Live chat is a powerful tool for enhancing user engagement and support in mobile apps. By allowing real-time communication, you can offer better customer service, drive user interaction, and improve overall app experience. In this article, we’ll explore the key steps to integrate a super live chat feature with ZEGOCLOUD in your app, helping you deliver instant and personalized support to your users.
What is Live Chat?
Live chat is a real-time messaging tool integrated into websites and mobile apps, allowing users to instantly communicate with customer service teams, sales representatives, or support agents. It enhances user experience by providing immediate answers to questions, resolving issues quickly, and building trust through direct interaction. Live chat is widely used in e-commerce, SaaS, and service-based industries to boost customer satisfaction and increase conversion rates.
Why Super Live Chat is Important?
Super live chat is a service that allows users to communicate with each other in real-time over the Internet. It is an important function of popular apps on the market, such as WhatsApp and Facebook Messenger.
In recent years, driven by the rapid development of mobile technology and the growing trend for social features in the app market, it has been widely used for communication on social media platforms, and in scenarios as diverse as e-commerce, live streaming, customer service, etc.
Research shows that more than 60% of apps on the market have the super live chat feature, through which users can chat with each other in real-time, thus improving app engagement and user experience. For app developers, Super live chat implementation has become a must-have skill.
With more than 10 years of experience in developing super live chat apps, the author shares three options for super live chat implementation and hopes this article could be of help to developers.
Essential Features of Live Chat for Apps
When building a modern app, integrating live chat functionality can significantly enhance user engagement and retention. Here’s a concise list of essential features of live chat for apps:
- Real-Time Messaging – Instant, two-way communication without delays.
- Typing Indicators – Show when the other user is typing, enhancing engagement.
- Read Receipts – Let users know when messages are seen.
- User Presence Status – Display online/offline status to manage expectations.
- Message History – Store and access past conversations for context.
- Push Notifications – Alert users of new messages even when offline.
- Media Sharing – Support sending images, videos, and files.
- Chat Moderation Tools – Block/report users, filter content, and manage abuse.
- Group Chat Support – Enable multi-user conversations for teams or communities.
- Security & Encryption – Ensure conversations are private and data is protected.
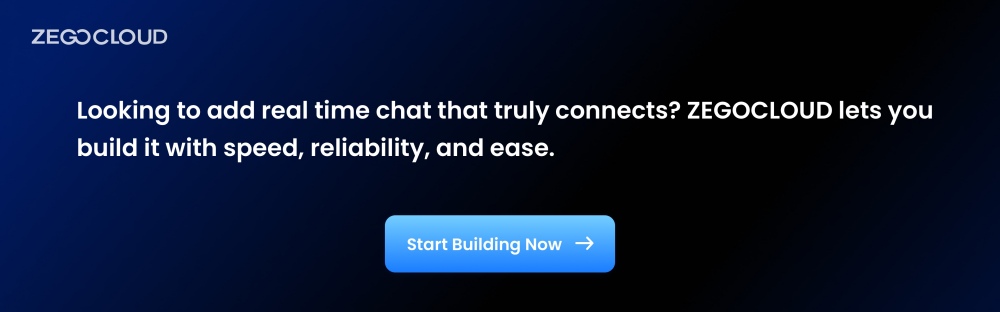
Best 10 Live Chat Apps in 2025
While there are many live chatting apps on the internet, not all are popular due to a lack of features or security concerns. Subsequently, to ensure you don’t have to go through the trouble, we have selected the best 10 live chat apps for both personal and business communications below:
- WhatsApp: A popular live chat app.
- Telegram: Secure live chat app.
- Signal: Privacy-focused chat app.
- Facebook: Social media chat app.
- Threema: Anonymous live chat app.
- LINE: Asian social chat app.
- WeChat: Chinese multifunctional chat app.
- Viber: Free messaging chat app.
- Snapchat: Creative photo chat app.
- Skype: Video and voice chat app.
