Are you interested in creating your own social audio platform? If so, this article on developing a Clubhouse clone app is for you.
We will provide you with a step-by-step guide with ZEGOCLOUD, covering everything from design to features, so you can be sure you have all the information you need to get started.
What is Clubhouse Clone
As a developer, venturing into creating a Clubhouse clone presents vast opportunities and gratifying outcomes. This clone app replicates the beloved social audio platform, enabling users to engage in dynamic real-time audio conversations. By crafting your own Clubhouse clone, you can harness the burgeoning demand for audio-centric social networking, captivating a global user base and fostering vibrant connections and communities.
Key Features of the Clubhouse App
Clubhouse, the trending audio-based social networking app, offers many captivating features that set it apart. Here are the key features of the Clubhouse:
- Clubhouse allows you to engage in real-time voice discussions, facilitating interactive and dynamic conversations with users worldwide.
- Create or join virtual rooms where users can host or participate in various topics, fostering meaningful and niche discussions.
- Stay connected with thought leaders and industry experts by following their profiles and joining their engaging conversations.
- Ensure a safe and respectful environment with robust moderation features that empower hosts to manage discussions effectively.
- With Clubhouse, you can connect with like-minded individuals, industry professionals, and potential collaborators, expanding your network and building valuable relationships.
- Receive personalized alerts about upcoming rooms, topics of interest, and engagements, ensuring you never miss an opportunity to participate.
- Explore a diverse range of rooms, covering various interests, hobbies, and industries, allowing you to discover new insights and perspectives.
- Maintain control over your profile and room accessibility, ensuring your desired level of privacy while engaging in discussions.
- Establish your own exclusive clubs or join existing ones to cultivate communities around specific interests, creating a space for in-depth conversations.
- You can also connect with fellow users privately through one-on-one messaging, enabling deeper connections beyond the audio rooms.
How to Build a Clubhouse Clone App using React Native
If you’re looking to develop a Clubhouse clone app using React Native, ZEGOCLOUD Voice Call SDK is an invaluable tool to consider. This SDK provides the necessary components for integrating real-time audio conversations into your app, allowing users to engage in interactive discussions like on Clubhouse.
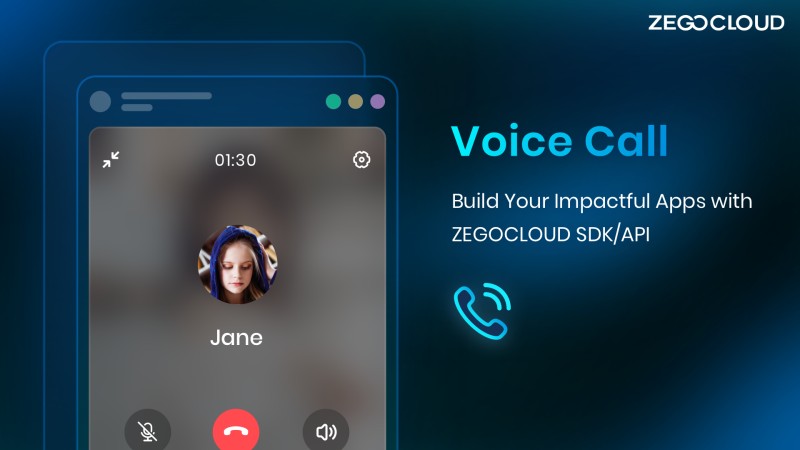
With its easy-to-use features, such as high-quality audio streaming, room creation, and moderation tools, ZEGOCLOUD’s Voice Call SDK simplifies the development process. Whether you’re an experienced React Native developer or a beginner, this SDK can help you create a seamless Clubhouse clone app with captivating audio experiences. Moreover, it works well with Flutter and Android if you have Flutter Clubhouse clone app need or Android Clubhouse clone app.
Preparation
- A ZEGOCLOUD developer account – Sign up
- Create a project to get app credentials
- A computer with internet and multimedia capabilities
- React Native 0.60.0 or later
- Android/iOS device or emulator
- Have NodeJS installed
If you have met the requirements above, you can now proceed with the following steps to kickstart the development of your audio-based app like Clubhouse:
Create a new project
Configure your development environment and initiate your project effortlessly by executing this command:
react-native init [YourProject]
With this command, you’ll be all set to commence your project, ensuring a smooth and efficient start to building your audio-based app.
