Token Authentication
Overview
When a user initializes ZEGO MiniGameEngine SDK, the ZEGOCLOUD server checks whether the user has the permissions to use the mini-game service based on the token in the user request. This process is called token-based authentication. Token-based authentication prevents risks caused by insufficient permissions or improper operations.
How it works
Before you initialize ZEGO MiniGameEngine SDK, your server needs to generate a token. The ZEGOCLOUD server checks whether the user is a legitimate user based on the token in the user request.
The following figure shows how to perform token-based authentication during initialization.
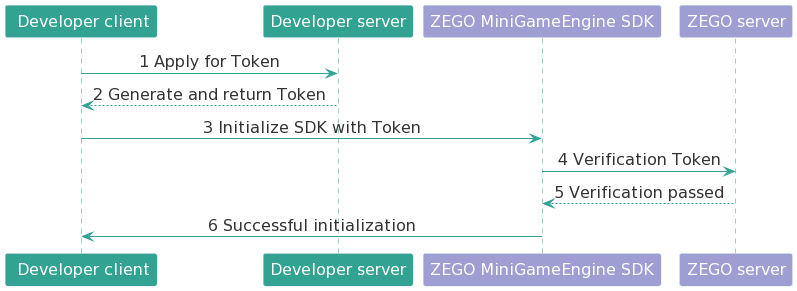
- A client applies for a token.
- The developer server generates a token and returns the token to the client.
- The client initializes ZEGO MiniGameEngine SDK with the token.
- ZEGO MiniGameEngine SDK automatically sends the token to the ZEGOCLOUD server for authentication.
- The ZEGOCLOUD server returns the authentication result to ZEGO MiniGameEngine SDK.
- ZEGO MiniGameEngine SDK returns the authentication result to the client. If the client is not authorized, the initialization fails.
Prerequisites
Before you perform token-based authentication, make sure that ZEGO MiniGameEngine SDK is integrated with the project. For more information, see Integrate the SDK.
Procedure
The following section describes how to generate a token on your server, configure the token by using ZEGO MiniGameEngine SDK, and handle an expired token.
Generate a token
To ensure security, we recommend that you generate a token on your own server.
Obtain the values of the AppID
and ServerSecret
parameters
Log in to the ZEGOCLOUD console and create a project. Obtain the values of the AppID
and ServerSecret
parameters that are required to connect to the mini-game service. Then, contact ZEGOCLOUD business staff to activate the mini-game service.
Generate a token on your server
The client applies for a token from your server. Your server generates a token and sends the token to the client.
For ease of use, ZEGOCLOUD provides the open source zego_server_assistant plug-in on GitHub and Gitee. The plug-in supports multiple programming languages, such as Go, C++, Java, Python, PHP, .NET, and Node.js. You can deploy the plug-in on your server to generate a token.
Language | Supported version | Core function | Code base | Sample code | |
---|---|---|---|---|---|
User identity Token | User privilege Token | ||||
Go | Go 1.14.15 or later | GenerateToken04 | |||
C++ | C++ 11 or later | GenerateToken04 | |||
Java | Java 1.8 or later | generateToken04 | |||
Python | Python 3.6.8 or later | generate_token04 | |||
PHP | PHP 7.0 or later | generateToken04 | |||
.NET | .NET Framework 3.5 or later | GenerateToken04 | |||
Node.js | Node.js 8 or later | generateToken04 |
Take Go language as an example, you can do the following steps to generate a Token:
- go get github.com/ZEGOCLOUD/zego_server_assistant
- import "github.com/ZEGOCLOUD/zego_server_assistant/token/go/src/token04"
- Call the
GenerateToken04
method to generate a Token.
The following code shows how to generate a user identity Token:
package main
import (
"fmt"
"github.com/ZEGOCLOUD/zego_server_assistant/token/go/src/token04"
)
/*
Sample code for generating a user identity Token:
*/
func main() {
var appId uint32 = <Your AppId> // The application ID that you obtain after you create a project in the ZEGOCLOUD console. The value is of the UINT32 type.
userId := <Your userID> // The user ID that you specify. The value is of the STRING type.
secret := <ServerSecret> // The secret key that you obtain after you create a project in the ZEGOCLOUD console. The value is a string of 32 bytes.
var effectiveTimeInSeconds int64 = <Your token effectiveTime> // The validity period of the token. The value is of the INT64 type. Unit: seconds.
var payload string = ""
token, err := token04.GenerateToken04(appId, userId, serverSecret, effectiveTimeInSeconds, payload)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(token)
}
Configure the token
Pass in the token when you call the init operation to initialize ZEGO MiniGameEngine SDK.
// Initialize ZEGO Express SDK. Otherwise, you cannot receive the callback of the `init` operation.
// Initialize ZEGO MiniGameEngine SDK.
ZegoGameUserInfo *userInfo = [[ZegoGameUserInfo alloc] init];
// After you create a project in the [ZEGOCLOUD console] (https://console.zego.im/), you can obtain the application ID from the project information. Append an "L" to the application ID. Example: 1234567890L.
long appID = 12345678L;
NSString *token = @"your token"; // The token that your server generates.
userInfo.userID = @"your user ID"; // The custom user ID.
userInfo.userName = @"your user name"; // The custom username.
userInfo.avatar = @"your user avatar"; // The URL of the user avatar.
[ZegoMiniGameEngine.sharedEngine init:appID token:token userInfo:userInfo callBack:^(NSInteger errorCode2) {
}];
Update the token
The SDK sends a notification by using the onTokenWillExpire callback 30 seconds before the token expires.
After you receive the callback, you need to obtain a new token from your server and update the token by calling the updateToken operation provided by the SDK.
// Obtain a notification indicating that the token expires after 30 seconds.
- (void)onTokenWillExpire {
// Updates the token.
[ZegoMiniGameEngine.sharedEngine updateToken:@"The new token"];
}