1. WhatsApp
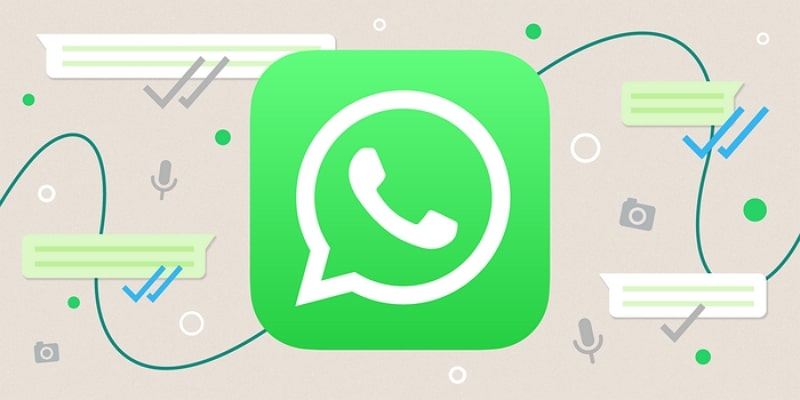
To begin with, the best free live chat app is WhatsApp, the most widely used app in the world. With over 2.25 billion users, it is far ahead of any other app. Owned by Facebook, it is currently available for free to download and use. With this app, you can text, voice, and video chat with your contacts. It also allows you to share files, locations, contacts, etc.
Furthermore, you can also use this app to connect with your customers if you run a business. There is also a WhatsApp Business for business owners, free to download and use.
2. Telegram
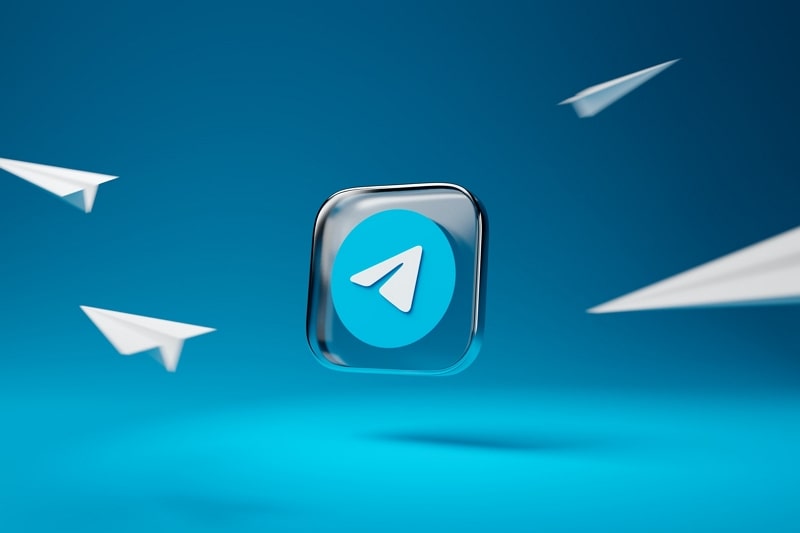
Known for providing secure and encrypted chats, Telegram is free to download and use live chat app. Although introduced in 2013, the app gained popularity in late 2020 after privacy concerns were raised over WhatsApp. It currently has over 700 million active users and is gaining popularity rapidly. This app’s secret chat feature allows users to chat with complete privacy.
Moreover, it offers secure voice and video chat services for individual and group chats. The app has an automated bulk message forwarding feature for business owners to ensure they stay connected with their base.
3. Signal
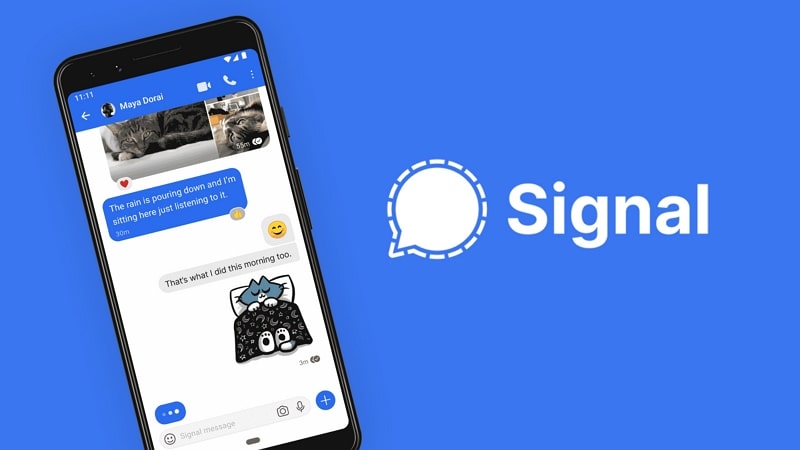
Released to be a more secure version of Telegram, Signal is an open-source and free live chat app. In Signal, the encryption of chats and media ensures data security. On the other hand, Telegram only encrypts the chats done using the secret chat feature. You won’t find a better app than Signal when it comes to privacy and data protection.
