Quick start
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Integrate the SDK
Import the SDK
- Add @zegocloud/zego-uikit-prebuilt-live-audio-room-rn as dependencies.
yarn
npm
yarn add @zegocloud/zego-uikit-prebuilt-live-audio-room-rn
1
npm install @zegocloud/zego-uikit-prebuilt-live-audio-room-rn
1
- Add other dependencies.
Run the following command to install other dependencies for making sure the @zegocloud/zego-uikit-prebuilt-live-audio-room-rn
can work properly:
yarn
npm
yarn add react-delegate-component zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.16.0 @zegocloud/zego-uikit-rn
1
npm install react-delegate-component zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.16.0 @zegocloud/zego-uikit-rn
1
Using the Live Audio Room Kit
- Specify the
userID
anduserName
for connecting the Live Audio Room Kit service. - Create a
roomID
that represents the live audio room you want to create.
Note
userID
,userName
, androomID
can only contain numbers, letters, and underlines (_).- Using the same
roomID
will enter the same live audio room.
Warning
With the same roomID
, only one user can enter the live audio room as host. Other users need to enter the live audio room as the audience.
App.js
import React, { Component } from 'react';
import { StyleSheet, View } from 'react-native';
import ZegoUIKitPrebuiltLiveAudioRoom, { HOST_DEFAULT_CONFIG } from '@zegocloud/zego-uikit-prebuilt-live-audio-room-rn'
export default function LiveAudioRoomPage(props) {
return (
<View style={styles.container}>
<ZegoUIKitPrebuiltLiveAudioRoom
appID={yourAppID}
appSign={yourAppSign}
