C and C++ are both popular programming languages with similar syntax but key differences. C is a simple, efficient language often used for system-level programming, while C++ builds on C by adding object-oriented features, making it suitable for larger, more complex applications. This article will highlight the difference between C and C++.
What is C?
C is a general programming language. Dennis Ritchie developed it at Bell Labs in the 1970s. Since then, it has become widely used worldwide. It is a compiled language. It converts source code into machine code. Compiled languages like C are fast, and efficient and allow low-level memory access.
Moreover, C has core concepts: functions, pointers, arrays, strings, structures, and files. Functions break up code into reusable parts. Pointers provide direct memory access to manage data. Arrays store collections of data. Strings handle text. Structures groups related data types. Files allow reading and writing data streams.
Furthermore, C lacks built-in features for some tasks. It does not have input/output, string handling, or advanced data structures like lists/trees. Instead, it uses code libraries for reusable utilities. C’s minimalist nature gives programmers more control over memory.
Features of C Language
From what we have covered so far, you can tell that the C language is compelling. We are yet to scratch the surface. The following are some of its impressive features:
1. Portability
It is one of the most preferred languages for low-level programming. This is because of the ease at which you can modify C programs to run on different architectures. For instance, a program written for the Intel CPUs for the Windows Operating System can be made quickly to run on the Mac with AMD processors. This is very useful, as it can save a lot of human and capital resources.
2. Rich Library Support
C has a feature-rich standard library for many operations. It has a library for input and output operation, file handling, mathematical operations, web, etc. The availability of many libraries in C makes it easy to get things done.
3. Easy to Learn
C syntax may look challenging, but you’re good to go once you know the fundamentals. Moreover, C’s keywords and data structures are relatively smaller than the ones we have today. This is because it was designed to be compact and efficient.
4. Fast and Efficient
C programs run directly in the language the computer understands native (i.e., machine language or binary).
What is C++?
It is a powerful and mature programming language that is widely used by developers.
Bjarne Stroustrup created C++ in the 1980s. He saw limitations in the C language and wanted to create a new language that was more powerful and flexible. C++ was designed to be a high-level language that was easy to use, while still providing the performance and control of a low-level language.
The object-oriented nature of C++ is one of its core powers. Object-oriented programming (OOP) is a way of organizing code into self-contained units called objects. Objects can contain data and code, and they can interact with each other. OOP makes code more modular and reusable, which makes it easier to maintain and extend.
If you are already familiar with the C programming language, then learning C++ will be relatively easy. Many of the keywords and concepts in C are also used in C++. However, there are also some important differences between the two languages, so it is important to learn about them.
Today, C++ is used in a wide variety of C++ projects. It is a popular choice for game development, system programming, and embedded systems. C++ is also used in a number of other areas, such as web development and finance.
Features of C++
Some of the key features of C++ include the following:
1. Object-oriented programming (OOP)
Firstly, C++ is a fully object-oriented language, which means that it allows programmers to create and manipulate objects. Objects are self-contained units of data and code that can be reused throughout a program. OOP is a powerful way to organize and structure code, and it can make programs more modular, reusable, and maintainable.
2. High performance
Secondly, C++ is a compiled language, which means that it is converted into machine code before it is executed. This makes C++ programs very fast and efficient. C++ is often used for high-performance applications, such as games, graphics, and scientific computing.
3. Memory management
Furthermore, C++ gives programmers direct control over memory management. This can be a powerful tool, but it can also be a source of errors. C++ programmers must be careful to manage memory correctly to avoid memory leaks and other problems.
4. Portability
Lastly, C++ is a portable language, which means that it can be compiled and run on a variety of platforms. Hence, this makes C++ a good choice for developing cross-platform applications.
In addition to these four features, C++ also has a number of other features, such as support for templates, namespaces, and exceptions. These features make C++ a powerful and versatile language that can be used for a wide variety of applications.
Similarities between C and C++
So far, you can see that they are two of the most powerful programming languages in the world. They are both powerful, efficient, and portable, and they are used to create a wide variety of software applications. There are many similarities between them:
- Syntax: Both C and C++ share a similar syntax, which makes transitioning from one to the other relatively easy for developers. They both use curly braces
{}
to define code blocks and semicolons;
to terminate statements. - Compiled Languages: Both C and C++ are compiled languages, meaning that the source code is translated into machine code by a compiler before execution.
- Low-level Programming: Both languages allow low-level memory manipulation and direct access to hardware resources, making them ideal for system-level programming and performance-critical applications.
- Efficiency: C and C++ are known for their high performance and efficiency, offering fine control over system resources like memory and processing power.
- Data Structures: Both C and C++ support basic data structures such as arrays, pointers, and structs, allowing efficient handling of data.
- Standard Libraries: Both languages come with their own standard libraries (e.g., C’s
stdio.h
,stdlib.h
and C++’siostream
,vector
) that provide functions and utilities for common programming tasks. - Preprocessor Directives: Both C and C++ use preprocessor directives like
#include
and#define
to manage file inclusion and macro expansion before code compilation. - Portability: Both C and C++ are highly portable and can run on virtually any platform, from embedded systems to high-performance computers.
Differences between C and C++
C and C++ are both powerful programming languages with a shared syntax, but they differ significantly in their features and capabilities. Below is a comparison that highlights the key differences between the two languages.
