Quick start
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Prepare the environment
Before you attempt to integrate the SDK, make sure that the development environment meets the following requirements:
- Xcode 15.0 or higher version.
- iOS 12.0 or higher version and iOS devices that support audio and video.
- The iOS device is connected to the Internet.
Integrate the SDK
Add dependencies
Do the following to add the ZegoUIKitPrebuiltLiveAudioRoom
dependencies:
-
Open Terminal, navigate to your project's root directory, and run the following to create a
podfile
:Untitledpod init
1 -
Edit the
Podfile
file to add the dependencies:Untitledpod 'ZegoUIKitPrebuiltLiveAudioRoom'
1 -
In Terminal, run the following to install the required dependencies with Cocoapods:
Untitledpod install
1
Import SDKs
Run the following to import the SDKs: ZegoUIKitPrebuiltLiveAudioRoom
and ZegoUIKit
.
import ZegoUIKitPrebuiltLiveAudioRoom
import ZegoUIKit
// YourViewController.swift
class ViewController: UIViewController {
//Other code...
}
Using the Live Audio Room Kit
- Specify the
userID
anduserName
for connecting the Live Audio Room Kit service. - Create a
roomID
that represents the live audio room you want to create.
userID
,userName
, androomID
can only contain numbers, letters, and underlines (_).- Using the same
roomID
will enter the same live audio room.
With the same roomID
, only one user can enter the live audio room as host. Other users need to enter the live audio room as the audience.
class ViewController: UIViewController {
let appID: UInt32 = <#AppID#>
let appSign: String = <#AppSign#>
var userID: String = <#UserID#>
var userName: String = <#UserName#>
var roomID: String = <#RoomID#>
@IBAction func startLiveAudio(_ sender: Any) {
let config: ZegoUIKitPrebuiltLiveAudioRoomConfig = ZegoUIKitPrebuiltLiveAudioRoomConfig.host()
let liveAudioVC: ZegoUIKitPrebuiltLiveAudioRoomVC = ZegoUIKitPrebuiltLiveAudioRoomVC(appID, appSign: appSign, userID: userID, userName: userName, roomID: roomID, config: config)
liveAudioVC.modalPresentationStyle = .fullScreen
self.present(liveAudioVC, animated: true, completion: nil)
}
@IBAction func joinLiveAudio(_ sender: Any) {
let config: ZegoUIKitPrebuiltLiveAudioRoomConfig = ZegoUIKitPrebuiltLiveAudioRoomConfig.audience()
let liveAudioVC: ZegoUIKitPrebuiltLiveAudioRoomVC = ZegoUIKitPrebuiltLiveAudioRoomVC(appID, appSign: appSign, userID: userID, userName: userName, roomID: roomID, config: config)
liveAudioVC.modalPresentationStyle = .fullScreen
self.present(liveAudioVC, animated: true)
}
}
Then, you can create a live audio room by presenting the VC
.
Configure your project
- Open the
Info.plist
, and add the following code inside thedict
part:
<key>NSCameraUsageDescription</key>
<string>Access permission to camera is required.</string>
<key>NSMicrophoneUsageDescription</key>
<string>Access permission to microphone is required.</string>
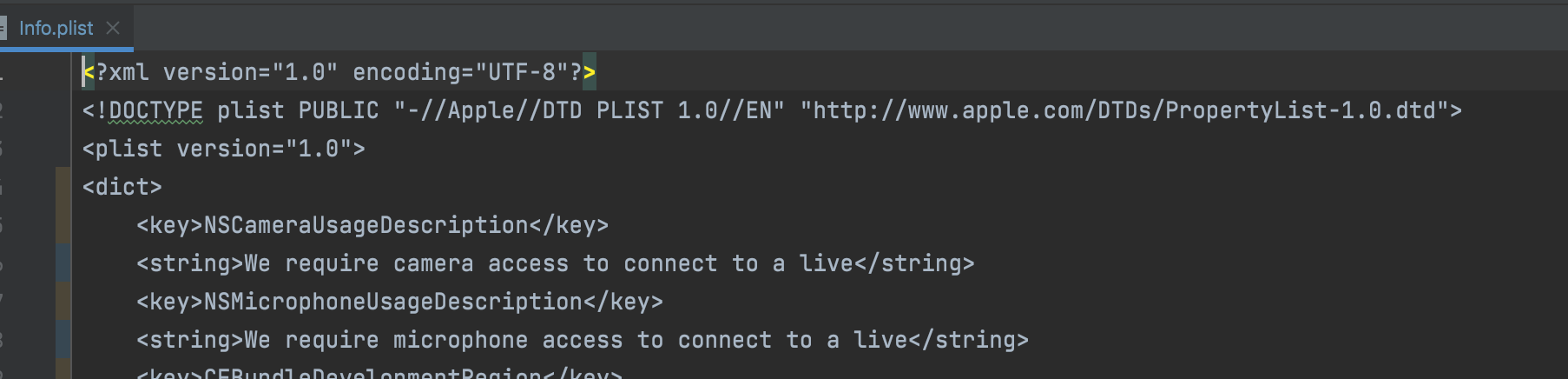
- Disable the Bitcode. Select your target project, and follow the notes on the following two images to disable the Bitcode respectively.
