Are you eager to create your very own Instagram-like app using React Native? Look no further! In this comprehensive guide, we will walk you through the step-by-step process of building an Instagram clone from scratch with ZEGOCLOUD. Get ready to harness the power of React Native and unleash your creativity in the world of app development
An Overview of Instagram
Instagram has become one of the most popular social media platforms, captivating millions of users worldwide. Launched in 2010, this visually-driven app offers a unique way for individuals and businesses to share their moments, stories, and products through photos and videos. With its user-friendly interface and engaging features, Instagram has revolutionized the way we connect and interact online.
At its core, Instagram enables users to upload and edit photos or videos, apply filters, and add captions or hashtags to enhance discoverability. The app’s Explore page suggests content tailored to users’ interests, fostering discovery and engagement. Additionally, Instagram Stories provides a temporary format for sharing ephemeral content, while IGTV offers longer-form videos for creators.
Instagram’s success can be attributed to its ability to adapt to the evolving digital landscape. It has introduced features like Reels, which allows users to create short videos set to music, and Shopping, enabling businesses to showcase and sell products directly on the platform.
With its massive user base and advertising options, Instagram has also become a valuable marketing tool for businesses. Influencers and brands leverage the platform’s visual appeal to reach and connect with their target audiences.
How Does Instagram Work?
Instagram has become a cultural phenomenon, but have you ever wondered how it actually works behind the scenes? Let’s dive into the inner workings of this popular social media platform.
At its core, Instagram is a mobile application that allows users to share photos and videos. The app operates on a simple principle: users create an account, upload visual content, and engage with others by liking, commenting, and following their posts.
When you upload a photo or video on Instagram, the platform processes and stores the media on its servers. It utilizes image recognition technology to suggest relevant hashtags and analyze the content for potential violations of its community guidelines. Once uploaded, your content becomes visible to your followers and can be discovered by others through hashtags or the Explore page.
The Explore page, powered by Instagram’s algorithm, curates content tailored to users’ interests based on their previous activity and engagement. This personalized approach enhances the user experience and encourages discovery.
Instagram’s social aspect revolves around interactions. Users can like, comment on, and share posts, fostering engagement and building connections. Additionally, the platform offers features like direct messaging and Stories, allowing users to communicate privately and share temporary content respectively.
From a business perspective, Instagram provides opportunities for brands and influencers to promote their products and services. The platform offers various advertising options, such as sponsored posts and stories, allowing businesses to reach their target audience effectively.
How to Make an App like Instagram with ZEGOCLOUD SDK
Building an app like Instagram requires careful planning and the right tools. One essential component is a reliable in-app chat system. ZEGOCLOUD In-app Chat SDK offers a robust solution, enabling real-time messaging between users within your app. With its easy integration and powerful features, ZEGOCLOUD can enhance user engagement and make your Instagram-like app truly interactive.
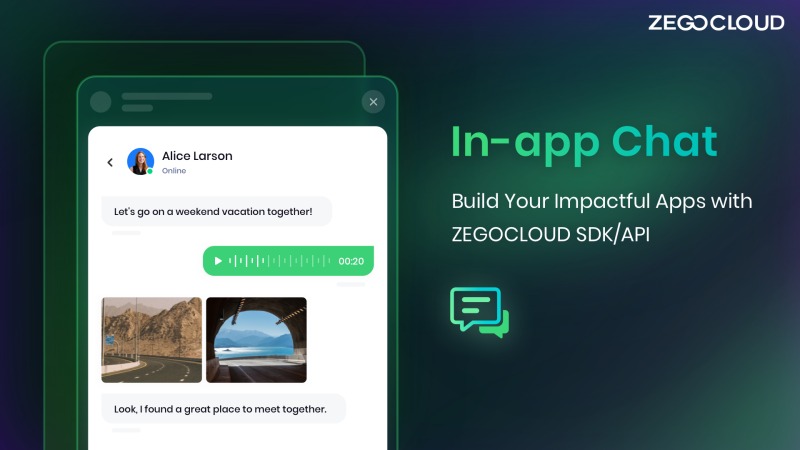
Preparation
- A ZEGOCLOUD developer account – Sign up
- Get app credentials and activate In-app chat functionality in the admin console
- Have NodeJS installed
- Basic knowledge of React Native
If you agree with the requirements above, you can follow the steps below to integrate chat functionality into your Instagram clone app.
