Have you ever dreamed of creating your own Android app? Building an app for Android devices is more achievable than you might think, especially with the right tools and guidance. In this concise article, we will walk you through the step-by-step process of developing an Android app from scratch. With the assistance of free software and basic coding skills, you’ll be able to bring your app concept to life in no time. So, let’s delve into the details and explore how to create an Android app!
What is Android App Development?
Android app development involves creating applications for devices that run the Android OS, which is the most widely used mobile operating system globally. Initially, developers plan and design the app’s functionalities and user interface, ensuring it meets user needs effectively.
Furthermore, setting up the development environment is crucial, typically involving Android Studio, which provides tools for coding, debugging, and testing. Subsequently, developers write code using languages like Java or Kotlin and leverage Android SDK and other libraries to build and enhance the app’s performance.
Moreover, designing a user-friendly interface is essential, using XML and Material Design principles to ensure attractiveness and intuitiveness. Afterward, testing the app on emulators and real devices helps identify any issues, ensuring reliability before launch.
Finally, once refined and tested, the app is submitted to the Google Play Store, making it available to users worldwide. This process requires a blend of technical skills and creativity to develop successful Android apps that resonate with users.
What Programming Language is Used for Android Apps?
Making apps for Android phones usually involves using a language called Java. Java is known for being simple, easy to take anywhere, and safe to use. It is the main programming language used to develop Android apps, and many of these apps are made with it.
There’s also Kotlin, which is becoming popular for making Android apps. Kotlin is designed to be simpler and more direct than Java. The best part is, that you can use Kotlin with Java in the same project.
While other programming languages such as C/C++, Python, and JavaScript can be used to develop Android apps, most people prefer using Java or Kotlin as they are considered the most suitable options.
If you’re thinking of trying to make Android apps, starting with Java might be a good idea. It has a big group of users and lots of resources to help you learn.
You may also like: What is the Difference Between C and C++?
How to Build an Android App
Making an Android app involves several key steps, from setting up your development environment to designing your app and coding its functionality. Here’s a straightforward guide to help you get started with Android app development:
1. Set Up Your Development Environment
First, you need to prepare your development environment. Download and install Android Studio, the official IDE for Android development. It includes the Android SDK, essential libraries, and a suite of developer tools to help you build Android apps. After installation, launch Android Studio and follow the setup wizard to install the Android SDK and any other required components. This setup is crucial as it provides the tools and environment needed to develop, test, and debug your apps effectively.
2. Start a New Project
Once your environment is ready, start a new project in Android Studio:
- Open Android Studio and click on “Start a new Android Studio project”.
- Choose a project template. For beginners, “Empty Activity” is recommended as it provides a basic starting point.
- Enter your application name, and choose your project’s save location.
- Specify your app’s package name, which is used as a unique identifier for your app.
- Select the programming language you prefer (Java or Kotlin).
- Set the minimum SDK; for broader compatibility, choose an earlier version, but for newer features, select a newer version.
3. Design the User Interface
Designing your app’s interface is done using XML in Android Studio’s layout editor:
- Navigate to the
res -> layout
folder and openactivity_main.xml
. - Use the layout editor to drag and drop UI components like buttons, text views, and edit texts onto your screen.
- Customize properties like size, margins, and paddings in the right-hand properties panel to refine your layout.
- For more advanced designs, you can directly edit the XML code to define your UI elements and layouts.
4. Add Functionality Using Code
With your UI in place, it’s time to add functionality to your app by editing the Java or Kotlin code files:
- In the
java
directory of your project, find and openMainActivity
. - Inside
MainActivity
, write the logic that defines your app’s behavior. For example, if you have a button in your UI, you can add code to determine what happens when the user clicks the button:
Button myButton = findViewById(R.id.my_button);
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Code to execute when the button is clicked
}
});
5. Run and Test Your App
Finally, test your application to ensure it works as expected:
- Run the App: Click the ‘Run’ button in Android Studio. This compiles your application and prompts you to select a device.
- Select a Device: You can test your app using a real Android device connected to your computer or use an Android Virtual Device (AVD). If you haven’t set up an AVD yet, you can create one through the AVD Manager in Android Studio.
- Observe and Debug: As your app runs, use Logcat and the debugger in Android Studio to monitor its behavior and troubleshoot any issues.
By following these steps, you can develop and refine your Android application from concept to completion, using Android Studio and the powerful tools it provides.
How Much It Will Cost to Create an Android App
The cost to make an Android app can vary widely based on several key factors, including the complexity of the app, the level of design detail, and the experience level of the developers involved. Here’s a breakdown of the main factors that influence the overall cost:
1. Complexity and Features
- Simple Apps: These might include basic functionality without back-end data storage, simple UI components, and limited user interaction. Development costs can range from $5,000 to $20,000.
