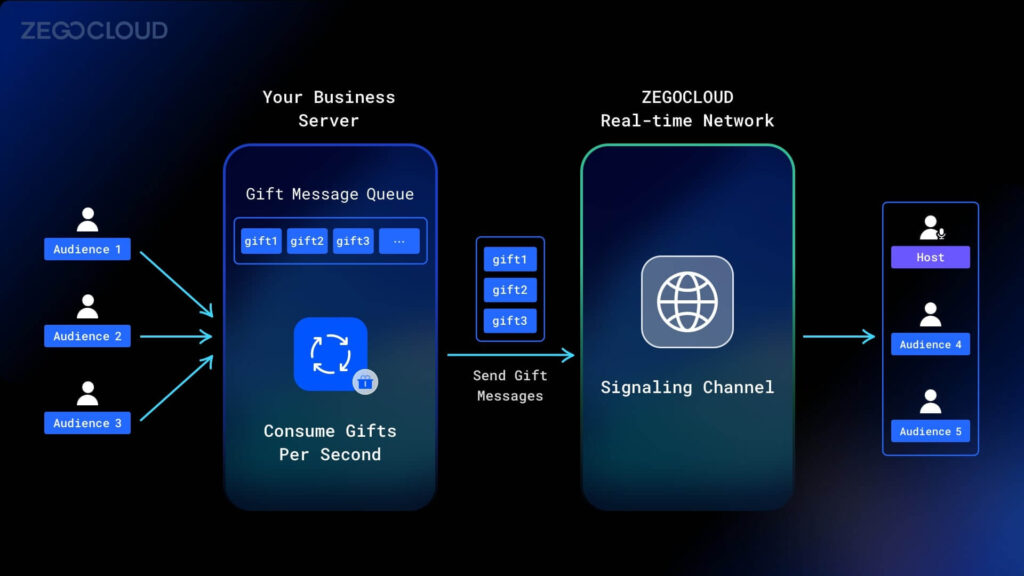
So if your application has many users, tens of thousands of rooms simultaneously exist. In this case, you need to:
- build a message queue on your server.
- consume the gift-giving messages in the queue once per second.
- combine the gift-giving messages in the same room into one message.
- call the send message of ZEGOCLOUD Real-Time Network API to send gift messages to hosts and audiences.
How to Display Gift Animation
Now the gift animations in the room are getting more dazzling and challenging to realize. However, thanks to the mature third-party animation library, the realization of animation becomes easy.
The designer must design and output the animation file in the corresponding format. One can achieve cool animation effects by using the animation library to display the file on the screen. Commonly used third-party animation libraries include Lottie, SVGA, and so on.
How to Implement the Virtual Gift Function with ZEGOCLOUD
The whole process of sending gifts has been understood above, and the following will explain how to realize this process.
1. Add a gift entry
UIKit provides the addButtonToBottomMenuBar
API to add a bottom button. You can add a gift button through this API and listen to the click event.