A non-profit organization owns Signal and doesn’t store your data either. Subsequently, this app has no ads, and its clean interface makes it excellent for important business discussions.
4. Facebook Messenger
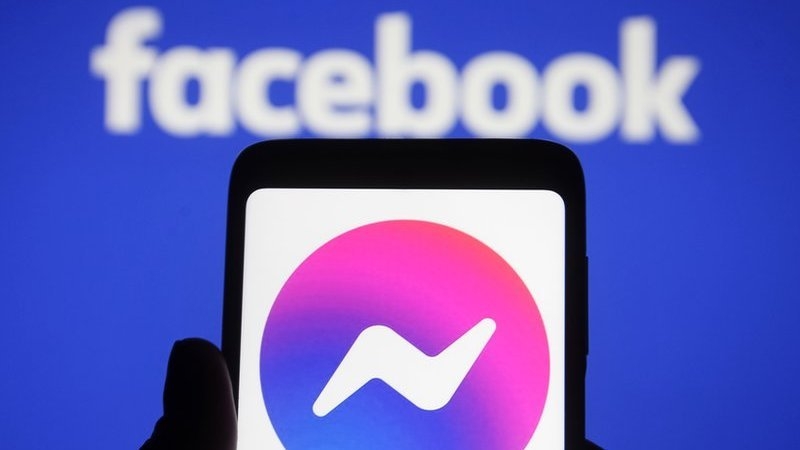
Facebook is widely popular among users worldwide, and that makes Facebook Messenger an excellent way to reach the masses. Using Facebook Messenger, you can connect to your FB friends through text, voice, and video chat. It is available as a stand-alone app for all major platforms like iOS, Android, Windows, and Mac.
The chats on this app aren’t encrypted in general, but users have the option to initiate an end-to-end encrypted chat. Since businesses widely use Facebook, it makes Facebook Messenger a perfect way to connect with customers.
5. Threema
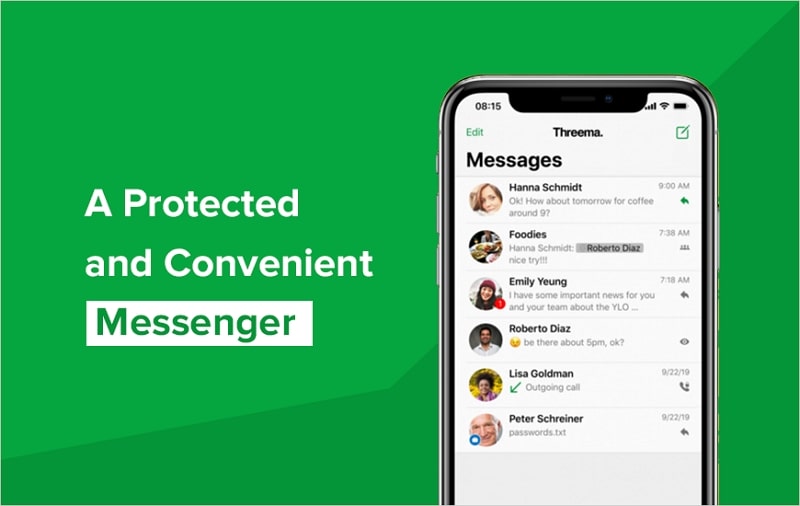
Referred to as the most private chatting app, Threema is a paid and decentralized live chat app released in 2012. Unlike all other apps, it doesn’t require your phone number, email, or other details. After buying the app, users are assigned a specific ID that sets up their Threema account. This app allows you to talk to friends using text, voice, and video chat options.
Since it is open-source, users can ensure that there is no security risk in using this app. Threema is usable after making a one-time payment of $4.99.
