Slack is a real-time team communication app with chat, file sharing, and project management features. React Native is a JavaScript framework for building native-looking mobile apps for iOS and Android. This guide will show you how to build a fully functional Slack clone app using React Native with ZEGOCLOUD. Let’s get started!
Is It Possible to Create a Successful Chat App?
Yes. The demand for effective communication platforms is skyrocketing in today’s interconnected world.
However, creating a successful chat app is not easy. It requires careful planning, innovative thinking, and a deep understanding of users’ wants. Many factors come into play from intuitive user interfaces to robust backend infrastructure.
Must-have Features of a Good Team Messaging App and Chat App
To facilitate seamless communication, a good team messaging and chat apps must possess certain essential features. Let’s explore these must-have features that contribute to the success of such applications.
1. Real-Time Messaging
A team messaging app should provide real-time messaging capabilities, allowing team members to exchange messages instantly. This feature ensures swift and efficient communication, fostering quick decision-making and collaboration.
2. Group Chats and Channels
The app should enable the creation of group chats and channels where team members can join specific conversations based on projects, departments, or interests. Group chat promotes organized communication and helps in keeping discussions focused.
3. File Sharing
An efficient team messaging app should facilitate seamless file sharing. Team members should be able to share documents, images, videos, and other relevant files within the app, eliminating the need for separate file-sharing platforms and ensuring all project-related assets are easily accessible.
4. Search Functionality
A robust search functionality becomes indispensable with the abundance of messages and files exchanged within a team messaging app. This feature lets users quickly search and locate specific messages, files, or conversations, saving time and enhancing productivity.
5. Integrations
A good team messaging app should offer integrations with other essential tools and platforms to streamline workflows and enhance productivity. Integration with project management tools, calendar apps, and document collaboration platforms allows seamless information sharing and enhances team efficiency.
6. Notifications and Alerts
Timely notifications and alerts keep team members updated on important messages and activities. The app should offer customizable notification settings, allowing users to prioritize and manage their alerts effectively.
7. Security and Privacy
A reliable encrypted team messaging app must prioritize data security and user privacy. End-to-end encryption, secure authentication methods, and user permission controls are crucial features to protect sensitive team information.
8. Cross-Platform Compatibility
To accommodate the diverse technology preferences of team members, a good team messaging app should be accessible across multiple platforms. Whether it’s desktop, web, or mobile devices, seamless cross-platform compatibility ensures team members can communicate and collaborate regardless of their chosen devices.
How Do I Create a Slack Clone?
If you want to create your Slack clone, ZEGOCLOUD’s In-app Chat SDK is a game-changer. With its powerful features, you can build a robust messaging platform similar to Slack. The SDK offers real-time messaging, group chats, file sharing, and more, ensuring a seamless user experience.
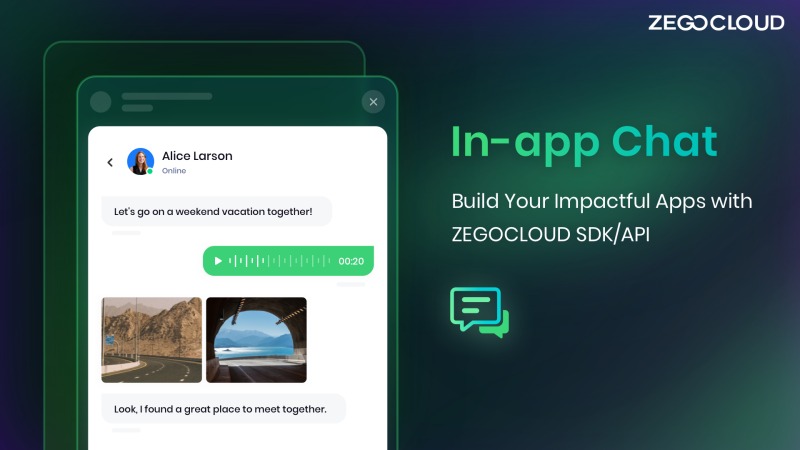
ZEGOCLOUD’s In-app Chat SDK simplifies development by providing ready-made components and scalable infrastructure. With their expertise, you can efficiently bring your Slack clone vision to life. Empower your users with a feature-rich chat experience using ZEGOCLOUD’s In-app Chat SDK.
Preparation
- A ZEGOCLOUD developer account – Sign up
- Create and activate In-app chat in the admin console
- Have NodeJS installed
- Basic understanding of web development
Once the requirements are met, you can proceed to the next steps.
