Building a JavaScript chat app is a great way to learn the basics of web development and communication. In this tutorial, you’ll learn how to create a simple chat app with ZEGOCLOUD that allows users to send and receive messages. You’ll also learn how to use JavaScript to add real-time functionality to your app.
How to Make a Chat App using JavaScript
How to build a chat app with JavaScript? ZEGOCLOUD’s In-app Chat SDK can further streamline and simplify the development process for the Javascript chat app. This chat SDK provides a variety of features, including real-time messaging, rich media sharing, and user presence management.
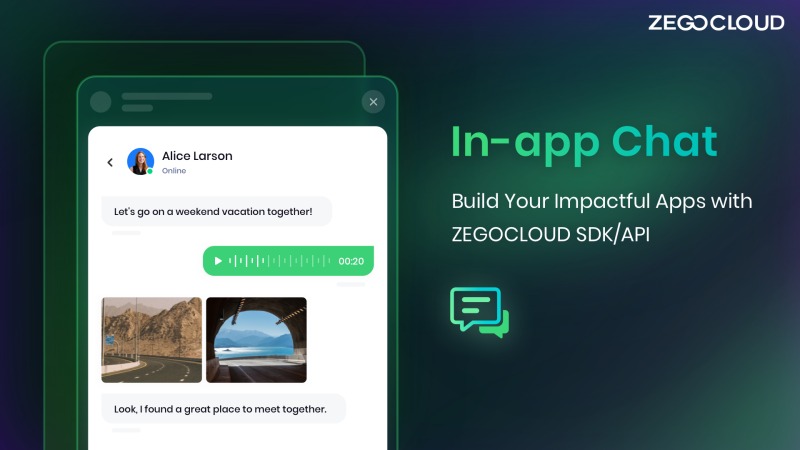
With ZEGOCLOUD’s SDK, you can create chat apps that are both engaging and efficient. Whether you’re a beginner or an experienced developer, building a chat app with JavaScript and ZEGOCLOUD’s In-app Chat SDK is a rewarding experience that can revolutionize communication in the digital world.
Preparation
- A ZEGOCLOUD developer account – Sign up
- Create a project and enable the In-app Chat SDK.
- A computer with internet connectivity
- Modern/updated web browsers
Once you have fulfilled the requirements mentioned above, you can proceed with the following steps to begin creating your JavaScript Chat App using ZEGOCLOUD’s In-app Chat SDK:
Create you app
- To set up a project folder for audio and video, create a folder with the following structure:
├── assets
│ ├── css
│ │ └── index.css # Page styling.
│ └── js
│ ├── biz.js # Your JavaScript code implementation here
│ └── zim.js # zim sdk
├── index.html # UI file of the application
- Copy and paste the code snippet below into the
index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no" />
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" />
<meta name="renderer" content="webkit" />
<title>ZIM</title>
<link rel="stylesheet" href="assets/css/index.css" />
</head>
<body></body>
<script src="./assets/js/zim.js"></script>
<script src="./assets/js/biz.js"></script>
</html>
Integrate the SDK
To create a dynamic JavaScript messaging app, the initial step involves integrating ZEGOCLOUD’s In-chat SDK into your project. Follow these unique steps to achieve this:
- To acquire the necessary dependencies, execute the provided command:
npm i zego-zim-web
- Within your project’s
zim.js
file, incorporate the subsequent import statement:
import { ZIM } from 'zego-zim-web';
Create a ZIM SDK instance
To begin a ZIM instance, the first step involves creating a separate instance for each client upon logging into the system. For instance, let’s consider two clients named A and B. In order for them to communicate with each other, both clients must use the create
method along with the AppID
obtained from the earlier mentioned Prerequisites section. This will generate individual ZIM SDK instances for each client, enabling seamless message exchange between them.
var appID = xxxx; // The appID is a sequence of digits, not a text string.
// The [create] method creates a ZIM instance only once. Subsequent calls to the [create] method will return null.
ZIM.create({ appID });
// Use [getInstance] to avoid hot updates and multiple [create] calls.
var zim = ZIM.getInstance();
Set event callbacks
Before a client user logs in, it is crucial to utilize the on method to personalize event callbacks. This enables receiving notifications for SDK errors and message-related callbacks, ensuring efficient management of different events.
// configure or set up a callback to receive error codes.
zim.on('error', function (zim, errorInfo) {
console.log('error', errorInfo.code, errorInfo.message);
});
// Register a callback to be notified of connection status changes.
zim.on('connectionStateChanged', function (zim, { state, event, extendedData }) {
console.log('connectionStateChanged', state, event, extendedData);
// If the SDK logs out due to a long period of network disconnection, you will need to log in again.
if (state == 0 && event == 3) {
zim.login(userInfo, token)
}
});
// Configure a callback to be notified of incoming one-to-one messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
// Configure a callback to be notified when the token expires.
zim.on('tokenWillExpire', function (zim, { second }) {
console.log('tokenWillExpire', second);
// To refresh the token, you have the option to utilize the renewToken method.
zim.renewToken(token)
.then(function({ token }){
// Renewed successfully.
})
.catch(function(err){
// Renew failed.