6. LINE
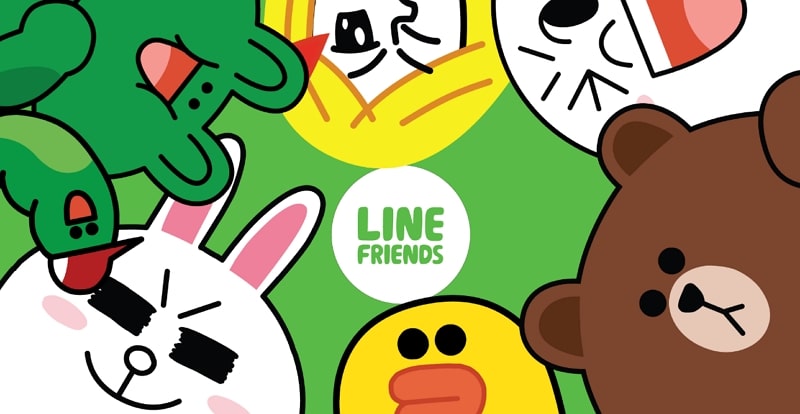
Available on Android, iOS, Windows, and Mac, LINE is a great option to connect with your acquaintances. It is widely popular in Japan, Thailand, and Indonesia and has 700 million users worldwide. LINE allows you to make voice and video chats and share other media like photos, documents, and audio.
This app also has many unique features, like LINE Games, LINE Pay, LINE Today, and LINE Taxi. It also has a wide variety of stickers to share during chats. All the communications on LINE app are end-to-end encrypted.
7. WeChat
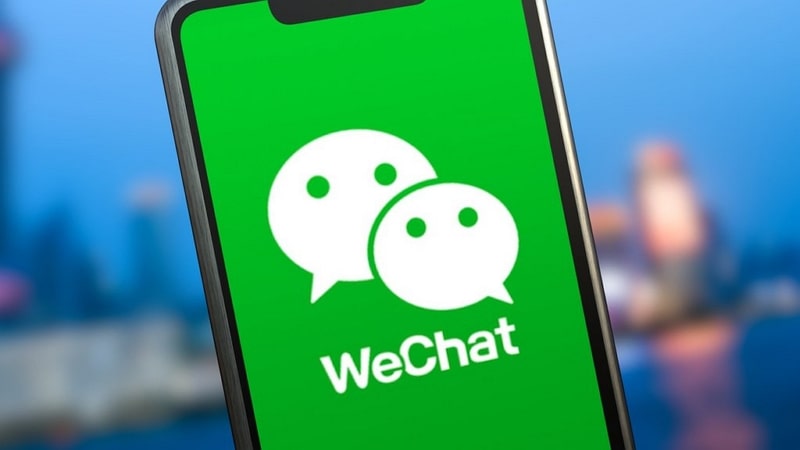
Most popular in China, WeChat is considered to be one of the most used and best live video chat apps. It has over a billion regular users, partially because most live chatting apps are banned in China. In China, it is known as the app for everything since it probably has more features than any other app on the list.
These features include text, voice, video chats, mobile payments, location sharing, and much more. If you are a business owner and want to reach out to Chinese customers, WeChat is the best choice.
8. Viber
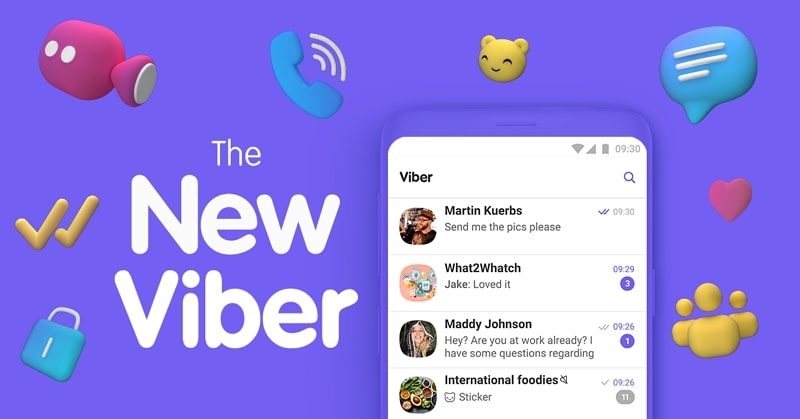
If you want to visit Eastern Europe, Viber is an excellent live chat app to use. It is widely popular in Russia, Ukraine, Bulgaria, and Greece, with over 300 million users. With this app, you can make high-quality group video calls, voice calls, and text messages. Viber has a unique feature called Viber Out which allows you to make calls to landline and mobile networks.