userID={userID} // userID can be something like a phone number or the user id on your own user system.
userName={userName}
roomID={roomID} // roomID can be any unique string.
config={{
...HOST_DEFAULT_CONFIG,
onLeave: () => { props.navigation.navigate('HomePage') },
}}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
zIndex: 0,
},
});
1
Configure your project
- Android:
- Open the
my_project/android/app/src/main/AndroidManifest.xml
file and add the following:
Untitled
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.VIBRATE"/>
1
- Open the
my_project/android/app/proguard-rules.pro
file and add the following:
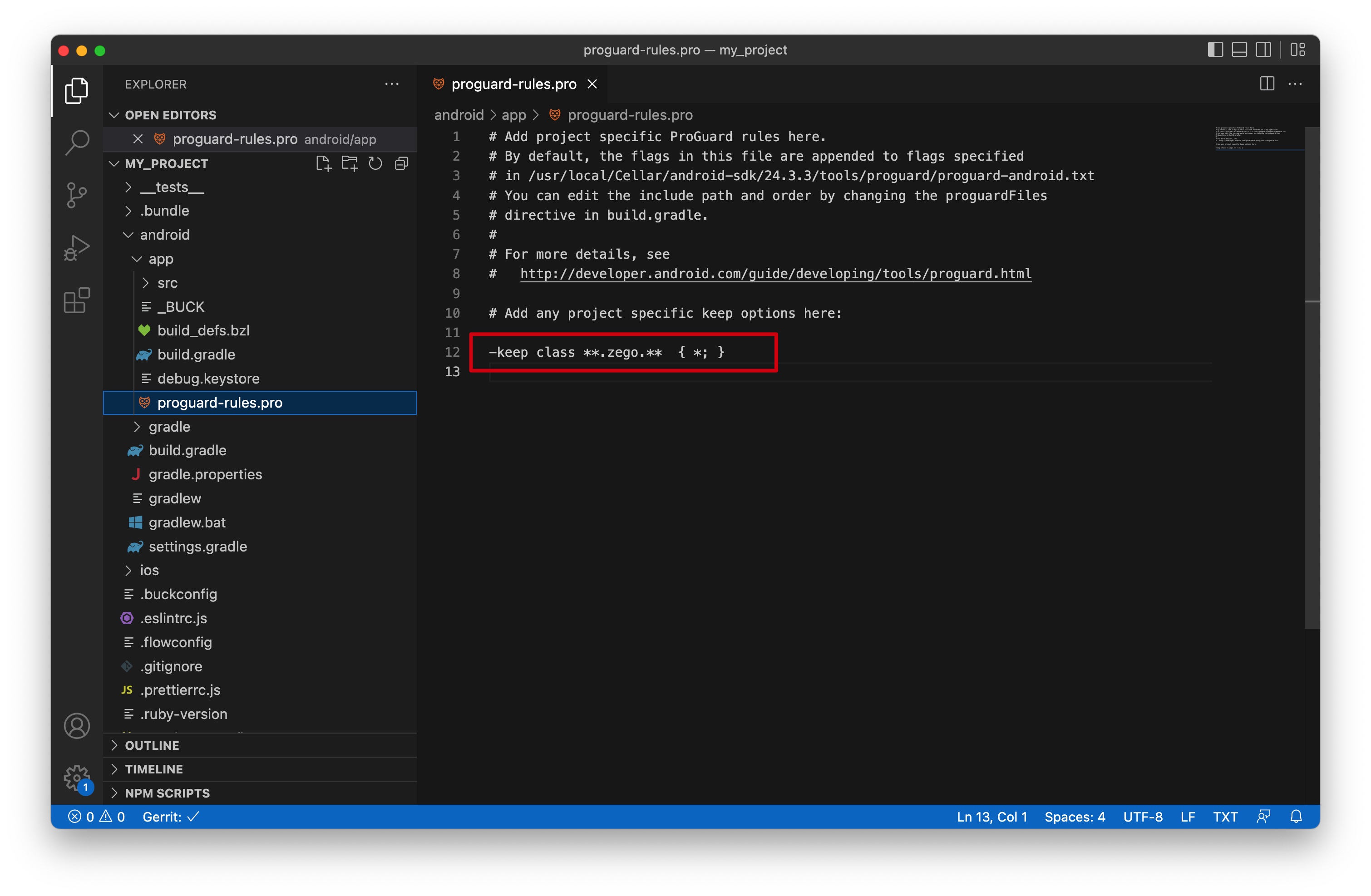
Untitled
-keep class **.zego.** { *; }
1
- iOS:
- Open the
my_project/ios/my_project/Info.plist
file and add the following:
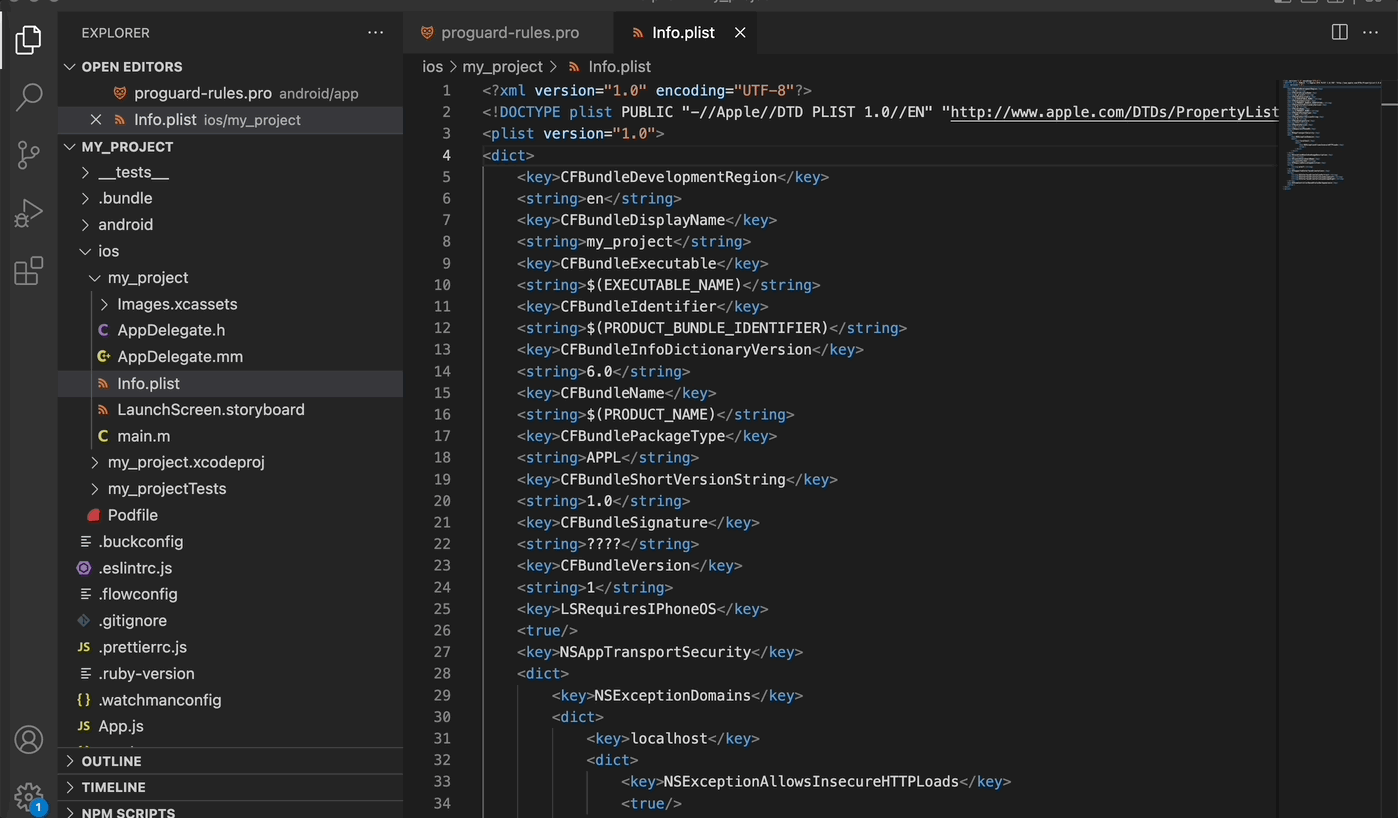