Live Audio Room Kit:
@override
Widget build(BuildContext context) {
return SafeArea(
child: Stack(
children: [
ZegoUIKitPrebuiltLiveAudioRoom(
appID: yourAppID,
appSign: yourAppSign,
userID: localUserID,
userName: 'user_$localUserID',
roomID: widget.roomID,
config: (widget.isHost
? ZegoUIKitPrebuiltLiveAudioRoomConfig.host()
: ZegoUIKitPrebuiltLiveAudioRoomConfig.audience())
..takeSeatIndexWhenJoining = widget.isHost ? 0 : -1
..bottomMenuBarConfig =
ZegoBottomMenuBarConfig(maxCount: 5, audienceExtendButtons: [
ElevatedButton(
style: ElevatedButton.styleFrom(
fixedSize: const Size(40, 40),
shape: const CircleBorder(),
),
onPressed: () {
_sendGift();
},
child: const Icon(Icons.blender),
)
]),
),
],
),
);
}
Live Streaming Kit:
@override
Widget build(BuildContext context) {
return SafeArea(
child: Stack(
children: [
ZegoUIKitPrebuiltLiveStreaming(
appID: yourAppID,
appSign: yourAppSign,
userID: localUserID,
userName: 'user_$localUserID',
liveID: widget.liveID,
config: (widget.isHost
? ZegoUIKitPrebuiltLiveStreamingConfig.host()
: ZegoUIKitPrebuiltLiveStreamingConfig.audience())
..bottomMenuBarConfig =
ZegoBottomMenuBarConfig(maxCount: 5, audienceExtendButtons: [
ElevatedButton(
style: ElevatedButton.styleFrom(
fixedSize: const Size(40, 40),
shape: const CircleBorder(),
),
onPressed: () {
_sendGift();
},
child: const Icon(Icons.blender),
)
]),
),
],
),
);
}
}
2. Implement the gift-sending API
When the audience clicks on the gift to send, it calls the gift-sending API of your business server. Request parameters can refer to:
{
"room_id": "room888", // Live Room ID
"user_id": "user987", // The user ID of the sender
"user_name": "James", // The user name of the sender
"gift_type": 1001, // Gift type
"gift_cout": 2, // Number of gifts
"access_token": "fasd2r34dfasd...fasdf", // The token used for authentication
"timestamp": 1670814533, // Request timestamp
}
You can add or delete request parameters according to your business needs. When an audience initiates a gift request, your business server will:
- judge whether the user’s balance is sufficient based on the requested parameters.
- deduct fees from the user’s balance.
- increase the amount of the host’s gift.
After processing, the corresponding status code returns to the audience. The gift is added to the message queue to notify the host and the other audiences that someone has sent a gift.
The demo uses Next.js to demonstrate how to call the ZEGOCLOUD Server API, you can download the source code for reference.
3. Notify the host and audience to show gift animation
ZEGOCLOUD provides various signaling channels for sending messages. You can choose a signaling channel suitable for you to send gift messages.
3.1 Unreliable message channel
The custom command provided by ZEGOCLOUD Real-Time Network is an unreliable channel. When the receiving end fails to send the message due to abnormal conditions such as network disconnection, this channel does not provide a retransmission mechanism. Therefore, there is a small probability that it won’t receive the notification.
You can send gift messages to all staff by calling the SendCustomCommand API.
The rate limit of this interface is 100 requests/second/appID. It is recommended that you send multiple gifts in one message. For example:
{
"FromUserId": "serverID",
"RoomId": "room888",
"MessageContent": [
{
"user_id": "user987",
"user_name": "James",
"gift_type": 1001,
"gift_cout": 2,
"timestamp": 1670814533,
},
...
],
}
3.2 reliable message channel
If you need to ensure that the host receives the gift message, you can call SendRoomMessage API, which needs to open the in-app chat Service.
The rate limit of this API is 10 requests/second/room, and combining multiple gifts into one message to send is recommended. For example:
{
"FromUserId": "serverID",
"RoomId": "room888",
"MessageType": 1,
"Priority": 1,
"MessageBody": {
"Message": [
{
{
"user_id": "user987",
"user_name": "James",
"gift_type": 1001,
"gift_cout": 2,
"timestamp": 1670814533,
},
...
],
"ExtendedData":"",
}
}
4. Listen to gift messages and display gift animations
Finally, you only need to monitor the gift message on the client, and when there is a new gift message, display the gift animation. Different roles need to listen to various gift message callbacks.
4.1 Gift giver
The gift giver will judge whether the gift is sent successfully according to the request status of the gift-sending API. When the status code of success is returned, it displays the gift animation.