Viber can be very helpful for businesses with its different crowdsource features like polling and mass forwarding. It is available for free to use on all major platforms.
9. Snapchat
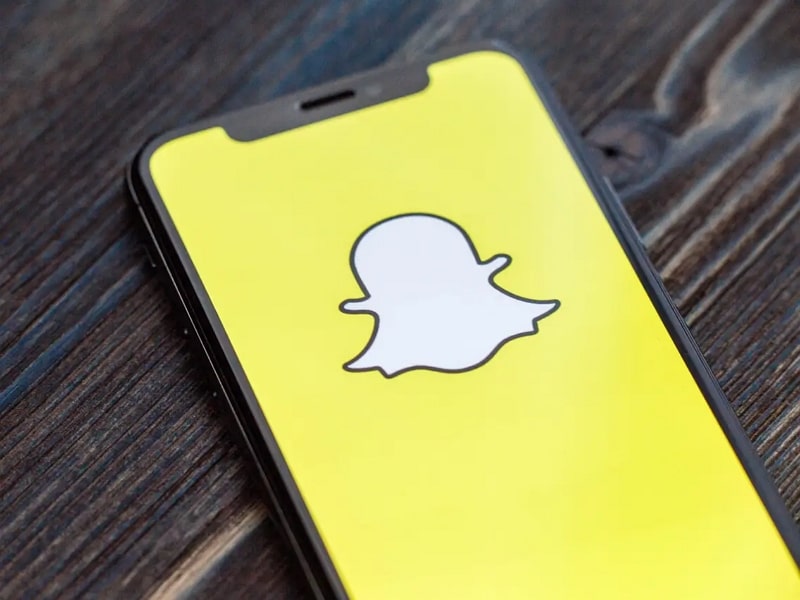
Did you know it is possible to make audio and video calls on Snapchat? While Snapchat is more famous for sharing stories and sending snaps, it is also an excellent free live chat app. In this app, users can quickly take snaps or make stories using a wide range of effects, filters, and stickers.
All the chats on Snapchat are deleted after some time, making it a secure platform. Businesses can promote themselves on Snapchat by creating their branded filters and stickers. Snapchat app is only available on Android and iOS devices.
10. Skype
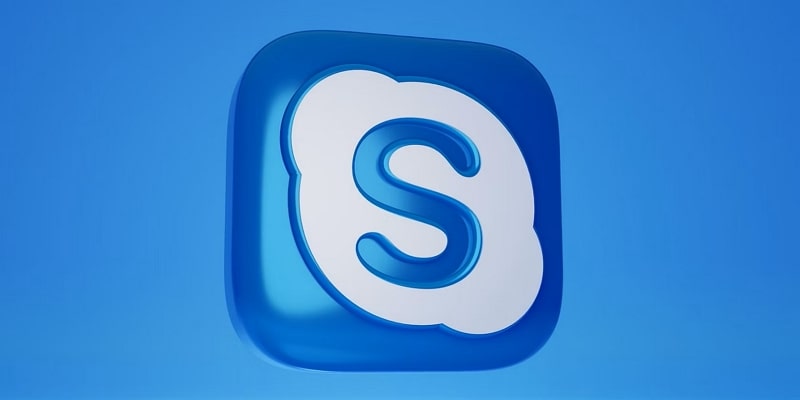
One of the oldest live chatting apps, Skype is known as the industry standard for making video and audio calls. It is widely used by businesses to arrange virtual meetings and conferences. While its general features are free to use, most unique features are premium. One such feature is the ability to make calls on landlines and mobile numbers.
You can buy an international number from Skype for marketing if you run a business. Through it, you can connect with customers and clients globally.