})
});
Log in to the ZIM SDK
To facilitate message exchange and token renewal after generating their ZIM SDK instances, both client A and client B should log in to the ZIM SDK. Follow these concise steps:
- Create a user object using the
ZIMUserInfo
method. - Utilize the
login
method, providing individual user information and the previously obtained Token from the Prerequisites section.
var userInfo = { userID: 'xxxx', userName: 'xxxx' };
var token = '';
zim.login(userInfo, token)
.then(function () {
// Login successful.
})
.catch(function (err) {
// Login failed.
});
Send messages
After a successful login by client A, they gain the ability to initiate message exchanges with client B.
The ZIM SDK offers a wide range of supported message types, including but not limited to:
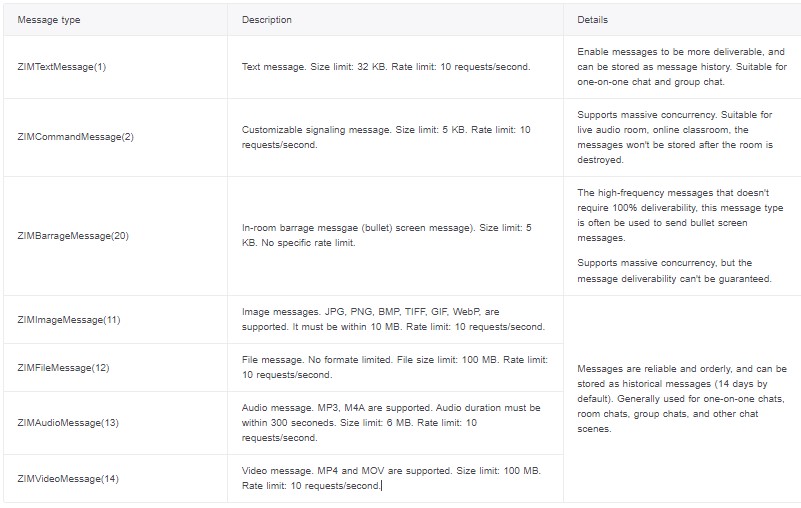
Client A can utilize the sendMessage
method to send one-to-one messages. By providing client B’s userID
, message content, and necessary information, client A can initiate the message exchange. The ZIMMessageSentResult
callback ensures that client A receives confirmation of the successful message delivery.
Furthermore, the onMessageAttached
callback offers access to a temporary ZIMMessage object for unsent messages. This functionality empowers client A to implement custom business logic, such as retrieving the message ID before sending it or creating interactive UI effects, especially when handling substantial content like videos.
// Send one-to-one text messages.
var toConversationID = ''; // Peer user's ID.
var conversationType = 0; // Message type; 1-on- chat: 0, in-room chat: 1, group chat:2
var config = {
priority: 1, // Set message priority [1,2,3]. Low (default), Medium, or High.
};
var messageTextObj = { type: 1, message: 'Message text', extendedData: 'Additional information for the message (optional)' };
var notification = {
onMessageAttached: function(message) {
// todo: Loading
}
}
zim.sendMessage(messageTextObj, toConversationID, conversationType, config, notification)
.then(function ({ message }) {
// Successful message
})
.catch(function (err) {
// Failed message.
});
Receive messages
Upon client B’s login, they will effortlessly receive client A’s messages via the receivePeerMessage
method’s designated callback. This pre-configured callback guarantees seamless reception and processing of incoming messages from client A.
// Configure a callback to receive one-to-one messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log out
To disconnect a client and conclude their session, simply invoke the logout
method, which effectively logs them out of the system.
zim.logout();
Destroy the ZIM SDK instance
To fully eliminate the ZIM SDK instance, utilize the destroy
method, which will thoroughly dismantle and erase all remnants of the SDK from the system.
zim.destroy();
Run a Demo
For comprehensive testing of ZEGOCLOUD’s In-app Chat SDK features, explore the unique sample demo provided.
Conclusion
Building a JavaScript Chat App opens up exciting possibilities for seamless communication. By leveraging the power of JavaScript and incorporating robust tools like ZEGOCLOUD’s In-app Chat SDK, you can create engaging chat experiences.
Follow the steps outlined in this guide to develop your own chat app and revolutionize the way people connect and interact online. Sign up to start building your JavaScript Chat App today and unlock the potential of real-time communication.
You may also like:
FAQ
Q1: What is the best way to build a JavaScript chat application?
The best way to build a JavaScript chat application is by using a combination of WebSockets for real-time communication, along with a frontend framework like React or Vue.js. You can also leverage chat SDKs, such as ZEGOCLOUD, to simplify the development process and ensure high-quality, scalable features.
Q2: What are the challenges in scaling a JavaScript chat application?
Scaling a JavaScript chat application involves challenges such as managing high user traffic, ensuring low latency, maintaining real-time synchronization across multiple clients, and optimizing server resources. Using cloud-based infrastructure and scalable backend services can help address these challenges, along with leveraging reliable chat SDKs.
Q3: What are the security considerations when building a JavaScript chat application?
Key security considerations include implementing end-to-end encryption, securing user authentication, protecting against cross-site scripting (XSS) attacks, and ensuring data privacy. It’s also important to regularly update and patch your software to protect against vulnerabilities.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!