Steps by Steps to Integrate the SDK for Slack Clone App
Optional: Create a new project
- Create a new React Native project called
Zego-zim-example
using the built-in command line utility. You can use theNode.js
npx
command to run the utility without installing it.
npx react-native init zego-zim-example
- To compile and run the project on iOS, open the project in Xcode and select Product > Run. You can as well use the command below:
yarn react-native run-ios
- To compile and run the project on Android, run the following command in your terminal:
yarn react-native run-android
Import the SDK
To import the In-app Chat SDK using NPM, follow these steps:
- Open a terminal window and navigate to the directory where you want to install the SDK. Run the following command:
npm install @zegocloud/zimkit-react-native
- Import the SDK into your project.
import { ZIMKiti18n } from '@zegocloud/zimkit-react-native';
Create a ZIM SDK instance
To initiate a ZIM instance, symbolizing client login, both clients A and B must utilize the create method. By providing the previously acquired AppID
they can establish their individual ZIM SDK instances from the initial prerequisites, enabling message exchange between them.
/*
By default, the ZIM SDK employs the AppSign authentication mode. To modify the authentication mode, either reach out to Technical Support for SDK 2.3.0 or later or make the change independently for SDK 2.3.3 or later.
To establish a ZIM SDK object, employ a static synchronized method and provide the AppID and AppSign. The create method generates a ZIM instance solely upon its initial invocation, returning null for subsequent usages.
*/
ZIM.create({ appID: 0, appSign: '' });
// To avoid hot updates that return null multiple times, get a single instance of the ZIM SDK using the getInstance method.
var zim = ZIM.getInstance();
Set event callbacks
To customize event callbacks, such as receiving SDK error notifications or message-related notifications, call the on
method before the client user logs in.
// Receive error codes by setting up a callback for the error event.
zim.on('error', function (zim, errorInfo) {
console.log('error', errorInfo.code, errorInfo.message);
});
// Listen for connection status changes by setting up a callback for the event.
zim.on('connectionStateChanged', function (zim, { state, event, extendedData }) {
console.log('connectionStateChanged', state, event, extendedData);
});
// Receive one-to-one messages by setting up a callback for the message event.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log in to the ZIM SDK
To enable message exchange and token renewal, clients A and B must log in to the ZIM SDK. They can achieve this by following these steps:
- Utilize the
ZIMUserInfo
method to generate a user object. - Utilize the
login
the method with their respective user information and the Token obtained from the previous Prerequisites steps.
// User IDs should be limited to 32 characters and can consist of letters, numbers, and the special characters: ~!@#$%^&*()_+-=`;',.<>/\.
// userName must be limited to 1-64 characters.
var userInfo = { userID: 'xxxx', userName: 'xxxx' };
/*
During the login process:
For Token-based authentication, provide the Token obtained from the [Guides - Authentication] document.
For AppSign authentication (default mode in SDK 2.3.0), leave the Token field empty.
*/
zim.login(userInfo, '')
.then(function () {
// Login successful.
})
.catch(function (err) {
// Login failed.
});
Send messages
Client A calls sendMessage
with B’s user ID, content, and type to send the message. sendMessage returns ZIMMessageSentResult
and calls onMessageAttached
with a ZIMMessage
. Use onMessageAttached
to get the ID or local ID before sending.
// To send a 1V1 message, set the ZIMConversationType to Peer.
var toUserID = 'xxxx1';
var config = {
priority: 1 // Set the message priority to one of three levels: low, medium, or high. Low is the default.
};
var type = 0; // The session type can be one-on-one (0), chat room (1), or group chat (2).
var notification = {
onMessageAttached: function(message) {
// todo: Loading
}
};
// Send a 1V1 text message.
var messageTextObj = { type: 1, message: 'Text message content' };
zim.sendMessage(messageTextObj, toUserID, type, config, notification)
.then(function ({ message }) {
// Successful messages.
})
.catch(function (err) {
// Failed messages.
});
Receive messages
When client B logs in, client A’s message will be delivered to the callback function that was previously set in the receivePeerMessage
method.
// Register a callback to receive one-to-one messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log out
To initiate the client’s logout process, you can use the logout
method.
zim.logout();
Destroy the ZIM SDK instance
To dismantle the ZIM SDK instance, invoke the destroy
method.
zim.destroy();
Run a Demo
To explore the capabilities of ZEGOCLOUD’s In-app Chat SDK, you can try our React Native sample app.
Conclusion
Building a Slack clone app with React Native is a great way to learn how to use React Native and build cross-platform mobile apps. By following the instructions in this guide, you can build a fully functional Slack clone app that you can use to communicate and collaborate with your team.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!