- Moderate Complexity Apps: These could include integrated APIs, moderate UI/UX design, and real-time features like messaging, or database management. Costs for these apps typically range from $20,000 to $50,000.
- Complex Apps: Featuring advanced functionalities like custom animations, third-party integrations (like payment gateways), real-time capabilities, complex back-end with multiple user roles, and sophisticated UI/UX designs, these can cost $50,000 to $100,000 or more.
2. Design and User Experience
- The more intricate and customized the user interface and user experience design, the higher the cost. Professional design services can add $5,000 to $20,000 or more to the development costs, depending on the app’s complexity.
3. Development Team
- In-House Team: Depending on their salaries and the length of the project, this could vary significantly.
- Freelancers: More affordable than agencies, but prices can vary widely based on their experience and location. Rates can range from $20 to $150 per hour.
- Outsourced Development Teams: Depending on the region, you might pay anywhere from $25 per hour in South Asia to $150 per hour in Western Europe or North America.
4. Maintenance and Updates
- After development, maintaining and updating the app is essential, typically costing around 15-20% of the original development cost per year.
5. Additional Costs
- Backend Server: Depending on whether you use BaaS (Backend as a Service) like Firebase or custom servers, costs can range from $25/month to $1000/month.
- App Store Fees: Google Play Store charges a $25 one-time fee and a 30% transaction fee for in-app purchases.
- Marketing and Launch: Marketing can greatly vary, but initial campaigns can start from $5,000 to over $20,000, depending on the scale.
Example Estimates:
- A simple Android app with basic functionality: $5,000 – $20,000
- A more feature-rich app with moderate complexity: $20,000 – $50,000
- A high-end app with complex features and design: $50,000 – $100,000+
Enhancing Android Apps with ZEGOCLOUD for Seamless Communication
In the world of Android app development, integrating advanced communication features can significantly enhance user engagement and functionality. ZEGOCLOUD provides developers with robust APIs that are perfect for adding real-time communication capabilities to any Android app. Whether you’re looking to include video calling, live broadcasting, or real-time messaging, ZEGOCLOUD’s SDKs make it straightforward.
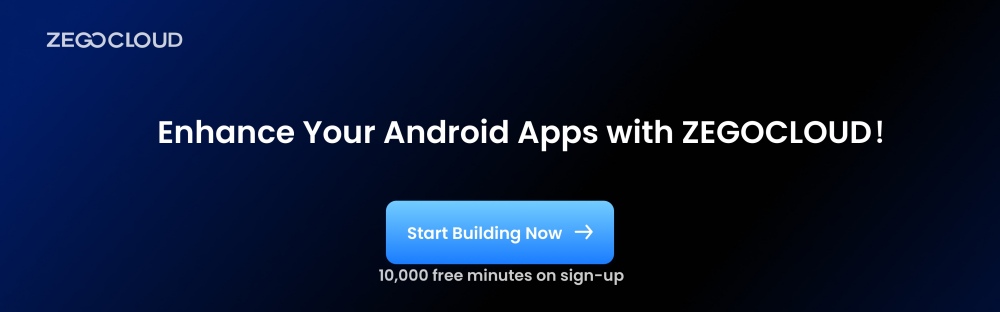
With ZEGOCLOUD UIKits just a few lines of code, you can integrate these features, which are built to deliver high performance and offer a native look and feel within your Android application. This integration not only elevates the user experience but also ensures scalability and reliability, making your app more interactive and engaging. Ideal for developers aiming to rapidly deploy advanced features, ZEGOCLOUD helps you stand out in the competitive app market by enhancing your app’s connectivity and appeal.
Conclusion
In conclusion, building an Android app is a process that combines creativity with technical skills. By setting up your development environment, designing a user-friendly interface, coding effectively, and testing thoroughly, you can create a robust Android application. Remember, the journey doesn’t end with development; regularly updating your app with new features and improvements based on user feedback is key to maintaining its relevance and popularity.
Read more:
FAQ
Q1: What are the basic requirements to start making an Android app?
To start making an Android app, you need a computer with Android Studio installed, basic knowledge of Java or Kotlin, and an understanding of Android’s app development framework.
Q2: How do I add real-time features like video calls to my Android app?
To add real-time features like video calls, integrate a reliable SDK like ZEGOCLOUD. Their SDK can help you easily implement video calling and live streaming functionalities.
Q3: What is the process for publishing my Android app on the Google Play Store?
To publish your app, create a developer account on Google Play, prepare your app with a signed APK or Android App Bundle, and submit it through the Google Play Console for review.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!