Import the SDK
Import ZEGOCLOUD’s Voice Call SDK into your workspace seamlessly by following these concise and unique steps below:
- Navigate to your project’s root directory, open the
package.json
file, and include the following line in the dependencies section:"zego-express-engine-reactnative": "^x.y.z"
. Remember to replace “x.y.z
” with the most up-to-date version of the SDK, which can be acquired by executing an npm command. - Install the SDK effortlessly by executing either of the following concise commands in the root directory of your project:
npm install zego-express-engine-reactnative --save
- To install the necessary dependencies in iOS, navigate to the iOS root directory and execute the concise command:
pod install
Add permissions
Permissions can be set as needed for Android and iOS apps. Here are the steps on how to do it:
Android
In the app/src/main/AndroidManifest.xml
file, include the following unique code snippet:
<!-- Permissions required by the SDK -->
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<!-- Permissions required by the App -->
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-feature
android:glEsVersion="0x00020000"
android:required="true" />
<uses-feature android:name="android.hardware.camera" />
<uses-feature android:name="android.hardware.camera.autofocus" />
Android 6.0 requires dynamic permissions for some important permissions. This means you must request permission from the user at runtime instead of declaring it in your manifest file.
To request dynamic permission, you can use the following code:
import {PermissionsAndroid} from 'react-native';
const granted = PermissionsAndroid.check(PermissionsAndroid.PERMISSIONS.CAMERA,
PermissionsAndroid.RECORD_AUDIO);
granted.then((data)=>{
if(!data) {
const permissions = [PermissionsAndroid.PERMISSIONS.RECORD_AUDIO, PermissionsAndroid.PERMISSIONS.CAMERA];
PermissionsAndroid.requestMultiple(permissions);
}
}).catch((err)=>{
console.log(err.toString());
})
iOS
- In Xcode, to access the Custom iOS Target Properties, select the target project and then go to the
Info
tab.
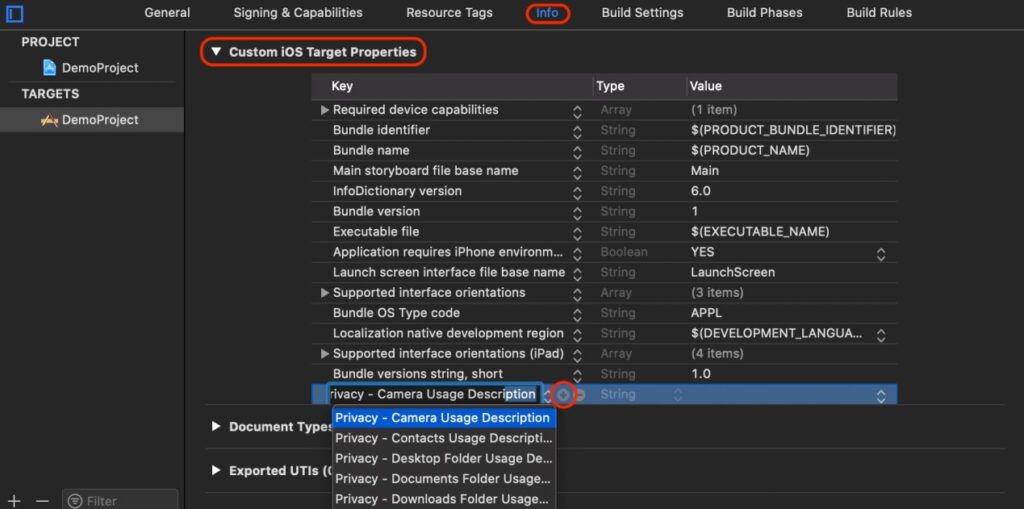
- To add microphone and camera permissions in Xcode, click the “
+
” button and select “Privacy
“. Then, select “Camera Usage Description
” and “Microphone Usage Description
“.
Privacy - Camera Usage Description
Privacy - Microphone Usage Description
The permissions will be added to the list and will be shown below:

Create a ZegoExpressEngine instance
Optional: Create the UI
Implement basic real-time audio, adding an audio window and an End button before creating a ZegoExpressEngine
instance.
To create ZegoExpressEngine
instance, call the createEngineWithProfile()
method with your project’s AppID
. To receive callbacks, call the on()
method after creating the instance.
// Import the ZegoExpressEngine
import ZegoExpressEngine from 'zego-express-engine-reactnative';
const profile = {
appID : xxx,
scenario : 0
};
ZegoExpressEngine.createEngineWithProfile(profile)
Log in to a room
To have access to a room, call the loginRoom()
method. A new room will be created if the room ID does not exist, and you will be logged in automatically.
// set the token
let roomConfig = {};
roomConfig.token = "xxxx";
// log in to a room
ZegoExpressEngine.instance().loginRoom('room1', {'userID': 'id1', 'userName': 'user1'}, roomConfig);
One can utilize event callback methods within the event handler to monitor and manage events occurring after successful room login. Several essential event callbacks pertain to room users and streams, offering valuable insights and control. These callbacks serve as crucial tools for developers to handle and respond to various interactions within the room, ensuring a smooth and dynamic user experience.
roomStateUpdate
: when the current user’s room connection status changes, the SDK sends an event notification through this callback.roomUserUpdate
: when other users join or leave the room, the SDK sends an event notification through this callback.roomStreamUpdate
: when new streams are published to the room or existing ones in the room stop, the SDK sends an event notification through this callback.