3 Methods to Implement Super Live Chat to Your App
There are three main ways to develop a super live chat app: using open-source code, building it in-house, or integrating a commercial SDK. The best approach depends on your project’s scale, available resources, and level of customization required. No matter which method you choose, each involves three key components: client-side development, server-side development, and operations and maintenance (O&M).
Method 1: Use open-source projects
Using an open-source project is the fastest way to implement super live chat as you can embark on a fast-track product development by drawing on the wisdom of the whole community.
What should we focus on when choosing an open-source project for super live chat? The maturity of functions and the number of active users are two important aspects to consider when choosing an open-source project. Based on my experience, I would like to share with you two good open-source projects.
1. MongooseIM
Project URL: https://github.com/esl/MongooseIM
MongooseIM is Erlang Solutions’ robust, scalable, and efficient XMPP server, aimed at large installations. Specifically designed for enterprise purposes, it is fault-tolerant and can utilize the resources of multiple clustered machines.
2. OpenIM
Project URL: https://github.com/OpenIMSDK/Open-IM-Server
OpenIM was founded and developed by senior IM architects and IM/WebRTC experts, aiming at creating service value and developing lightweight, high-availability super live chat architecture through open-source technology. With OpenIM, developers can easily build multiple IM and real-time interactive audio/video scenarios in an app.
An open-source project is an excellent option for developers who have to meet a tight deadline and without too many needs for customization to realize fast IM implementation. However, most open-source projects only offer basic functionality, and extending them often depends on the pace and direction of the community’s development. If you want to create a bespoke IM app, or if you expect a growing business, I advise you against using this method.
Teams with R&D capabilities usually prefer in-house development if they want a custom IM product. The next part will guide you through the technical difficulties and pitfalls in the process of in-house development.
Method 2: In-house development
In-house development provides complete control over the app’s design, functionality, and scalability. However, it demands significant financial investment, time, and technical expertise. Key technical challenges include:
- Message reliability and order under heavy traffic
- Handling high concurrency in real-time messaging
- Choosing between push and pull models for message storage
- Accurate unread counts and group acknowledgments
- Multi-data center deployment and sync logic
To accelerate development, enterprises can integrate existing solutions for features like file storage, security audits, and offline notifications, replacing them with in-house components when needed.
Pros: Full control, high scalability, tailored solutions.
Cons: High costs, longer development cycles, and resource-intensive maintenance.
In-house development is best suited for large enterprises with strong technical teams and a long-term vision for their product.
Method 3: Integrate a commercial SDK
Integrating a commercial SDK offers the fastest and most efficient way to implement a super live chat app. This approach reduces development complexity while allowing for feature scalability and reliable performance.
Advantages:
- Quick Deployment: Get to market faster with ready-made solutions.
- Cost-Effectiveness: Requires less financial and technical investment compared to in-house development.
- Reliability: Ensures stability and minimizes O&M efforts.
- Scalability: New features can be easily added by coordinating with the SDK provider.
Implementation Steps:
- Register and obtain a user account and credentials from the SDK provider.
- Develop a lightweight service backend for authentication.
- Integrate the SDK into your app, conduct necessary testing, and complete feature integration..
Example Workflow for Sending Messages:
Modules within the SDK handle user authentication, message routing, and data storage, ensuring seamless interaction between the client and server.
This method is ideal for small and medium-sized businesses or startups looking to launch quickly without compromising on functionality or scalability.
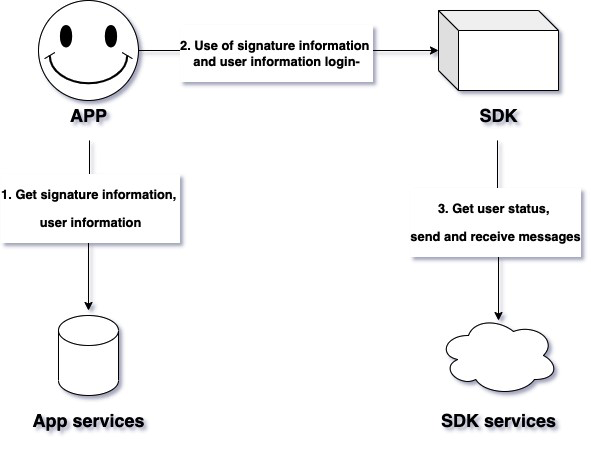
How Can ZEGOCLOUD Help for Super Live Chat Implementation
ZEGOCLOUD provides comprehensive solutions to help developers implement super live chat functionalities efficiently and effectively. With its powerful SDKs and developer resources, ZEGOCLOUD enables seamless integration of real-time messaging and live streaming capabilities into mobile and web applications.