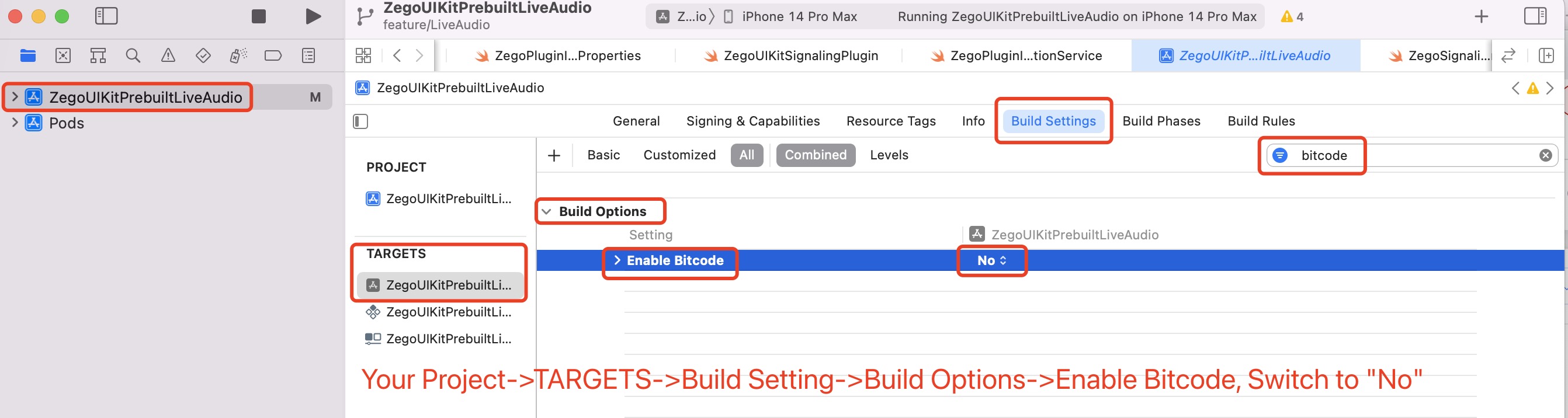
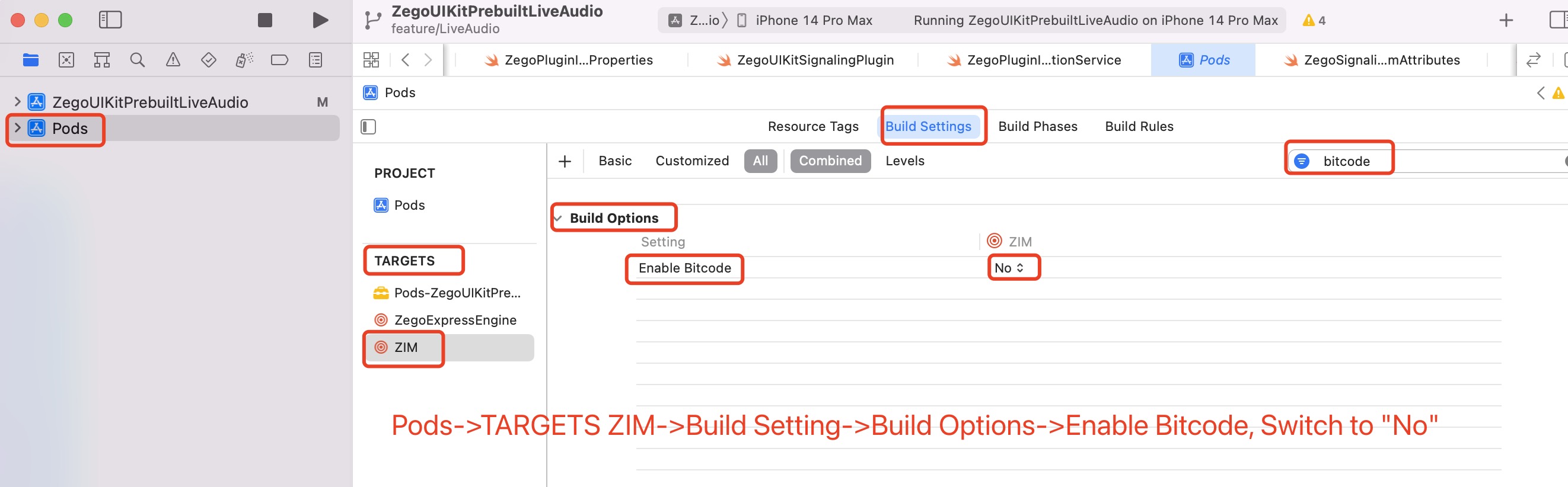
Run & Test
Now you have finished all the steps!
You can simply click the Run in XCode to run and test your App on your device.
Related guide
Resources
Click here to get the complete sample code.