Future<void> _sendGift() async {
late http.Response response;
try {
response = await http.post(
Uri.parse('https://zego-example-server-nextjs.vercel.app/api/send_gift'),
headers: {'Content-Type': 'application/json'},
body: json.encode({
'app_id': yourAppID,
'server_secret': yourServerSecret,
'room_id': widget.roomID,
'user_id': localUserID,
'user_name': 'user_$localUserID',
'gift_type': 1001,
'gift_count': 1,
'timestamp': DateTime.now().millisecondsSinceEpoch,
}),
);
if (response.statusCode == 200) {
// When the gift giver calls the gift interface successfully,
// the gift animation can start to be displayed
GiftWidget.show(context, "assets/sports-car.svga");
}
} on Exception catch (error) {
debugPrint("[ERROR], store fcm token exception, ${error.toString()}");
}
}
4.2 Host and non-gift-giving audiences
unreliable signaling channel
Suppose the server uses an unreliable signaling channel to broadcast messages. In that case, it needs to monitor the notification callback method onInRoomCommandReceived
of unreliable messages to determine whether someone has sent a gift. When a new gift notification is received, it displays the gift effect.
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((timeStamp) {
subscriptions..add(
ZegoUIKit().getInRoomCommandReceivedStream().listen(
onInRoomCommandReceived));
});
}
@override
void dispose() {
super.dispose();
for (var subscription in subscriptions) {
subscription?.cancel();
}
}
// if you use unreliable message channel, you need subscription this method.
void onInRoomCommandReceived(ZegoInRoomCommandReceivedData commandData) {
debugPrint("onInRoomCommandReceived, fromUser:${commandData.fromUser}, command:${commandData.command}");
// You can display different animations according to gift-type
if (commandData.fromUser.id != localUserID) {
GiftWidget.show(context, "assets/sports-car.svga");
}
}
Reliable signaling channel
Suppose the server uses a reliable signaling channel to broadcast messages. In that case, it needs to listen to the notification callback method onInRoomTextMessageReceived
of reliable messages, and when a new notification is received, it displays the gift effect.
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((timeStamp) {
subscriptions..add(
ZegoUIKit().getSignalingPlugin().getInRoomTextMessageReceivedEventStream().listen(
onInRoomTextMessageReceived));
});
}
@override
void dispose() {
super.dispose();
for (var subscription in subscriptions) {
subscription?.cancel();
}
}
// if you use reliable message channel, you need subscription this method.
void onInRoomTextMessageReceived(List<ZegoSignalingInRoomTextMessage> messages) {
debugPrint("onInRoomTextMessageStream:$messages");
// You can display different animations according to gift-type
var message = messages.first;
if (message.senderUserID != localUserID) {
GiftWidget.show(context, "assets/sports-car.svga");
}
}
Run a demo
You can download theĀ sample code for this article from here.
Conclusion
In conclusion, adding virtual gifts to your live streaming app can significantly enhance the viewer experience and open up new revenue streams. By carefully integrating this feature, you ensure that your platform is more engaging and supportive for creators, fostering a vibrant community where viewers can express their appreciation in a fun and interactive way. Implement these steps thoughtfully to maximize the benefits of virtual gifts and elevate your live streaming service to the next level.
FAQ about Virtual Gifts
Q1: What are virtual gifts in live streaming?
Virtual gifts are digital items that viewers can purchase and send to streamers during a live broadcast. These gifts can take many forms, such as emojis, stickers, or other unique icons, and often contribute to the streamer’s income.
Q2: How do virtual gifts work in live streaming platforms?
On most live-streaming platforms, viewers can buy virtual gifts with real money or in-app currency. Once purchased, these gifts can be sent to streamers during a live broadcast as a show of support or appreciation.
Q3: Why are virtual gifts important in live streaming?
Virtual gifts serve as a revenue source for streamers and the platform. They also enhance viewer engagement by allowing viewers to interact with the streamer and tangibly show their support.
Q4: Can virtual gifts be converted into real money?
Yes, on many live streaming platforms, streamers can convert the virtual gifts they receive into real money, although the platform may take a percentage as commission. The specific process and conversion rate can vary by platform.
Read more:
Letās Build APP Together
Start building with real-time video, voice & chat SDK for apps today!