Feature | C | C++ |
---|---|---|
Programming Paradigm | Procedural Programming | Multi-paradigm (Procedural, Object-Oriented) |
Object-Oriented Support | No | Yes (Classes, Objects, Inheritance, Polymorphism, Encapsulation) |
Data Abstraction | No built-in support for abstraction | Supports data abstraction through classes and objects |
Memory Management | Manual memory management (malloc, free) | Manual memory management (new, delete) + RAII (Resource Acquisition Is Initialization) |
Function Overloading | Not supported | Supported |
Operator Overloading | Not supported | Supported |
Namespaces | No | Yes |
Exception Handling | Not supported | Supported (try, catch, throw) |
Standard Library | Standard library (stdio.h, stdlib.h, etc.) | Larger Standard Library (includes STL: Standard Template Library) |
Compatibility | Compatible with most low-level systems | Compatible with most systems, but may be more complex due to OOP features |
Inheritance | Not supported | Supported |
Access Modifiers | No | Yes (public, private, protected) |
Multithreading | Manual management (via libraries) | Native support via libraries and tools (e.g., threads in C++11) |
Code Complexity | Simpler and smaller, typically | More complex due to object-oriented features |
Use Cases | System-level programming, embedded systems, OS development | Application software, game development, complex systems |
Example Program of C and C++
As we delve into example programs, we’ll uncover these distinctions, offering clarity on their unique features.
Let’s start with the C version:
// Simple Calculator Program in C
#include <stdio.h>
int main() {
char operator;
double num1, num2, result;
// Get input from the user
printf("Enter an operator (+, -, *, /): ");
scanf("%c", &operator);
printf("Enter two numbers: ");
scanf("%lf %lf", &num1, &num2);
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0)
result = num1 / num2;
else {
printf("Error: Division by zero\n");
return 1;
}
break;
default:
printf("Error: Invalid operator\n");
return 1;
}
// Display the result
printf("Result: %.2lf\n", result);
return 0;
}
C++ version:
// Simple Calculator Program in C++
#include <iostream>
using namespace std;
int main() {
char op;
double num1, num2, result;
// Get input from the user
cout << "Enter an operator (+, -, *, /): ";
cin >> op;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch (op) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0)
result = num1 / num2;
else {
cout << "Error: Division by zero" << endl;
return 1;
}
break;
default:
cout << "Error: Invalid operator" << endl;
return 1;
}
// Display the result
cout << "Result: " << result << endl;
return 0;
}
How ZEGOCLOUD Leverages C/C++ for Real-Time Communication
ZEGOCLOUD is a powerful platform that provides real-time communication (RTC) services such as video calling, live streaming, and messaging. While developers typically interact with ZEGOCLOUD using higher-level languages like JavaScript, Swift, or Kotlin, the platform’s core functionality is built on C and C++. These languages are essential for WebRTC (Web Real-Time Communication), which powers ZEGOCLOUD’s ability to deliver low-latency, high-quality media streaming and real-time interactions.
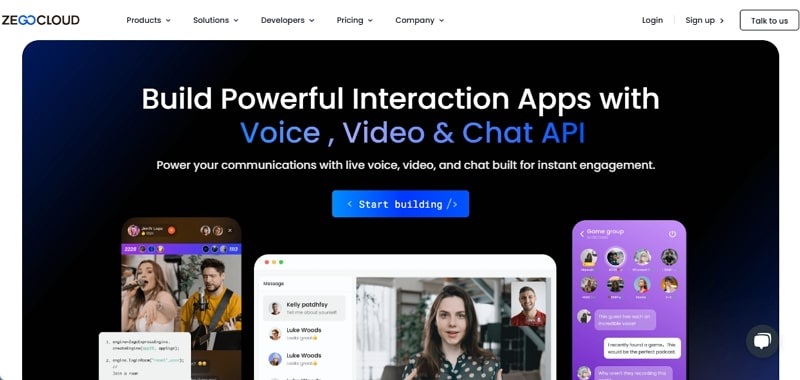
C/C++ play a crucial role in performance-critical tasks such as video encoding and decoding, network data transmission, and audio processing. By utilizing these languages, ZEGOCLOUD ensures that its real-time communication services can handle complex tasks efficiently while maintaining smooth and reliable performance. Whether it’s for mobile apps or desktop applications, the integration of C/C++ into ZEGOCLOUD’s SDKs helps deliver seamless, high-performance communication experiences across platforms.
Conclusion
C++ and C are both powerful programming languages, but they have different strengths and weaknesses. Ultimately, determining the most suitable language for you entails immersing yourself in both options and discerning your personal preference through firsthand experience.
If you’re looking for a way to build video call applications in C++, ZEGOCLOUD’s SDK is a great option. It’s easy to use, reliable, and cross-platform. So, what are you waiting for? Sign up and start building your video call application today!
Read more:
- Swift vs. Objective-C: How to Choose for iOS App Development?
- What is Java: The Complete Beginner’s Guide
FAQ
Q1: What is the main difference between C and C++?
The main difference is that C is a procedural programming language, while C++ supports both procedural and object-oriented programming. C++ introduces features like classes, inheritance, and polymorphism, allowing for more complex and reusable code.
Q2: Which is better to learn, C or C++?
It depends on your goals. C is ideal for low-level system programming, while C++ is better for larger, object-oriented projects and offers more advanced features. If you plan to work on complex applications, C++ is a better choice.
Q3: Is C harder than C++?
C is generally simpler because it lacks object-oriented features. However, C++ can be more challenging due to its additional complexity, but it offers more powerful tools for building scalable applications.
Q4: Can I learn C++ without learning C?
Yes, you can learn C++ directly. While C++ builds on C, it introduces object-oriented programming, so it’s not necessary to learn C first. However, learning C can help you better understand low-level programming concepts in C++.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!