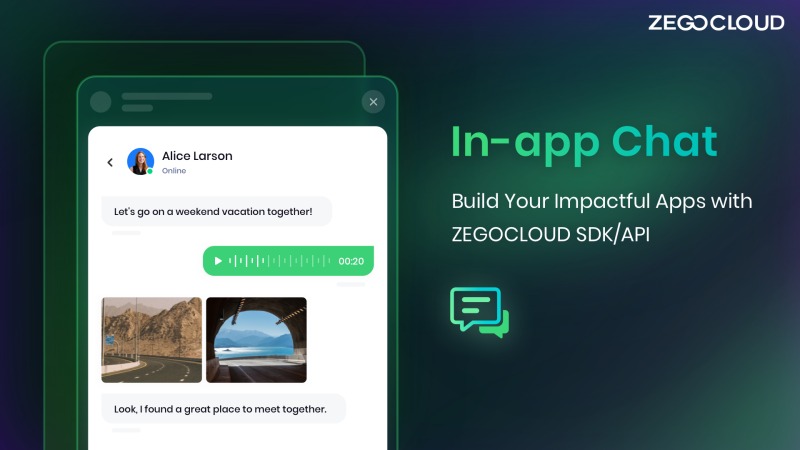
In-App Chat SDK supports key features like push notifications, read receipts, user and group management, and rich media attachments. It also ensures offline messaging for uninterrupted user experiences, making it ideal for applications that require reliable and interactive chat systems.
For apps needing live streaming capabilities, ZEGOCLOUD’s Live Streaming SDK delivers ultra-low latency, scalability for large audiences, and high reliability, ensuring stable performance even under challenging network conditions. This SDK is suitable for interactive streaming scenarios, enhancing user engagement.
ZEGOCLOUD also provides extensive developer support, including detailed documentation, demo applications, and accessible SDK downloads. These resources simplify the integration process, allowing developers to customize and deploy chat and streaming features with ease.
Additional tools like AI Effects and the Super Board further enrich the user experience by adding real-time video effects and collaborative whiteboard functionalities, making the application more engaging.
By leveraging ZEGOCLOUD’s SDKs and tools, businesses can quickly implement scalable, high-performance super live chat solutions that cater to diverse user needs while reducing development time and costs.
Final Thoughts
In conclusion, implementing a super live chat for customer support is a strategic move to enhance user satisfaction, streamline communication, and boost engagement. Whether you choose to build it using open-source code, in-house development, or integrate a commercial SDK, the right approach depends on your resources, timelines, and business goals. By focusing on seamless client-side interfaces, robust server-side functionality, and reliable operations and maintenance, you can deliver a live chat experience that meets user expectations and supports your organization’s growth. Start today, and transform your customer support into a real-time, personalized solution that keeps your customers coming back.
Read More
FAQ
Q1: How to set up super chats?
To set up super chats, you typically need to enable monetization features on platforms like YouTube or integrate a third-party live chat SDK that supports tipping or highlighted messages. If you’re building your own app, this involves adding payment integration and UI logic for paid message display.
Q2: How do you implement LiveChat?
LiveChat can be implemented by embedding a prebuilt widget or integrating a live chat SDK into your app or website. Most commercial providers offer simple JavaScript snippets or SDKs for mobile platforms, along with APIs for customizing the chat experience.
Q3: What is super LiveChat?
Super LiveChat refers to an advanced live chat experience that goes beyond basic text messaging. It often includes features like real-time video/audio, interactive UI, file sharing, emojis, moderation tools, and monetization options like tips or virtual gifts.
Q4: How do you implement chat service?
You can implement a chat service using one of three approaches: building it from scratch, using an open-source solution, or integrating a commercial chat SDK like ZEGOCLOUD. The core components include the client-side UI, a messaging server, database storage, and real-time data transmission.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!