// This callback is called when the current user's room connection status changes, such as when the user is disconnected from the room or login authentication fails.
ZegoExpressEngine.instance().on('roomStateUpdate', (roomID, state, errorCode, extendedData) => {
});
// This callback is called when other users join or leave the room.
ZegoExpressEngine.instance().on('roomUserUpdate', (roomID, updateType, userList) => {
});
// This callback is called when new streams are published to the room or existing streams in the room stop.
ZegoExpressEngine.instance().on('roomStreamUpdate', (roomID, updateType, streamList) => {
});
Publish streams
Start publishing a stream
Publish a local audio or video stream to remote users by calling the startPublishingStream() method and passing the stream ID to the streamID parameter.
ZegoExpressEngine.instance().startPublishingStream("streamID");
Start the local video preview
Initiate the local video preview, using the startPreview()
method and designating the canvas parameter to render the local video onto a specific view. By calling this method and configuring the appropriate canvas, the application enables users to visualize their own video feed, enhancing their overall engagement and interaction within the platform.
import { findNodeHandle } from 'react-native';
// To enable local video preview, obtain a Ref attribute from React that references a view of the local preview.
let localViewRef = findNodeHandle(this.refs.zego_preview_view);
// Enable the local preview
ZegoExpressEngine.instance().startPreview({
'reactTag': localViewRef,
'viewMode': 0,
'backgroundColor': 0
});
// React render
render() {
return (
<View>
<ZegoTextureView ref='zego_preview_view'/>
</view>
)
}
Configure publish stream callback
Implement the event callback to listen for and handle events that may happen after stream publishing starts. This callback is fired when the status of stream publishing changes, such as when the stream is interrupted due to network issues and the SDK retries to start publishing the stream again.
ZegoExpressEngine.instance().on("PublisherStateUpdate", (streamID, state, errorCode, extendedData) => {
// The SDK sends an event notification for stream status changes, such as interruptions and retries.
});
Play streams
Start playing a stream
To start playing a remote audio or video stream, call the startPlayingStream()
method and pass the stream ID to the streamID
parameter and the view for rendering the video to the view parameter.
import { findNodeHandle } from 'react-native';
// To enable local video preview, get a Ref attribute from React that references a view of the local preview.
let remoteViewRef = findNodeHandle(this.refs.zego_play_view);
// Start playing a stream
ZegoExpressEngine.instance().startPlayingStream("streamID", {
'reactTag': remoteViewRef,
'viewMode': 0,
'backgroundColor': 0
});
// React render
render() {
return (
<View>
<ZegoTextureView ref='zego_play_view'/>
</view>
)
}
Configure stream play callbacks
Implement the event callback to listen for and handle events that may happen after stream playing starts. This callback is fired when the status of the stream playing changes, such as when the stream is interrupted due to network issues and the SDK retries to start playing the stream again.
ZegoExpressEngine.instance().on("PlayerStateUpdate", (streamID, state, errorCode, extendedData) => {
/**
The SDK sends an event notification through this callback if the status of a stream changes after it starts playing. This can happen if the stream is interrupted due to network issues and the SDK retries to start playing it again.
**/
});
Halt publishing and playing streams
- Stop publishing a stream
The stopPublishingStream()
function stops the transmission of a local audio or video stream. This allows users to control their audio and video output as needed.
ZegoExpressEngine.instance().stopPublishingStream();
2. Stop the local video preview
In order to stop a local video preview, call the stopPreview()
method.
ZegoExpressEngine.instance().stopPreview();
3. Stop playing a stream
To cease playback of a remote audio or video stream, developers can employ the stopPlayingStream()
function, providing the corresponding stream ID
as a parameter.
ZegoExpressEngine.instance().stopPlayingStream("streamID");
Log out of a room
Log out of a room, call logoutRoom()
with the room ID
. This will stop all stream publishing, stream playing, and local video preview, and send a notification of the change of room connection status through the roomStateUpdate
callback.
ZegoExpressEngine.instance().logoutRoom('room1');
Destroy the ZegoExpressEngine instance
Release resources and terminate the ZegoExpressEngine
instance, simply calling destroyEngine()
. This method effectively dismantles the instance and ensures the efficient allocation of system resources.
ZegoExpressEngine.destroyEngine();
Run a Demo
You can check out the sample demo to get a practical overview of ZEGOCLOUD’s Voice Call SDK.
Conclusion
Building React native Clubhouse clone app requires careful planning and the right tools. ZEGOCLOUD’s Voice Call SDK can help developers create a seamless and high-quality audio communication experience for their users. With its user-friendly interface and customizable features, ZEGOCLOUD’s Voice Call SDK can help developers create a captivating and engaging platform that emulates the success of Clubhouse. Sign up to get started!
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!