Integrate the SDK
Optional: Create a new project
- To begin, initiate a fresh React Native project named “
Zego-zim-example
” using the convenient command line tool. You can effortlessly execute the utility without the need for installation by utilizing the Node.jsnpx
command.
npx react-native init zego-zim-example
- For iOS, compile and run the project by opening it in Xcode and selecting Product > Run. You also have the option to employ the subsequent command as an alternative:
yarn react-native run-ios
- To compile and run the project on Android, execute the following command in your terminal:
yarn react-native run-android
Import the SDK
To import the In-app Chat SDK using NPM, adhere to the following steps:
- To install the SDK, open a terminal window and navigate to your desired directory. Execute the following command:
npm install @zegocloud/zimkit-react-native
- Incorporate the SDK into your project seamlessly with the code below:
import { ZIMKiti18n } from '@zegocloud/zimkit-react-native';
Create a ZIM SDK instance
To commence a ZIM instance for client login, both clients A and B need to utilize the create method. By supplying the AppID
obtained from the initial prerequisites, they can establish their respective ZIM SDK instances, facilitating seamless message exchange between the two clients.
/*
The ZIM SDK uses the AppSign authentication mode by default. To change the authentication mode, contact Technical Support for SDK 2.3.0 or later, or make the change independently for SDK 2.3.3 or later.
To create a ZIM SDK object, use the static synchronized create method and provide the AppID and AppSign. The create method only creates a ZIM instance on its first invocation, returning null for subsequent calls.
*/
ZIM.create({ appID: 0, appSign: '' });
// To avoid multiple null returns from hot updates, obtain a single instance of the ZIM SDK using the getInstance() method.
var zim = ZIM.getInstance();
Set event callbacks
To personalize event callbacks, including receiving SDK error notifications and message-related notifications, invoke the on
method prior to the client user’s login process.
// Capture error codes by configuring a callback for the error event.
zim.on('error', function (zim, errorInfo) {
console.log('error', errorInfo.code, errorInfo.message);
});
// Stay informed about connection status changes by establishing a callback for the event.
zim.on('connectionStateChanged', function (zim, { state, event, extendedData }) {
console.log('connectionStateChanged', state, event, extendedData);
});
// Effortlessly receive one-to-one messages by configuring a callback for the message event.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log in to the ZIM SDK
For message exchange and token renewal, client A and client B can log in to the ZIM SDK by following these steps:
- Create a user object by leveraging the capabilities of the
ZIMUserInfo
method. - Log in using the
login
method, providing the user information andToken
obtained from the previous Prerequisites steps.
/* User IDs must be 32 characters or less and can contain letters, numbers, and the following special characters: ~!@#$%^&*()_+-=`;',.<>/.
Usernames must be 1 to 64 characters.
*/
var userInfo = { userID: 'xxxx', userName: 'xxxx' };
/*
During login, use the following authentication methods:
* Token-based: Provide the token from the [Guides - Authentication] document.
* AppSign (default in SDK 2.3.0): Leave the Token field empty.
*/
zim.login(userInfo, '')
.then(function () {
// Login successful.
})
.catch(function (err) {
// Login failed.
});
Send messages
To send a message, client A can utilize the sendMessage
method by specifying B’s user ID, content, and type. Upon utilizing this method, you will receive ZIMMessageSentResult
, which will activate onMessageAttached
with a ZIMMessage
. Prior to sending, leverage onMessageAttached
to obtain the ID or local ID of the message.
// To send a one-on-one (1V1) message, simply configure the ZIMConversationType as Peer.
var toUserID = 'xxxx1';
var config = {
priority: 1 // Choose the desired message priority level from three options: low, medium, or high. Low is the default priority.
};
var type = 0; // The session type can be categorized as one-on-one (0), chat room (1), or group chat (2) for different communication scenarios.
var notification = {
onMessageAttached: function(message) {
// todo: Loading
}
};
// Send a 1V1 text message.
var messageTextObj = { type: 1, message: 'Text message content' };
zim.sendMessage(messageTextObj, toUserID, type, config, notification)
.then(function ({ message }) {
// Successful messages.
})
.catch(function (err) {
// Failed messages.
});
Receive messages
Once client B logs in, any messages sent by client A will be delivered to the pre-configured callback function set in the receivePeerMessage
method.
// To receive one-to-one messages, establish a callback registration.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log out
To initiate the client’s logout
, utilize the logout
method.
zim.logout();
Destroy the ZIM SDK instance
To remove the ZIM SDK instance, simply invoke the destroy
method to dismantle it.
zim.destroy();
To add voice and video calling to your Instagram clone app, use ZEGOCLOUD’s Voice call SDK and live Video Call SDK.
Run a Demo
Experience the full potential of ZEGOCLOUD’s In-app Chat SDK by experimenting with our React Native sample app.
Conclusion
Building an Instagram clone with React Native is a great way to learn the basics of React Native and create a fully functional mobile app. By following the steps outlined in this guide, you can create a clone of Instagram that looks and feels like the real thing. With a little creativity and effort, you can even add your own unique features to make your app stand out from the crowd.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!