Untitled
<key>NSCameraUsageDescription</key>
<string>We need to use the camera</string>
<key>NSMicrophoneUsageDescription</key>
<string>We need to use the microphone</string>
1
- Disable the Bitcode.
a. Open the your_project > ios > [your_project_name].xcworkspace
file.
b. Select your target project, and follow the notes on the following two images to disable the Bitcode respectively.
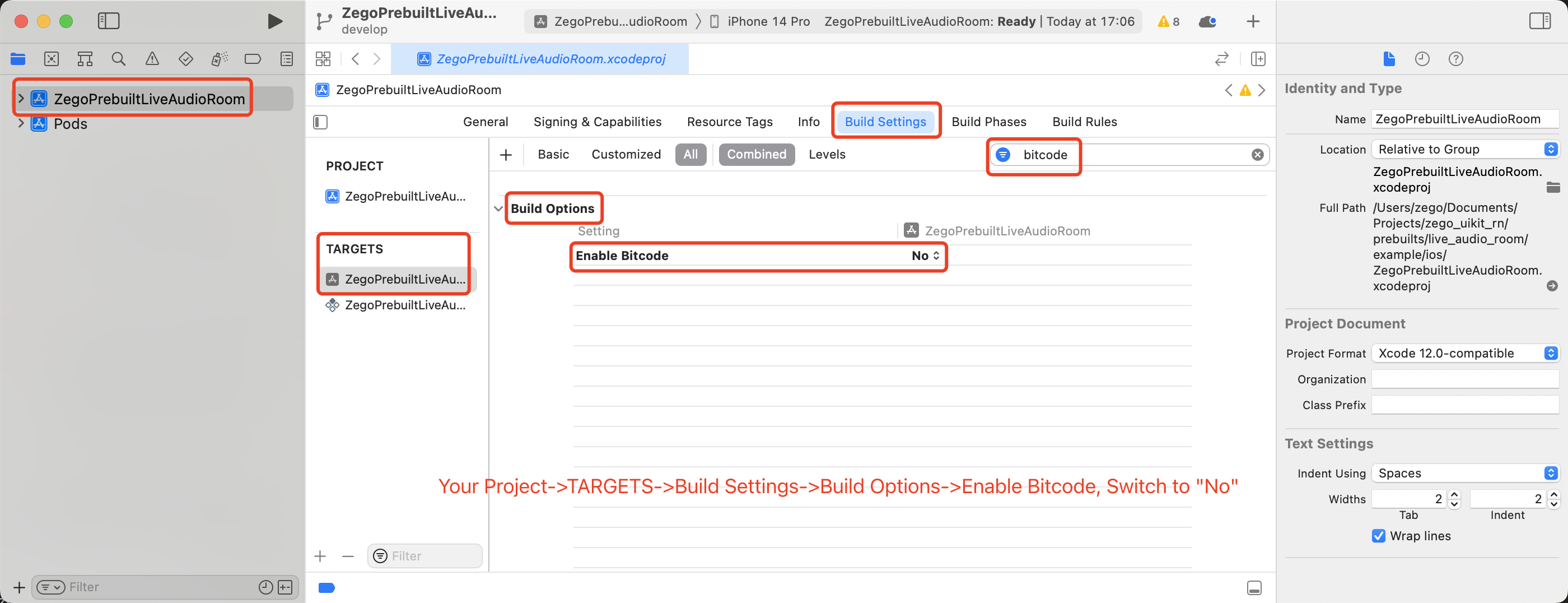
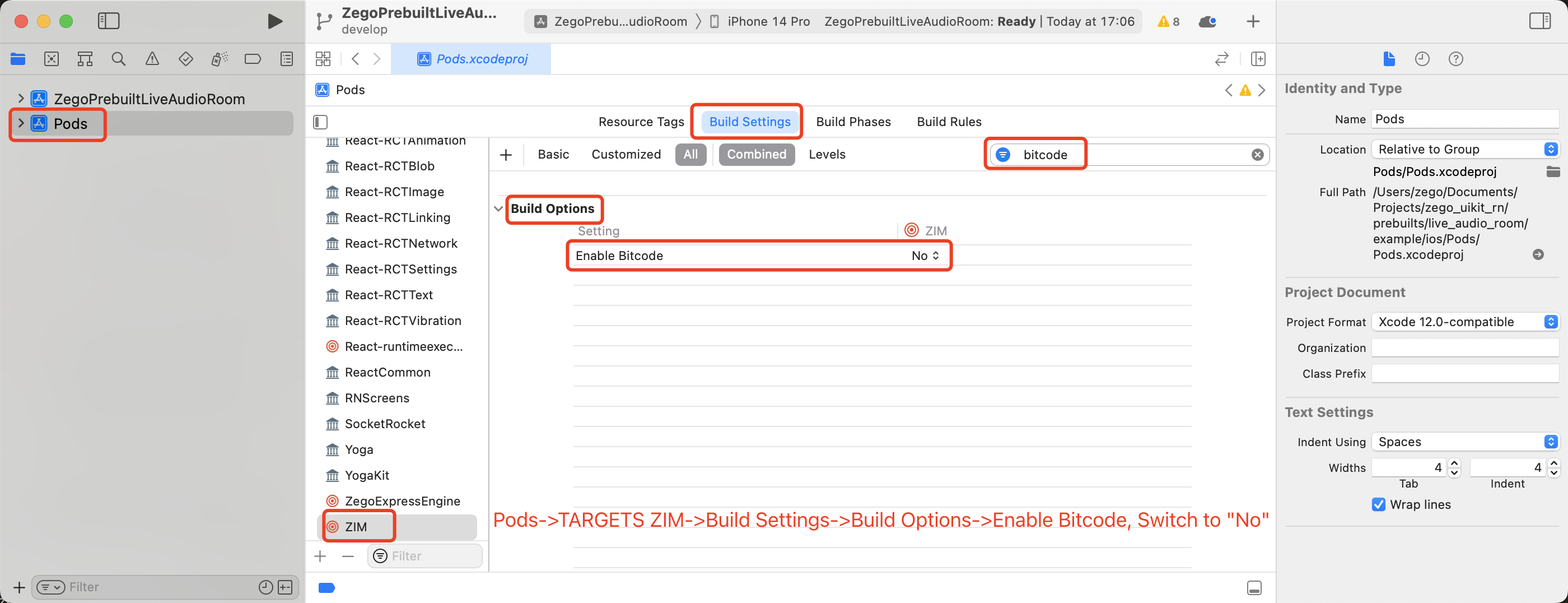
Run & Test
Note
If your device is not performing well or you found a UI stuttering, run in Release mode for a smoother experience.
- Run on an iOS device:
yarn
npm
yarn ios
1
npm run ios
1
- Run on an Android device:
yarn
npm
yarn android
1
npm run android
1
Related guide
Resources
Sample code
Click here to get the complete sample code.